Has anyone had success in building a Mocking Library to mimic the SLProtocol/SLProtocolExt for unit testing for protocol development?
Hi Rebecca!
We have some videos explaining how to do unit tests and in this part we go through the process of mocking the SLProtocol.
You can watch the video or read a bit of the explanation.
Mainly, I use the Moq library as the example here:
[TestMethod()] public void Rewrite_PathWithItemsToAbbreviate_ReturnsAbbreviatedPath() { // Arrange PathRewriter pathRewriter = new PathRewriter(); string path = @"Visios\Customers\Skyline\Protocols\Test"; string expected = @"V\C\Skyline\P\Test"; var fakeSlProtocol = new Mock<SLProtocol>(); // Act string result = pathRewriter.Rewrite(fakeSlProtocol.Object, path); // Assert Assert.AreEqual(expected, result); }
Hope that helps!
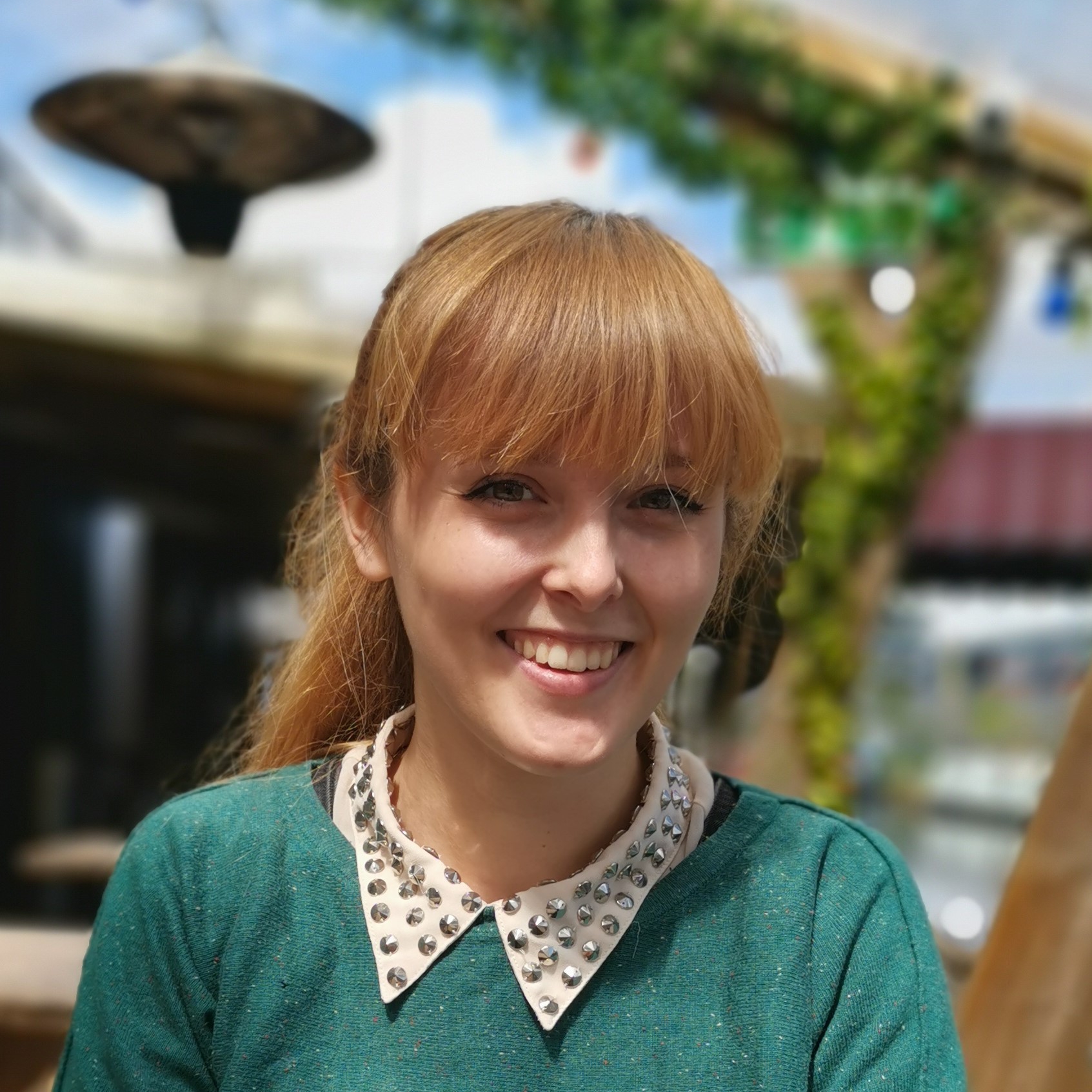
Hi,
Since DataMiner 10.0, the SLProtocol/SLProtocolExt classes have been changed into interfaces. That makes it possible to mock it for unit testing.
If a Nuget with a mocking framework, like Moq, is being used then it's possible to write unit tests and mock the SLProtocol calls.
For example:
Mock<SLProtocol> protocolMock = new Mock<SLProtocol>();
protocolMock.Setup(p => p.DataMinerID).Returns(dmaId);
protocolMock.Setup(p => p.ElementID).Returns(elementId);
protocolMock.Setup(p => p.ElementName).Returns("Dummy Element Name");
protocolMock.Setup(p => p.GetParameter(testParameterId1)).Returns("DummyValue1");
protocolMock.Setup(p => p.GetParameter(testParameterId2)).Returns("DummyValue2");// Using the mocked SLProtocol
SLProtocol protocol = protocolMock.Object;
string param1 = Convert.ToString(protocol.GetParameter(testParameterId1));
protocol.SetParameter(testSetParameterId, "DummyValue")// Asserting
Assert.AreEqual(param1, "DummyValue1");
protocolMock.Verify(p => p.SetParameter(testSetParameterId, It.Is<string>(incomingValue => VerifyIncomingValue(incomingValue)))); // verify that a setparameter has been called with the expected value// For larger collections, FluentAssertions can be used to have a more easy verification when asserting.
Regards,
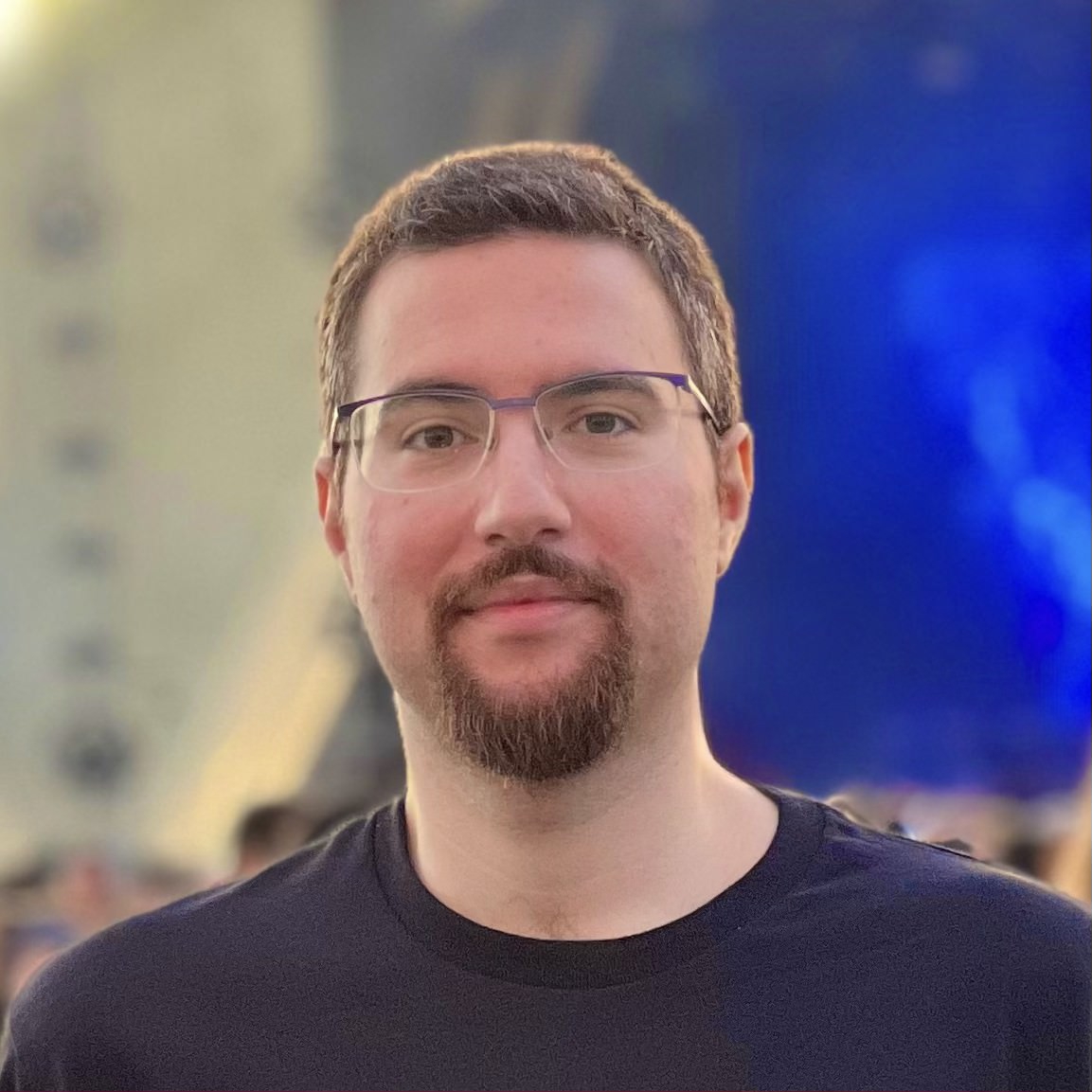
Thanks Laurens, we’ve been able to mock both setting and getting of standalone parameters and tables however, for tables it has proven to be quite an involved process as we’ve been required to mock the NotifyProtocol method which depends on an object array representation of tables that is hard to mimic in code. Are there any wrappers or libraries built from Skyline which can aid in mocking table manipulation behaviour in a more object-oriented manner?
Alternatively, should we be using other methods to read and write into tables?

Hi,
Mocking the NotifyProtocol calls is indeed a bit more work.
If the unit test can run against a more recent DataMiner version (10.1.1 (RN 27995) onwards, then some notifies can be mocked with the alternative method. For example NotifyProtocol(149 /*NT_ADD_ROW*/) has an alternative method protocol.AddRow(). NotifyProtocol(220 /*NT_FILL_ARRAY_WITH_COLUMN*/) has as alternative protocol.FillArrayWithColumn(). These methods used to be extension methods that could not be mocked, but since 10.1.1 this has become part of the SLProtocol interface.
Of course some of these methods and the NotifyProtocol method still could rely on object arrays as input or return value and then one still needs to create these objects.
For example to simulate the response of a NotifyProtocol 321, it could then look like this:
object[] tableInputCols = new object[4];
tableInputCols[0] = pkCol;
tableInputCols[1] = inputLabelCol;
tableInputCols[2] = stateCol;
tableInputCols[3] = lockedCol;
protocolMock.Setup(p => p.NotifyProtocol(321, inputsTableParameterId, It.Is(ui => ui.Length == 4 && ui[0] == 0 && ui[1] == 1 && ui[2] == 2 && ui[3] == 3))).Returns(tableInputCols);
If the assertion of the executed calls is a bit larger, then custom created methods could be created to make it more readable.
protocolMock.Verify(p => p.NotifyProtocol(220, It.Is(tablePids => VerifyTablePidInfo(tablePids, expectedTablePids)), It.Is(tableContent => VerifyTableInfo(tableContent, expectedTableContent))));
The methods VerifyTablePidInfo() and VerifyTableInfo() then validate the content of the objects that were used to execute that notify 220 and return a boolean if it was as expected or not.
An alternative for the verification of the content of received object arrays is to use the FluentAssertions NuGet package in the unit test. Then it’s possible to execute something like expectedDataObjectArray.Should().BeEquivalentTo(receivedDataObjectArray); , if the content is not equal then the unit test will fail.
As far as I’m aware there are no wrappers or libraries yet available to help mock or validate the table manipulation calls.
There does exist a framework that allows writing unit tests and mocking SLProtocol in a way that you just provide what your expected end-result should be. It doesn't care if you used setcolumn, setrow, fillarray and will just check if your 'final table data' matches what you expect.
This was being worked on by several devops engineers when they encountered the same difficulties after we introduced unit testing for QActions.
I believe this will likely help. You'll lose some details on how you perform sets, which can also be important! But it does help a lot to avoid regression when refactoring and writing tests quicker.
That library is currently a NuGet that's still internal and in beta. But it's going through the last few steps to make it public.
I can check with our PO and the developers of the library to give it a little push.
Example
```csharp
[TestMethod()]
[DeploymentItem(@"TestFiles\response_content.xml")]
public void TestCallback()
{
// Arrange
var protocolModel = new ProtocolModelExt(path);
var protocolMock = new SLProtocolMock(protocolModel);
string responseContent = File.ReadAllText("response_content.xml");
var response = new HttpResponse("uri", "HTTP1.1 200 OK", responseContent);
var callback = new GetMediaUsageByUtRangeResponse();
// Act
callback.Run(protocolMock.Object, response);
// Assert
mock.Assert()
.Table(Parameter.Mediainstancesusagetable.tablePid)
.Column(Parameter.Mediainstancesusagetable.Pid.mediainstancesusagetableid_2401)
.Should()
.Contain("test");
}
```
another example:
```csharp
# AddRow
// Create a new Protocol Model
var protocolModel = new ProtocolModelExt(path);
// Create a new SLProtocolMock and pass it the Protocol Model
var mock = new SLProtocolMock(protocolModel);
// The row to be set
object[] row1 = new object[] { "one.1", "two.1", "three.1", "four.1", "five.1" };
object[] row2 = new object[] { "one.2", "two.2", "three.2", "four.2", "five.2" };
// Make a call using the mock object, here in this example we are adding two rows, row1 and row2, to the table with ID 900
mock.Object.AddRow(900, row1);
mock.Object.AddRow(900, row2);
// Define the expected column values
string[] expectedColumn1 = { "one.1", "one.2" };
string[] expectedColumn2 = { "two.1", "two.2" };
string[] expectedColumn3 = { "three.1", "three.2" };
string[] expectedColumn4 = { "four.1", "four.2" };
string[] expectedColumn5 = { "five.1", "five.2" };
// Invoke the mock assert method, select the ID of the Table and the column to check, and then compare it with the expected column
mock.Assert().Table(900).Column(901).Should().Equal(expectedColumn1);
mock.Assert().Table(900).Column(901).Should().Equal(expectedColumn2);
mock.Assert().Table(900).Column(901).Should().Equal(expectedColumn3);
mock.Assert().Table(900).Column(901).Should().Equal(expectedColumn4);
mock.Assert().Table(900).Column(901).Should().Equal(expectedColumn5);
```
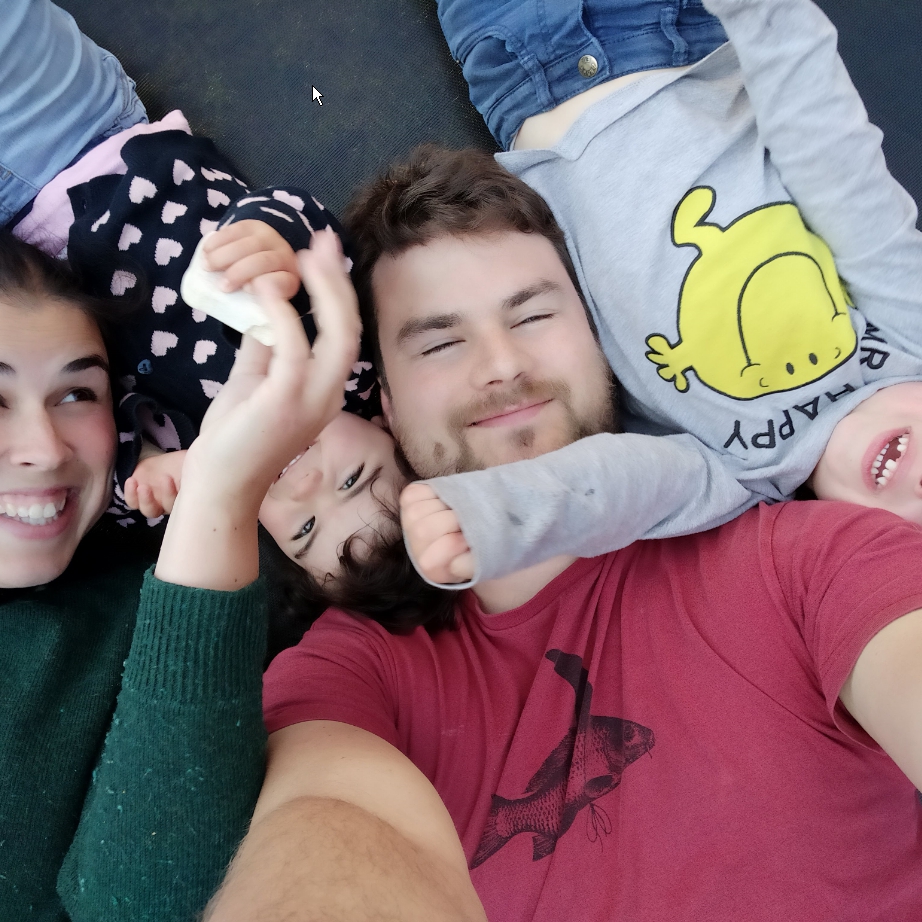
Hi, Any update on this? Where can community users find the NuGet? Thank you
Thank you for your answer. We are already using Moq similar to the above but, we were hoping somebody had already built an independant helper library that mocked things like the NotifyProtocol to help with validating table calls that are represented as object arrays which can be hard to write mocks for.