The latest version of DIS, 2.32.1, comes with an update of the class library. This new version 1.2.0.5 of the class library features support for creating, updating and retrieving HTTP connections of elements, and for creating and deleting DataMiner properties.
Creating, updating, and retrieving HTTP connections of elements
We have added support for HTTP connections of elements (virtual and SNMP connections were already supported). Here is an overview of the provided interfaces:
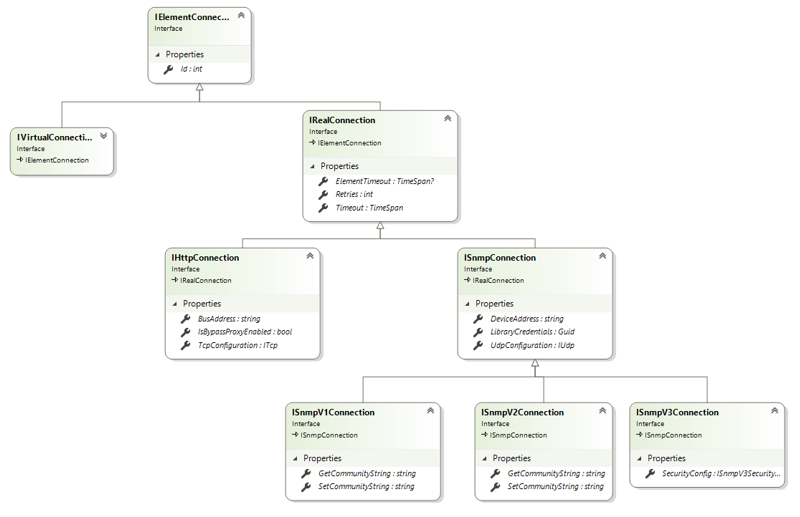
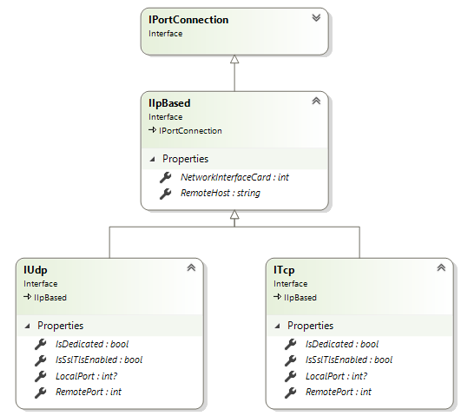
The following example illustrates how to create an element with an HTTP connection:
private static void CreateElement(SLProtocol protocol) { IDms dms = protocol.GetDms(); IDma agent = dms.GetAgent(protocol.DataMinerID); IDmsProtocol elementProtocol = dms.GetProtocol("MyProtocolName", "1.0.0.1"); ITcp port = new Tcp("127.0.0.1", 8888); IHttpConnection myHttpConnection = new HttpConnection(port); var configuration = new ElementConfiguration( dms, "MyElementName", elementProtocol, new List<IElementConnection> { myHttpConnection }); DmsElementId createdElementId = agent.CreateElement(configuration); }
This next example illustrates how to update an HTTP connection of an existing element:
private static void SetPort(SLProtocol protocol) { IDms myDms = protocol.GetDms(); var element = myDms.GetElement("myHttpElement"); int portNumber = 8888; ElementConnectionCollection connections = element.Connections; // We assume that in this example we know the first connection is the HTTP connection. if (connections.Length > 0) { var httpConnection = connections[0] as IHttpConnection; if (httpConnection != null) { var currentPortNumber = httpConnection.TcpConfiguration.RemotePort; if (currentPortNumber != portNumber) { httpConnection.TcpConfiguration.RemotePort = portNumber; // Apply changes. element.Update(); } } } }
Creating and deleting properties in a DataMiner System
We have also added support for creating and deleting element, view or service properties in a DataMiner System. The following example illustrates how you can create an element property:
IDms dms = protocol.GetDms(); string propertyName = "MyCustomElementProperty"; bool isFileterEnabled = true; bool isReadOnly = false; bool isVisibleInSurveyor = true; int propertyId = dms.CreateProperty(propertyName, PropertyType.Element, isFileterEnabled, isReadOnly, isVisibleInSurveyor);
The example below shows how to delete a custom property:
IDms dms = protocol.GetDms(); var elementProperty = dms.ElementPropertyDefinitions["MyCustomElementProperty"]; dms.DeleteProperty(elementProperty.Id);
Reference
For more detailed information, refer to the Skyline.DataMiner.Library.Common namespace section of our DataMiner Development Library.