I'd like to send a command from a protocol to retrieve data from a Linux server via SSH.
However that command needs sudo elevated privileges. The response I retrieve is just the text that is asking for a username/pwd.
How can I do this from a protocol, please?
Hi Jochen,
I created a small automation script that send a command that requires sudo privileges. I believe this can also be implemented in a connector. In this case you will need to use ShellStream to be able to get the sudo password request:
public void Run(Engine engine)
{
string sLogin = "myUser";
string sPassword = "myUserPassword";
string sudoPassword = "myRootPassword";
string sHostname = "10.11.12.13";
string sCommand = "sudo dmidecode -t system | grep Serial";
string sExpectedResponse = "";
int iPort = 22;SshClient sshClient = new SshClient(sHostname, iPort, sLogin, sPassword);
// Establish the connection
sshClient.Connect();IDictionary<Renci.SshNet.Common.TerminalModes, uint> modes = new Dictionary<Renci.SshNet.Common.TerminalModes, uint>();
modes.Add(Renci.SshNet.Common.TerminalModes.ECHO, 53);// Create a shell stream
var shellStream = sshClient.CreateShellStream("xterm", 255, 50, 800, 600, 1024, modes);// Get logged in
sExpectedResponse = shellStream.Expect(new Regex(@"[$>]"));
engine.GenerateInformation("[INFO]|Run|Output:" + sExpectedResponse);// Send command that requires sudo privileges
shellStream.WriteLine(sCommand);// Expect password or user prompt
sExpectedResponse = shellStream.Expect(new Regex(@"([$#>:])"));
engine.GenerateInformation("[INFO]|Run|Output:" + sExpectedResponse);// Check to send the password
if (sExpectedResponse.Contains(":"))
{
// Send password
shellStream.WriteLine(sudoPassword);// Expect user or root prompt
sExpectedResponse = shellStream.Expect(new Regex(@"[$#>]"));engine.GenerateInformation("[INFO]|Run|Output:" + sExpectedResponse);
}// End ifsshClient.Disconnect();
}// End method Run
For this example, I was able to get the serial number:
The main drawback of this approach is that the response will contain extra characters that you don't need (see screenshot above). Based on this, I believe it will be difficult to extract the information that you are looking for.
I saw other options as well, see this answer in StackOverflow, but they are insecure.
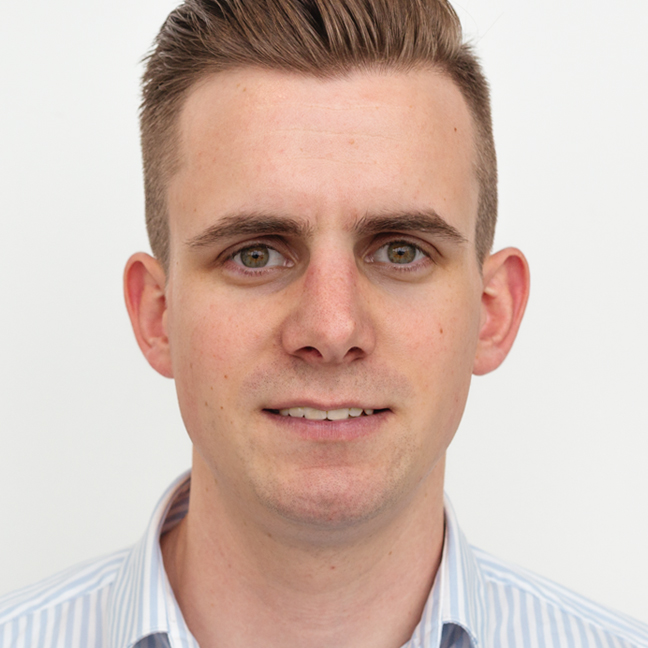
For automation script, that’s ok. But for protocol that’d mean I have to put everything in QActions, that’s not ideal…