Has anyone had success in building a Mocking Library to mimic the SLProtocol/SLProtocolExt for unit testing for protocol development?
There does exist a framework that allows writing unit tests and mocking SLProtocol in a way that you just provide what your expected end-result should be. It doesn't care if you used setcolumn, setrow, fillarray and will just check if your 'final table data' matches what you expect.
This was being worked on by several devops engineers when they encountered the same difficulties after we introduced unit testing for QActions.
I believe this will likely help. You'll lose some details on how you perform sets, which can also be important! But it does help a lot to avoid regression when refactoring and writing tests quicker.
That library is currently a NuGet that's still internal and in beta. But it's going through the last few steps to make it public.
I can check with our PO and the developers of the library to give it a little push.
Example
```csharp
[TestMethod()]
[DeploymentItem(@"TestFiles\response_content.xml")]
public void TestCallback()
{
// Arrange
var protocolModel = new ProtocolModelExt(path);
var protocolMock = new SLProtocolMock(protocolModel);
string responseContent = File.ReadAllText("response_content.xml");
var response = new HttpResponse("uri", "HTTP1.1 200 OK", responseContent);
var callback = new GetMediaUsageByUtRangeResponse();
// Act
callback.Run(protocolMock.Object, response);
// Assert
mock.Assert()
.Table(Parameter.Mediainstancesusagetable.tablePid)
.Column(Parameter.Mediainstancesusagetable.Pid.mediainstancesusagetableid_2401)
.Should()
.Contain("test");
}
```
another example:
```csharp
# AddRow
// Create a new Protocol Model
var protocolModel = new ProtocolModelExt(path);
// Create a new SLProtocolMock and pass it the Protocol Model
var mock = new SLProtocolMock(protocolModel);
// The row to be set
object[] row1 = new object[] { "one.1", "two.1", "three.1", "four.1", "five.1" };
object[] row2 = new object[] { "one.2", "two.2", "three.2", "four.2", "five.2" };
// Make a call using the mock object, here in this example we are adding two rows, row1 and row2, to the table with ID 900
mock.Object.AddRow(900, row1);
mock.Object.AddRow(900, row2);
// Define the expected column values
string[] expectedColumn1 = { "one.1", "one.2" };
string[] expectedColumn2 = { "two.1", "two.2" };
string[] expectedColumn3 = { "three.1", "three.2" };
string[] expectedColumn4 = { "four.1", "four.2" };
string[] expectedColumn5 = { "five.1", "five.2" };
// Invoke the mock assert method, select the ID of the Table and the column to check, and then compare it with the expected column
mock.Assert().Table(900).Column(901).Should().Equal(expectedColumn1);
mock.Assert().Table(900).Column(901).Should().Equal(expectedColumn2);
mock.Assert().Table(900).Column(901).Should().Equal(expectedColumn3);
mock.Assert().Table(900).Column(901).Should().Equal(expectedColumn4);
mock.Assert().Table(900).Column(901).Should().Equal(expectedColumn5);
```
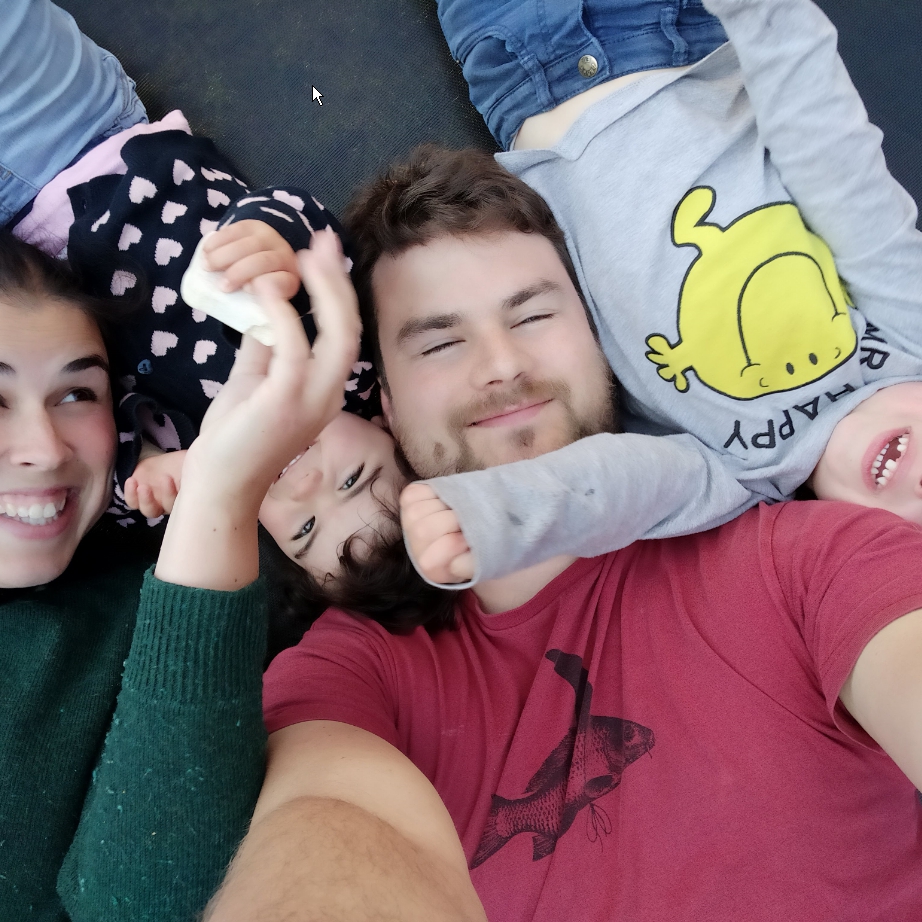
Hi, Any update on this? Where can community users find the NuGet? Thank you