Hello Dojo Community !
I have been working on an automation script with additional logic to clear out week old Correlated warning alarms that have their 'auto-clear' functionality disabled. The original script was kindly provided by Joachim from Dojo community :
SLC-AS-ClearNoticeAlarmsOlderThanAWeek/Clear Notice Alarms Older Than A Week_1/Clear Notice Alarms Older Than A Week_1.cs at master · SkylineCommunications/SLC-AS-ClearNoticeAlarmsOlderThanAWeek (github.com)
previous question : Method of Clearing Alarms from the alarm console using an Automation script - DataMiner Dojo
I’ve managed to select and filter the necessary alarm properties (AlarmID, Element ID, Hosting ID, Source, Value) using the SLNet Client tool. However, after executing the provided script in Visual Studio and testing in Cube AS validation, I encountered errors.
It appears that one of the arguments I’m passing isn’t correct. I receive an error stating the constructor takes 4 arguments in the format (int, int, int, string)
. Given that our DM version is 10.3.0.0-13184, I suspect that some .NET methods might behave differently. Also the Alarm is not getting cleared as expected (manually we do this by right click on the alarm and selecting the option 'clear alarm')
Could anyone kindly help me identify which argument might be incorrect and suggest how to adapt the script for my environment? I’d appreciate any guidance on fixing the issue so that the script clears the alarms as expected.
Thank you for your continued support!
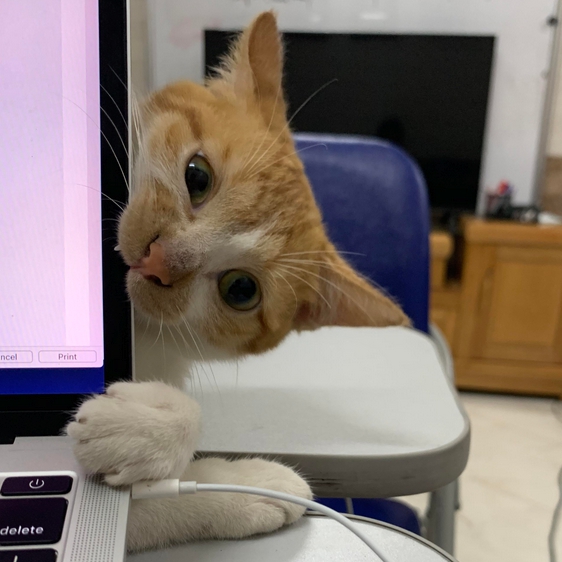
namespace ClearAllActiveAlarms
{
using System;
using System.Linq;
using System.Threading.Tasks;
using Skyline.DataMiner.Automation;
using Skyline.DataMiner.Net.Messages;public class Script
{
public void Run(IEngine engine)
{
try
{
engine.GenerateInformation("Script starting.");
ClearAllAlarms(engine);
engine.GenerateInformation("Script ending.");
}
catch (Exception e)
{
engine.ExitFail("Run|Something went wrong: " + e);
}
}private void ClearAllAlarms(IEngine engine)
{
try
{
engine.GenerateInformation("Fetching all active alarms to clear.");
var messages = GetActiveAlarms(engine);if (messages == null || messages.Length == 0)
{
engine.GenerateInformation("No active alarms were retrieved.");
return;
}engine.GenerateInformation($"Received {messages.Length} messages from the DataMiner system.");
ProcessActiveAlarms(messages, engine);
}
catch (Exception ex)
{
engine.GenerateInformation($"ClearAllAlarms|Something went wrong: {ex.Message}\n{ex.StackTrace}");
engine.ExitFail("ClearAllAlarms|Something went wrong.");
}
}private DMSMessage[] GetActiveAlarms(IEngine engine)
{
try
{
var getActiveAlarmsMessage = new GetActiveAlarmsMessage();
return engine.SendSLNetMessage(getActiveAlarmsMessage);
}
catch (Exception ex)
{
engine.GenerateInformation($"Failed to retrieve active alarms: {ex.Message}");
return null;
}
}private void ProcessActiveAlarms(DMSMessage[] messages, IEngine engine)
{
var oneWeekAgo = DateTime.UtcNow.AddDays(-7);foreach (var message in messages)
{
if (message is ActiveAlarmsResponseMessage alarmMessage)
{
Parallel.ForEach(alarmMessage.ActiveAlarms, alarm =>
{
if ((alarm.Severity == "Notice" || alarm.Severity == "Warning") && alarm.RootCreationTime > oneWeekAgo)
{
ClearSingleAlarm(alarm, engine);
}
else
{
engine.GenerateInformation($"Skipping Alarm {alarm.RootAlarmID} with Severity '{alarm.Severity}' or CreationTime '{alarm.RootCreationTime}' not matching criteria.");
}
});
}
else
{
engine.GenerateInformation("Received a message that is not an ActiveAlarmsResponseMessage. Skipping...");
}
}
}private void ClearSingleAlarm(AlarmEventMessage alarm, IEngine engine) // Updated type
{
try
{
engine.GenerateInformation($"Clearing Alarm {alarm.RootAlarmID} with Severity '{alarm.Severity}' and Source '{alarm.Source}'.");var clearingMessage = $"Cleared the alarm with DataMinerID '{alarm.DataMinerID}' and RootAlarmID '{alarm.RootAlarmID}' from Automation.";
var setAlarmStateMessage = new SetAlarmStateMessage(
alarm.DataMinerID,
alarm.RootAlarmID,
7,
clearingMessage
);
engine.SendSLNetMessage(setAlarmStateMessage);
engine.GenerateInformation($"Alarm with RootAlarmID '{alarm.RootAlarmID}' cleared.");
}
catch (Exception ex)
{
engine.GenerateInformation($"Failed to clear Alarm {alarm.RootAlarmID}: {ex.Message}\n{ex.StackTrace}");
}
}
}
}
Hi, you get the error for alarms that are in warning state correct?
Those alarms cannot be cleared, only alarms that are in Normal state can be cleared, should be the same in the alarm console, the option Clear Alarm… should only be displayed when the alarm return to Normal state.
Can you please check if this is the case? Otherwise could you show screenshot of the alarm console with the alarm that you want to clear and the information events generated by the script?
Hi I am clearing out warning and notice alarms that are generated by correlation rule which are clearable, so the script checks for the last seven days if there are these severity of alarms and then sends the command to SLNET to clear / change to normal. The alarms that cannot be cleared are not cleared ( original intention) this runs on a scheduled basis daily on our system to tidy up the alarm console.
Could @Tom Waterbley or anybody tell me where can I find the value "7" for the state "Clear" and also for the other State values listed please.
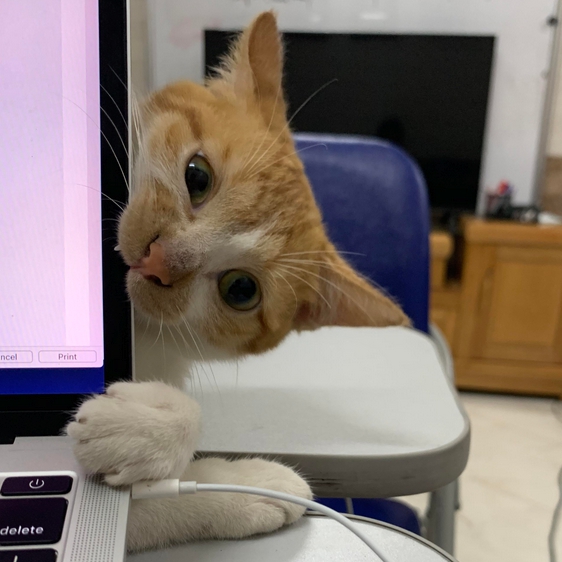
@Mohan, could you create a separate question for this? As this is a comment on an older question, it is likely that it will no longer get noticed by our content experts.
As this question has now been inactive for a long time, I will close it. If you still need assistance with this, could you contact tech support? For more info, see https://docs.dataminer.services/user-guide/Troubleshooting/Contacting_tech_support.html