I'm developing a driver that gets information from a REST API. I'm retrieving the ping status from the endpoint into the protocol.
The issue appears when this value changes (API Status) — the parameter does not refresh. To force an update, I need to restart the element on the DMA and show current status.
Get 200 status in Wireshark, Qaction detect this but don't refresh parameter
Qaction 101
using Skyline.DataMiner.Scripting;
using System;
using System.Net;
using System.Text.RegularExpressions;
public class TimeoutWebClient : WebClient
{
public int Timeout { get; set; }
public TimeoutWebClient(int timeout)
{
Timeout = timeout;
}
protected override WebRequest GetWebRequest(Uri address)
{
var request = base.GetWebRequest(address);
if (request != null)
{
request.Timeout = Timeout;
if (request is HttpWebRequest httpRequest)
{
httpRequest.ReadWriteTimeout = Timeout;
}
}
return request;
}
}
public class QAction
{
public void Run(Skyline.DataMiner.Scripting.SLProtocol protocol)
{
try
{
string ip = protocol.GetParameter(15) as string;
protocol.Log("IP : " + ip, LogType.Information);
if (string.IsNullOrEmpty(ip) || !ip.Contains("."))
{
protocol.SetParameter(10, "Fail");
protocol.Log("Invalid IP Address", LogType.Error);
return;
}
string url = $"http://{ip}/api/v1/system/network/ping";
var client = new TimeoutWebClient(180000);
client.Headers[HttpRequestHeader.ContentType] = "application/json";
string response = client.DownloadString(url);
protocol.Log("Raw response: " + response, LogType.Information);
var match = Regex.Match(response, "\"ping\"\\s*:\\s*\"([a-zA-Z]+)\"");
if (match.Success)
{
string pingValue = match.Groups[1].Value.ToLower();
protocol.Log("Ping Value: " + pingValue, LogType.Information);
if (pingValue == "ok")
{
protocol.SetParameter(10, "Normal");
}
else
{
protocol.SetParameter(10, "Fail");
}
}
else
{
protocol.SetParameter(10, "Fail");
protocol.Log("No match found in ping response", LogType.Information);
}
}
catch (WebException ex)
{
protocol.SetParameter(10, "Fail");
protocol.Log("Ping WebException: " + ex.Message, LogType.Error);
}
catch (Exception ex)
{
protocol.SetParameter(10, "Fail");
protocol.Log("Ping Exception: " + ex.Message, LogType.Error);
}
}
}
Protocol
<Paramid="10"trending="false"save="true">
<Name>ServerStatus</Name>
<Description>API Status</Description>
<Type>read</Type>
<Information>
<Includes>
<Include>time</Include>
<Include>range</Include>
<Include>steps</Include>
<Include>units</Include>
</Includes>
</Information>
<Interprete>
<RawType>other</RawType>
<Type>string</Type>
<LengthType>next param</LengthType>
</Interprete>
<Alarm>
<Monitored>true</Monitored>
<Normal>Normal</Normal>
<CH>Fail</CH>
</Alarm>
<Display>
<RTDisplay>true</RTDisplay>
<Positions>
<Position>
<Page>General</Page>
<Row>0</Row>
<Column>0</Column>
</Position>
</Positions>
</Display>
<Measurement>
<Type>string</Type>
</Measurement>
</Param>
</QAction>
<QActionid="101"name="PingCheck"encoding="csharp"triggers="10">
</QAction>
</QActions>
<Groups>
<Groupid="10">
<Name>Update Parameters basic</Name>
<Description>Updte info of Titan Live</Description>
<Type>poll</Type>
<Content>
<Param>11</Param>
<Param>12</Param>
<Param>14</Param>
<Param>15</Param>
</Content>
</Group>
<Groupid="20">
<Name>Update Parameters advance</Name>
<Description>Updte info of Titan Live</Description>
<Type>poll</Type>
<Content>
<Param>1101</Param>
<Param>1102</Param>
<Param>1103</Param>
<Param>1104</Param>
<Param>13</Param>
</Content>
</Group>
<Groupid="30">
<Name>Health Startup Group</Name>
<Type>poll action</Type>
<Description>Health API Module</Description>
<Content>
<Action>2</Action>
</Content>
</Group>
<Groupid="40">
<Name>Healthcheck module</Name>
<Type>poll action</Type>
<Description>Health API Module</Description>
<Content>
<Action>2</Action>
</Content>
</Group>
</Groups>
<Triggers>
<Triggerid="1">
<Name>Health trigger</Name>
<Time>after startup</Time>
<On>protocol</On>
<Type>action</Type>
<Content>
<Id>1</Id>
</Content>
</Trigger>
</Triggers>
<Actions>
<Actionid="1">
<Name>After Startup Health Trigger</Name>
<Onid="30">group</On>
<Type>execute</Type>
</Action>
<Actionid="2">
<Name>Call Health Trigger</Name>
<Onid="10">parameter</On>
<Type>run actions</Type>
</Action>
<Actionid="3">
<Name>Login Button Action</Name>
<Onid="300">parameter</On>
<Type>run actions</Type>
</Action>
<Actionid="4">
<Name>Healthcheck Module</Name>
<Onid="10">parameter</On>
<Type>increment</Type>
</Action>
</Actions>
<Timers>
<Timerid="1">
<Name>health check Module</Name>
<Timeinitial="true">160000</Time>
<Interval>180</Interval>
<Content>
<Group>10</Group>
</Content>
</Timer>
</Timers>
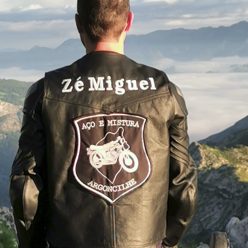
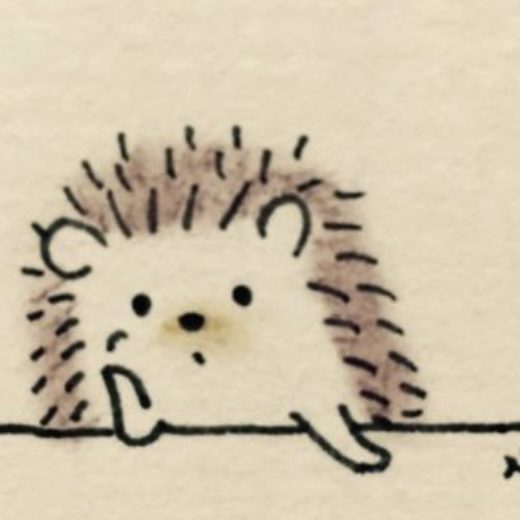
Hi Jose,
was update with images.
Thanks
Jose
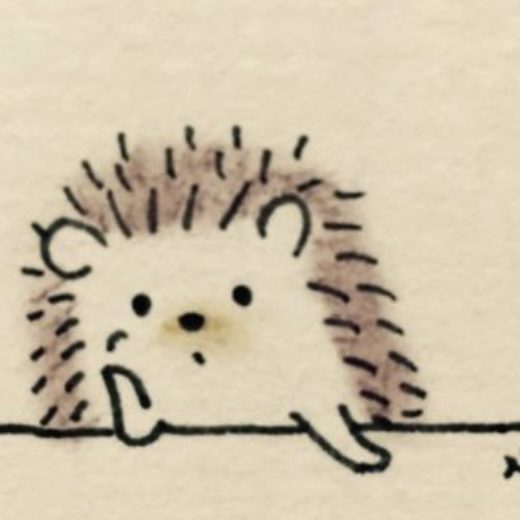
Hi,
This is resolved using dummy parameter and Actions.
Thanks
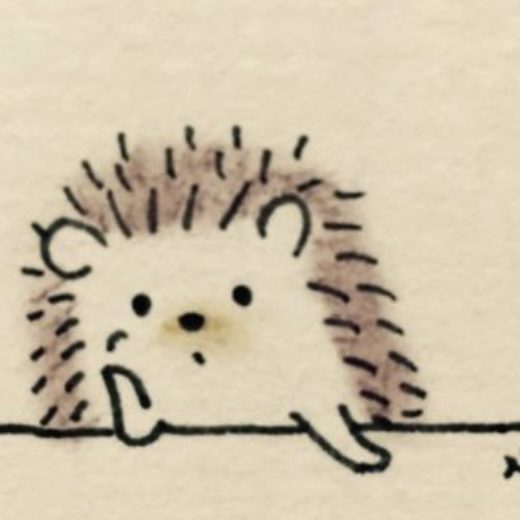
Hi Jose,
I fixed this using Param Dummy and set QAction.
thanks
It looks like this question has already been answered in the comments. If this is indeed the case, could you select this answer to indicate that the question can be closed?
Hi Jose,
I noticed you're trying to share some images, but unfortunately, I'm not able to see them on my end. Could you please try uploading them again?
Thanks
Kind regards,