Hi,
I have an adhoc GQI data source which creates this table:
public GQIColumn[] GetColumns()
{
return new GQIColumn[]
{
new GQIStringColumn("Date"),
new GQIStringColumn("Script Number"),
new GQIIntColumn("SKNs"),
new GQIStringColumn("Code"),
new GQIStringColumn("Title")
};
}
public GQIPage GetNextPage(GetNextPageInputArgs args)
{
var items = _jammDbHelper.GetData();
var rows = new List<GQIRow>();
foreach (var item in items)
{
var cells = new GQICell[]
{
new GQICell() { Value = item.Date.Value.ToString("dd/MM/yyyy") },
new GQICell() { Value = item.ScriptNumber },
new GQICell() { Value = item.ProductNumbers.Count},
new GQICell() { Value = item.ShowCode},
new GQICell(){ Value = item.ShowName},
};
var row = new GQIRow(cells);
rows.Add(row);
}
return new GQIPage(rows.ToArray());
}
And this produces a nice table on the low code app. However what I'd ideally like is 1 table on the UI for each data set where it's grouped by Date. So we only see the date once, but then the rest of the info for that date.
The user can currently do the grouping in 1 table by right clicking the column and choosing group, however this is removed the next time the app is loaded.
So my questions are: Can I force the grouping on that specific column by default so the user doesn't have to click it every time? Or, even better, can I create 4 separate tables from that 1 data source if I apply a group somewhere and create a table for each group?
I'm wondering if this is possible without me having to create 4 different Adhoc data sources and assigning 1 to each table that I want on the UI
Thanks!
Hi Carl,
This is indeed possible, there are several solutions which might fit your use-case:
Applying the grouping for all users
After clicking the arrow in front of the query you can see the columns. Dragging and dropping the column in the 'Group' box will apply the grouping. The difference with the approach you described, is that, in this case, the grouping will be applied for all the users.
Add a filter operator to create the groups
Not sure if that will work in your use-case but you could also create 4 different queries each having a different filter operator. The filter operator makes sure only the data for that certain group is visible. Through this, you can have 4 different tables. Do note that this will, with your current implementation, fetch the data 4 times.
Extend the ad hoc data source with an input argument
Something else you could possibly do is introducing an input argument, which indicates for what group the ad hoc datasource should fetch the data. Your ad hoc script can act based on that input argument.
More info on how to add these input arguments: https://docs.dataminer.services/user-guide/Advanced_Modules/Dashboards_and_Low_Code_Apps/GQI/Extensions/Ad_hoc_data/Ad_hoc_Tutorials.html
Hope this helps, feel free to let me know if anything is unclear!
Best regards, Ward
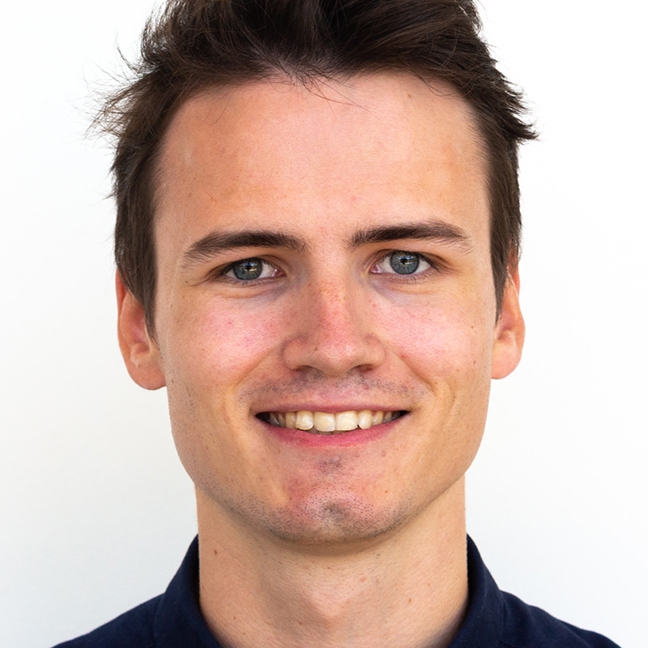
Hi Carl, yes that’s possible!
What you could do is, for example, create a GQIIntArgument, indicating the amount of days to add. (0, 1, 2 …) In your ad hoc script you can retrieve that value and provide it to the AddDays method.
Best regards, Ward
Hi Carl,
I think a good option is to make use of an input parameter. In the documentation, there is an example, where a minimum age is used as an input parameter to only show the people older than that minimum age. Analogously, you could use a date as an input parameter to show only the rows of that specific date.
In this way, you avoid having to make 4 different Ad Hoc Data Sources.
You even could go one step further and let that input parameter come from a Feed, for example, a date you select in a dropdown. I would advise however to implement this step by step, so as a first step, you could try with a hardcoded date.
Kind regards,
Joachim
Thanks so much for the pointers, the input argument looks promising. However if I’m grouping by Date, I would want the input parameter to be defined dynamically for each data source.
For example, my API always returns 4 days worth of data from DateTime.Today
So when specifying the input parameter on the low code UI, I don’t want to just select a date or type a date to group by, but I want to use DateTime.Today for source 1, and DateTime.Today.AddDays(1) for source 2, etc etc.
Is this possible?
Thanks, Carl