Hi,
In a QAction in C#, I try to retrieve a value in a table ID 1000. The parameter is a string called “Subscription ID” and is also the IDX of the table. I use the following command to proceed in a “for {}” loop:
for (int i = 1;i <= row ; i++)
{
string sI = i.ToString();
subscription_ID = Convert.ToString(protocol.GetParameterIndexByKey(1000, sI, 1));
…}
The table is 35 row length but for each of them I receive the following error message:
NT_GET_PARAMETER_INDEX for 1000/1/1 failed. 0x80040221
NT_GET_PARAMETER_INDEX for 1000/2/1 failed. 0x80040221
etc …
Here follows the header of the table:
<Param id=”1000″ trending=”true”>
<Name>Remotes Status</Name>
<Description>Remotes Status</Description>
<Type>array</Type>
<ArrayOptions index=”0″>
<ColumnOption idx=”0″ pid=”1001″ type=”retrieved” value=”” options=”” />
<ColumnOption idx=”1″ pid=”1002″ type=”r ….
I use the same method to retrieve parameter from another table and it works correctly. Both tables are filled with data. So why would it work fine with one table but not with another?
I will be very grateful for any idea:)
Hi Dominique
Instead of retrieving cell by cell, you can retrieve the entire column at once with the following call: NT_GET_TABLE_COLUMNS (321) | DataMiner Docs
When using DIS there is also a snippet for this call. This way you reduce the amount of protocol calls (performance) and you get the data all at once.
EDIT: I’ve included a small code example how to retrieve the PK & the column that contains the subscription ID (you’ll have to change it to the matching names from your protocol).
var columnIndexes = new uint[] { Parameter.RemotesStatus.indexColumn, Parameter.RemotesStatus.Idx.remotesStatusSubscriptionId };
var columns = (object[])protocol.NotifyProtocol((int)SLNetMessages.NotifyType.NT_GET_TABLE_COLUMNS, Parameter.RemotesStatus.tablePid, columnIndexes);var keys = (object[])columns[0];
var values = (object[])columns[1];for (int i = 0; i < keys.Length; i++)
{
string key = Convert.ToString(keys[i]);
string subscriptionId = Convert.ToString(values[i]);// Use the subscriptionId to get the remote status via API. Or store in a list to call in bulk later in code
}
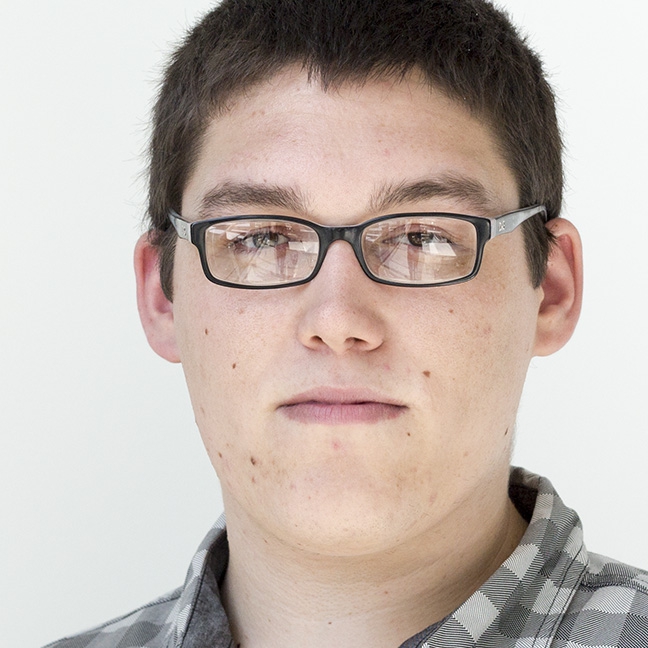
Hi Dominique
I've updated my answer with a small code example on how to use the getcolumn call. As you can see, it's very similar to your original code with the difference it can deal with a variable amount of rows. If extra data is needed, you can extend the call and use it within the same forloop.
Hi Michiel,
I am now trying to implement your code. But, as i am not familiar with this method, how do I need to configure the columnIndexes and columns variable compare to my table?
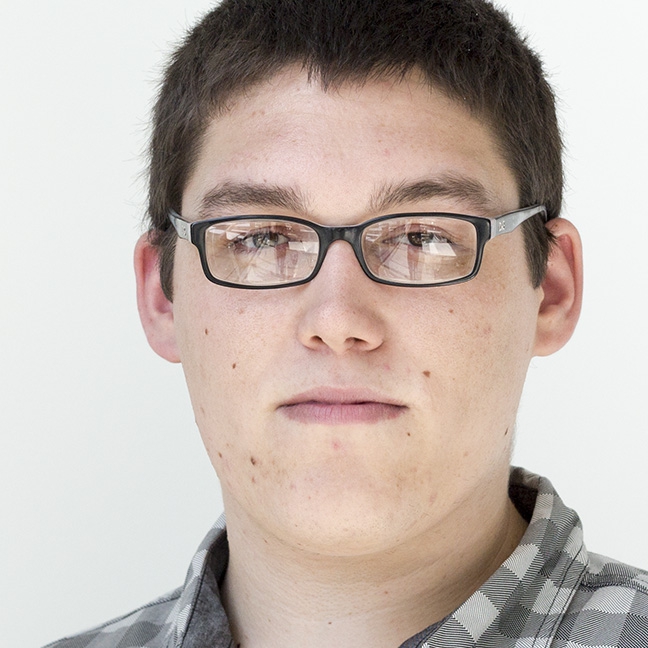
You can update the columnIndexes to refer to the column indexes you actually want to retrieve (matches the idx attribute in the XML). I use the Parameter class to give it a readable name instead of numbers, but that is up to you.
For each entry in the columnIndexes, a new object will in the columns variable. As you can see in my example, I retrieve 2 columns (PK + another one). As such I extract the 2 columns after the protocol call to their own object arrays so I can loop over it. If you want to retrieve more or less columns, you can add or remove the 'var xxx = (object[])columns[x];'.
Hi Michiel,
In fact I just need to retrieve one parameter per line to build my API request. So the question is why it is not working for my table 1000 but it does work to retrieve individual column? For example,
Terminal_ID = Convert.ToString(protocol.GetParameterIndexByKey(2000, sI, 3)); works fine but
subscription_ID = Convert.ToString(protocol.GetParameterIndexByKey(1000, sI, 1)); doesn't work. However, I don't see any syntax error and both tables exist.
The main difference between table 1000 and table 2000 is that in table 1000 parameter type is "retrieved" and in table 2000, it is "custom"…
Please I just need advice on that 🙂
Hi Michiel,
Before I can try your code, do you see anything wrong with my line to retrieve the “subscription_ID” parameter:
subscription_ID = Convert.ToString(protocol.GetParameterIndexByKey(1000, sI, 1));
Knowing that “1000” is the table ID, sI is the row # starting from 1 and “1” is the column # where my data is located.
Thank you Michiel, well noted. However, in this case I need to retrieve each ocurence of that table which in turn will be part of an API call to exctract data from my vendor site. The API string is built in the "sUrl" string variable as follow:
sUrl = "ext/api/v1/whs/services/" + sid + "/subscriptions/";
sUrl += subscription_ID; // Append Subscription ID
sUrl += "/data/rf?time_span_key=12Hours";
But the result is the field between "/subscriptions/" and "/data …" is empty:
"ext/api/v1/whs/services/200396/subscriptions//data/traffic?time_span_key=12Hours"
And in the log, error 0x80040221 appears for each instance.