I have multiple service data and I fetch and then on that I iterate the elements to get some info and then build the cells, which sometimes I see it goes on time out:
For createLEmentRow:
public GQIPage GetNextPage(GetNextPageInputArgs args)
{
const int batchSize = 25;
var rows = new List<GQIRow>();
var serviceList = GetElements();
_logger.Information("SERVICE LIST -- " + serviceList.Count);
Parallel.ForEach(serviceList, service =>
{
_logger.Information("ServiceName - " + service.Name);
Parallel.ForEach(service.Children, childInfo =>
{
var localRows = new List<GQIRow>();
CreateElementRow(localRows, childInfo, service.Name);
lock (_allRows)
{
_allRows.AddRange(localRows);
_logger.Information("ALL ROWS Length -- " + _allRows.Count);
}
private void CreateElementRow(List<GQIRow> rows, LiteServiceChildInfo element, string name)
{
var cells = Enumerable.Repeat(new GQICell { Value = "" }, _resources.Count + 2).ToArray();
var lockObject = new object(); // Lock object for thread safety
// Separate parameters into two groups
var group1 = element.Parameters.Where(p => p.ParameterID >= 7004 && p.ParameterID <= 7035).ToList();
var group2 = element.Parameters.Where(p => p.ParameterID >= 703 && p.ParameterID <= 1908).ToList();
// Define a method to process a group of parameters
void ProcessGroup(List<ServiceParamFilter> group, int endParameterId, string groupName)
{
foreach (var parameter in group)
{
var data = _resources.Find(x => x.ParamterId.Equals(parameter.ParameterID));
if (data == null)
{
continue;
}
_logger.Information($"START {groupName}");
lock (lockObject)
{
BuildCells(parameter, element, ref cells, rows);
}
........
private void BuildCells(ServiceParamFilter parameter, LiteServiceChildInfo element, ref GQICell[] cells,
List<GQIRow> rows)
{
string cellValue = string.Empty; // Default value.
ResourcesDetails data = null;
data = _resources.Find(x => x.ParamterId.Equals(parameter.ParameterID));
if (data == null)
{
return;
}
try
{
var requestMsg = new GetParameterMessage(element.DataMinerID, element.ElementID,
parameter.ParameterID,
parameter.FilterValue, true);
var paramInfo = _dms.SendMessage(requestMsg) as GetParameterResponseMessage;
//var paramInfo = responseMsg.ChangeType<GetParameterResponseMessage>().OfType<GetParameterResponseMessage>().FirstOrDefault();
cellValue = paramInfo.Value.ToString().Equals("EMPTY", StringComparison.CurrentCultureIgnoreCase)
? string.Empty
: paramInfo.Value.ToString();
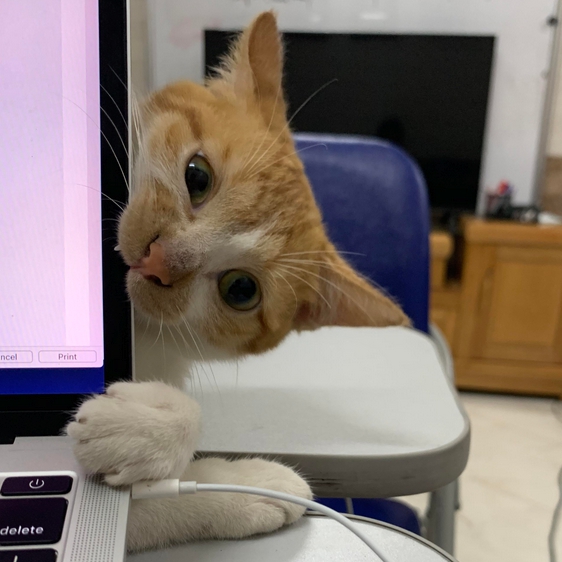
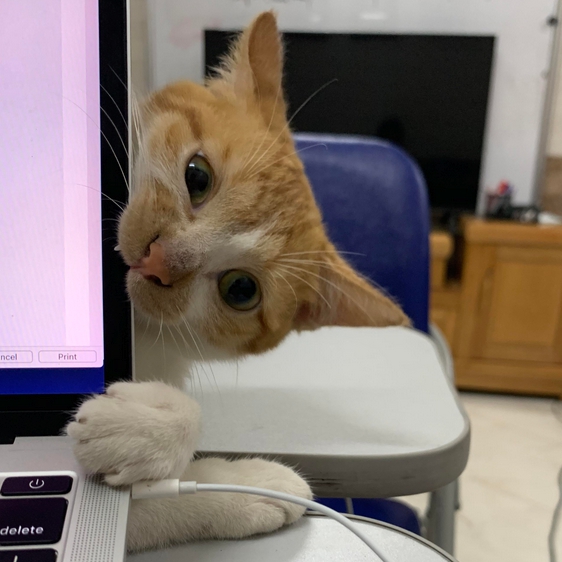
As this question has now been inactive for a very long time, I will close it. If you still want more information about this, could you post a new question?
If you say it goes in time out -sometimes-, how long does it usually take when it doesn't go in time out? Could there be any meaningful difference in the data between those scenarios? How many services, elements, parameters are we talking about?
If it usually takes well below 30s, there might be a race condition or deadlock.
Otherwise, if it's not uncommon to take that long, it's probably better not to execute this computation in a query for a Dashboard or Low-code app, since these are only meant to do relatively quick on-the-fly queries.
Depending on your use-case, you could look into using e.g. the Data Aggregator to compute and store this aggregated data in the background at regular intervals independently from your app. (See: Data Aggregator | DataMiner Docs)
I see that this question has been inactive for some time. Do you still need help with this? If not, could you select the answer (using the ✓ icon) to indicate that the question is resolved?