Hi,
What’s the best way to handle the catch and handle the error “Could not verify that parameter XXXX was correctly set (50 retries).” in an automation script?
Hi Robin,
By default, when doing a set on a parameter, DataMiner will check if the value changed in the corresponding read parameter. The error you encounter is because this check failed (will internally verify 50 times).
This setting can also be disabled, by disabling the following option (when executing the script from Cube):
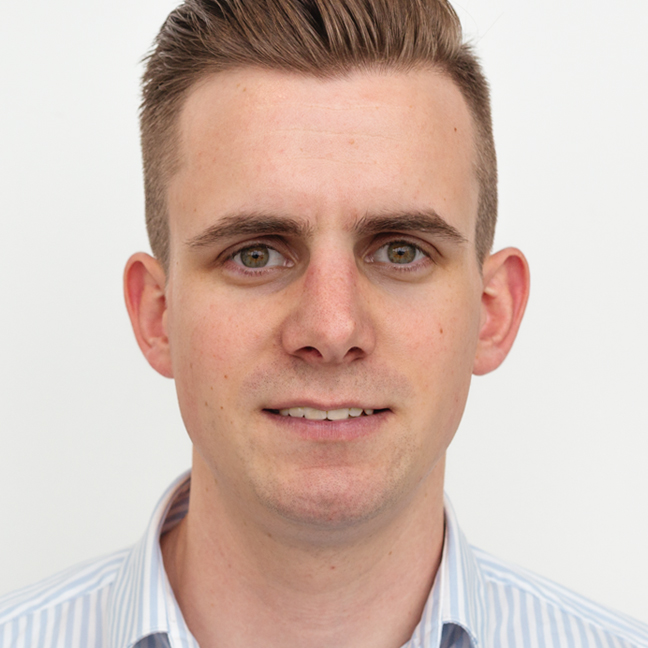
Hi Robin, it’s not async. It doesn’t throw an exception because the SET as such succeeded and there’s no check done after the SET (if you uncheck that checkbox Joey mentioned). So there’s no exception to catch in that case.
If you uncheck the checkbox that Joey mentioned, it’s only going to raise an exception if the SET itself (not the check after SET) fails. E.g. if your parameter doesn’t exist.
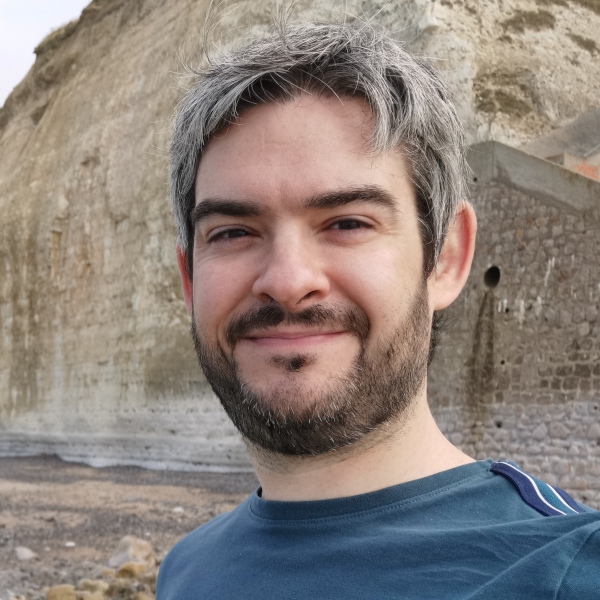
It will indeed be necessary to add a checking mechanism.
Typically this is done in a for loop in which a engine.sleep is added and a parameter read action. The for loop makes sure there’s a maximum amount of read actions and is exited early when the set succeeded.
ScriptDummy dummy = engine.GetDummy(“dummy1”);
bool setSucceeded = false;
dummy.SetParameter(8,15);
for (int i = 0;i<10;i++)
{
engine.Sleep(1000);
int iValue = Convert.ToInt32(dummy.GetParameter(8));
if (iValue == 15)
{
setSucceeded = true;
break;
}
}
if (setSucceeded)
engine.GenerateInformation("the set succeeded");
else
engine.GenerateInformation("the didn't succeed");
@Jeroen: I see. Would this require the write param to be setter=”false” and update the read param programmatically?
Hi Joey,
Sorry for being unclear. I’m actually looking for how to catch the error in the script and execute C# code if the script throws the errors. As of now a script would continue after the SetParameter call without knowing if it failed or not, which might lead to unwanted consequences. Because the method seems to be executing asynchronously, a normal try-catch is not possible.