For an integration we need to create a regular booking via an automation script.
- Is there a code example available or a template where we can start from?
- Are there key items we need to keep in mind?
If you are using SRM Standard solution you can create bookings using one of the two following ways:
- Use method BookingManager.TryCreateNewBooking(Engine engine, Booking bookingData, IEnumerable<Function> functions, IEnumerable<Event> events, IEnumerable<Property> properties, IEnumerable<Group> groups, out ReservationInstance reservationInstance); (documentation below). Here you can find the same information that you see when creating a booking using the wizard. So in argument ‘bookingData’, you define the information that you see in the first screen of the wizard, in the ‘functions’ you define your resources and profiles, those are mandatory. Then you can also define properties, events and groups, these are optional.
//
// Summary:
// Tries to create a new booking using SRM Standard solution.
//
// Parameters:
// engine:
// Link with Skyline DataMiner.
//
// bookingData:
// Booking data, description, times, etc. Mandatory.
//
// functions:
// Functions to include in the booking. Mandatory.
//
// events:
// If Skyline.DataMiner.Library.Solutions.SRM.BookingManager.CustomEvents is true,
// then the desired ones can be filled here. Optional.
//
// properties:
// If Skyline.DataMiner.Library.Solutions.SRM.BookingManager.CustomProperties is
// true, then the desired ones can be filled here. Optional.
//
// groups:
// If filtering is configured in the Service Definition, group filters can be filled
// here. Optional.
//
// reservationInstance:
// The created reservation;otherwise null.
//
// Returns:
// True if the booking was created;otherwise false.
public bool TryCreateNewBooking(Engine engine, Booking bookingData, IEnumerable<Function> functions, IEnumerable<Event> events, IEnumerable<Property> properties, IEnumerable<Group> groups, out ReservationInstance reservationInstance);
E.g.: Bellow an example, taken for one of your test scripts:
var start = DateTime.SpecifyKind(DateTime.Now.AddDays(1), DateTimeKind.Local);
var end = DateTime.SpecifyKind(start.AddHours(1), DateTimeKind.Local);var functions = FunctionsFactory.GetSatelliteDownlink(
TestSystemIds.Resources.Satellite.EUTELSAT_10A,
TestSystemIds.Resources.Antenna.MGR_ANTENNA_01,
TestSystemIds.Resources.Demodulating.MGR_MDM6100_01,
TestSystemIds.Resources.Decoding.KFS_RX8200_01);var bookingData = new Booking
{
Description = $”Booking_{DateTime.Now.ToString(“dd_HH_mm_ss”)}”,
Recurrence = new Recurrence
{
StartDate = startTime,
EndDate = endTime
},
ServiceDefinition = TestSystemIds.ServiceDefinitions.SDMN_SAT_Satellite_Downlink.ToString(),
};var success = this.bookingManager.TryCreateNewBooking(Engine, bookingData, functions, null, null, null, out var reservation);
2. Use method BookingManager.TryCreateNewBooking(Engine engine, Model.DefineBooking.InputData inputData, out ReservationInstance reservationInstance); (documentation below). With this method you will make use of Service Profiles, so you just need define the booking basic information, like timing and naming, and the ServiceProfileInstance that you want to use.
//
// Summary:
// Tries to create a new booking using SRM Standard solution relying on Service
// Profile Data.
//
// Parameters:
// engine:
// Link with Skyline DataMiner.
//
// inputData:
// Input Data that describes the Service Profile Data to be used.
//
// reservationInstance:
// The created reservation;otherwise null.
//
// Returns:
// True if the booking was created;otherwise false.
public bool TryCreateNewBooking(Engine engine, Model.DefineBooking.InputData inputData, out ReservationInstance reservationInstance);
If you are not using the SRM Standard solution you will have to build a ReservationInstance object with all the necessary info and then use ResourceManagerHelper to save schedule your booking.
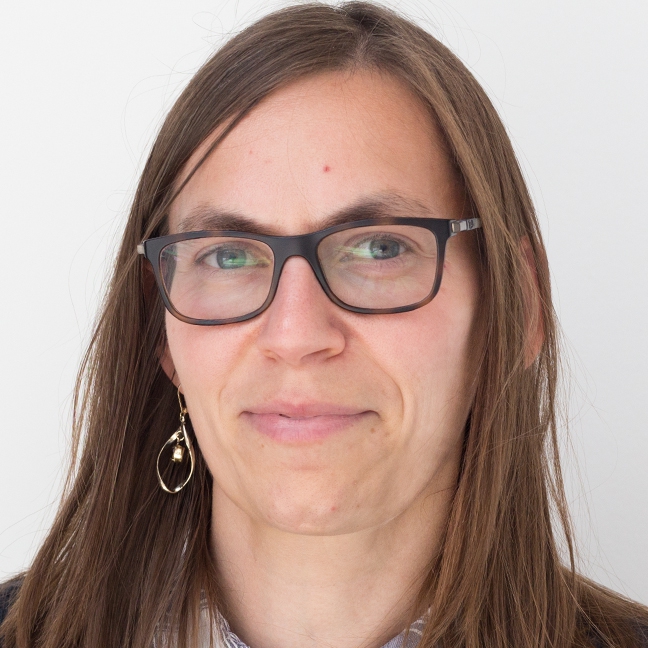
Below you can find a very simple example, of a booking with 1 resource, and two events: one at start and another at end, but there are endless other options to fill.
var reservationInstance = new ReservationInstance(new TimeRangeUtc(DateTime.UtcNow.AddMinutes(1), DateTime.UtcNow.AddMinutes(2)))
{
Name = “BookingName”,
Status = ReservationStatus.Confirmed
};
var resourceUsage = new ServiceResourceUsageDefinition
{
GUID = resourceId, // Set the guid of the desired resource id
RequiredCapabilities = new List(), // Define here your required capabilities, if any
RequiredCapacities = new List(), // Define here your required capacities, if any
NodeConfiguration = new NodeConfiguration
{
ProfileInstanceID = profileInstanceId, // Id of the profile instance that you want to use, if any
ResourceID = resourceId, // Fill again with the desired resource id
OverrideParameters = new ProfileParameterEntry[0], // Define here the parameters that you want to apply in the resource, if any
FunctionID = resourceFunctionId // Id of the function used to create the resource
}
};
reservationInstance.ResourcesInReservationInstance.Add(resourceUsage);
reservationInstance.AddEvent(reservationInstance.Start, new ReservationEvent(“START”, “fill the necessary string to execute the desired script”));
reservationInstance.AddEvent(reservationInstance.End, new ReservationEvent(“END”, “fill the necessary string to execute the desired script”));
var resourceManagerHelper = new ResourceManagerHelper(engine.SendSLNetSingleResponseMessage);
resourceManagerHelper.AddOrUpdateReservationInstances(reservationInstance);
var traceData = resourceManagerHelper.GetTraceDataLastCall();
if (!traceData.HasSucceeded())
{
engine.GenerateInformation(traceData.ToString());
}
Hi Jorge,
I forgot to mention we don’t have a service definition.
I guess we then don’t need to go via the standard solution.
Would you be able to pass me example how to use the ReservationInstance object?