Hello,
I have to create an Automation Script for creating debug elements. Before I create a debug element, I need to make sure that the current license allows for the creation of another element. For example, if the license allows for up to 50 elements and there are already 50 elements present, I would inform the user as to why the debug element was not created. Is it possible to retrieve license information from an Automation Script, and if so, how?
Hi Emir,
You can use SLNet calls to get that info.
Please find below some example code.
using Skyline.DataMiner.Net.Messages;
using Skyline.DataMiner.Automation;
using System.Linq;public class Script
{
public void Run(Engine engine)
{
var infoReqLicensedElements = new GetInfoMessage()
{
Type = InfoType.Licenses
};var responseLicensedElements = (GetLicensesResponseMessage)engine.SendSLNetSingleResponseMessage(infoReqLicensedElements);
var licensedElements = responseLicensedElements.Licenses.ToList().First(lic => lic.StartsWith(“elements”, System.StringComparison.OrdinalIgnoreCase));
engine.GenerateInformation($”Licensed elements: {licensedElements}”);var infoReqActivatedElements = new GetInfoMessage()
{
Type = InfoType.ActivatedLicenseCounters
};var responseActivatedElements = (GetActivatedLicenseCountersResponseMessage)engine.SendSLNetSingleResponseMessage(infoReqActivatedElements);
var licInfo = string.Join(“;”, responseActivatedElements.ActivatedLicenseCounters);
engine.GenerateInformation($”Activated elements: {licInfo}”);}
}
Hope this helps
note: This is an internal call and we do not recommend using this, as it is not officially supported and we cannot guarantee that it will still work in the future. As a rule, you should avoid using SLNet calls, as these are subject to change without notice.
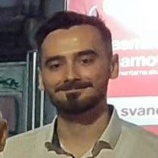
Thank you, I will try this.