Hello,
I am building an interactive UI and I want to add validation to a textbox which should match specific patterns. If the user input doesn't match the pattern then UI should declare it as invalid state and show some tooltip to guide the user.
Need some guidance on how i can add it in interactive UI.
Thanks and Regards,
Aditi.
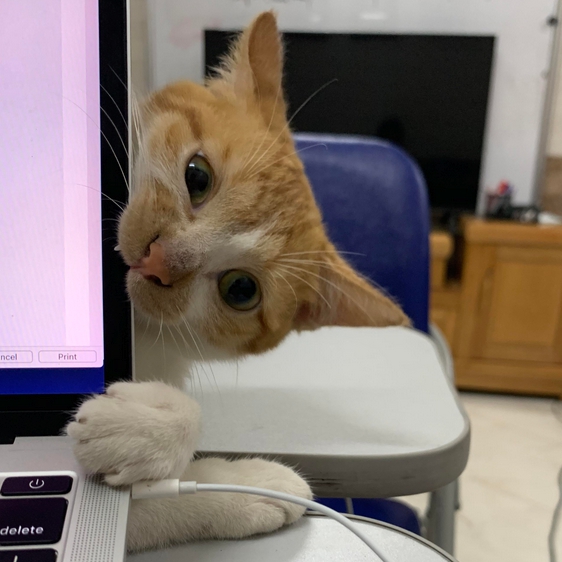
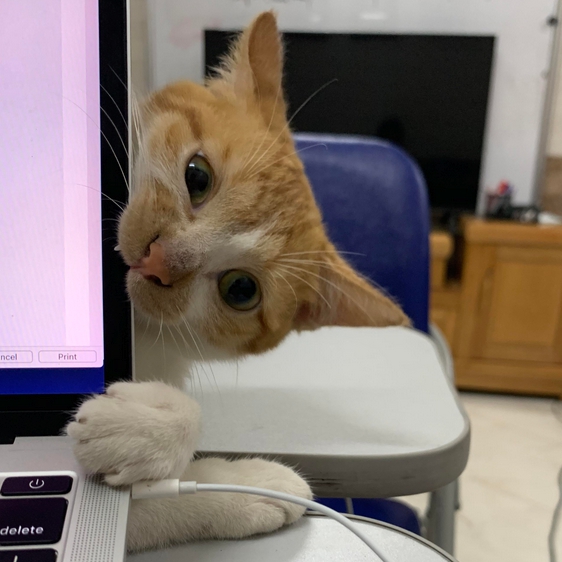
As this question has now been inactive for a long time, I will close it. If you still want more information about this, could you post a new question?
Hi Aditi,
One way of achieving this result would be to add an extra 'Label' to the dialog where you present to the user if the input is valid or invalid. This extra label will then serve as a status for your input validation.
Example:
// Initialize dialog with a textbox and label serving as status validation
Label labelInfoUserInput = new Label("User Input:");
TextBox textBoxInput = new TextBox();
Label labelStatusInput= new Label(String.Empty);AddWidget(labelInfoUserInput , row, 0);
AddWidget(textBoxInput, row, 1);
AddWidget(labelStatusInput, row, 2);
When the user updates the value within your TextBox, validate the new data by listening to the Changed event and update the status label.
Example:
textBoxInput.Changed += TextInputChanged;
private void TextInputChanged(object sender, TextBox.TextBoxChangedEventArgs e)
{
Regex regex = new Regex("*"); // update pattern to match
if (!regex.IsMatch(e.Value))
{
labelStatusInput.Text = "Invalid input!";
}
else
{
labelStatusInput.Text = "Input: OK";
}
}
Would this be a viable option for your use case?
Hi, I have added below code for testing purpose. Can you let me know where I can add data to listen those change in textbox :
class script{
public void Run(Engine engine){
controller = new InteractiveController(engine);
InputParams inputDialog = new InputParams(engine);
string serviceName = inputDialog.serviceNameTb.Text;
}
public class InputParams : Dialog
{
public Label LbiErrorInput = new Label(string.Empty);
private readonly Label serviceNameLabel = new Label(“Service Name: “);
public InputParams(Engine engine) : base(engine)
{
//Service Name Tb
serviceNameTb = new TextBox
{
Width = 250,
};
// Define layout
AddWidget(serviceNameLabel, 3, 0);
AddWidget(serviceNameTb, 3, 1);
AddWidget(LbiErrorInput, 6, 0);
}
public TextBox serviceNameTb { get; set; }
}

Hi Aditiben,
It’s possible to define that listener where you initialized the dialog within your run method.
Or create a small ‘Presenter’ class according to the Model-View-Presenter pattern to abstract the view from the presenting logic.
The result could be something like this:
public void Run(IEngine engine)
{
controller = new InteractiveController(engine);
InputParams inputDialog = new InputParams(engine);
InputParamsPresenter presenter = new InputParamsPresenter(inputDialog);
inputDialog.BtnConfirm.Pressed += (sender, args) =>
{
if (presenter.ValidateServiceName(inputDialog.ServiceNameTb.Text))
{
// Valid service name -> perform next actions
// Eg. controller.Run(nextDialog);
var progressDialog = new ProgressDialog(engine) { Title = “Result” };
progressDialog.OkButton.Pressed += (o, eventArgs) => engine.ExitSuccess(“OK”); // Make sure the OK button does something
progressDialog.AddProgressLine(“Resulting service name: ” + inputDialog.ServiceNameTb.Text);
controller.ShowDialog(progressDialog); // show this dialog to the user instead
}
};
controller.Run(inputDialog);
}
public class InputParams : Dialog
{
private readonly Label serviceNameLabel = new Label(“Service Name: “);
public Label LbiErrorInput { get; } = new Label(string.Empty);
public TextBox ServiceNameTb { get; } = new TextBox { Width = 250 };
public Button BtnConfirm { get; } = new Button(“Confirm”);
public InputParams(IEngine engine) : base(engine)
{
// Define layout
AddWidget(serviceNameLabel, 3, 0);
AddWidget(ServiceNameTb, 3, 1);
AddWidget(LbiErrorInput, 6, 0);
AddWidget(BtnConfirm, 7, 0);
}
}
public class InputParamsPresenter
{
private readonly InputParams dialog;
public InputParamsPresenter(InputParams dialog)
{
this.dialog = dialog;
// Define events
dialog.ServiceNameTb.Changed += ServiceNameTbOnChanged;
}
public bool ValidateServiceName(string serviceName)
{
Regex regex = new Regex(“*”); // update pattern to match
if (!regex.IsMatch(serviceName))
{
dialog.LbiErrorInput.Text = “Invalid input!”;
return false;
}
else
{
dialog.LbiErrorInput.Text = “Input: OK”;
return true;
}
}
private void ServiceNameTbOnChanged(object sender, TextBox.TextBoxChangedEventArgs e)
{
ValidateServiceName(e.Value);
}
}
I see that this question has been inactive for some time. Do you still need help with this? If not, could you select the answer (using the ✓ icon) to indicate that the question is resolved?