Hi Dojo,
I’m trying to select all reservations in an automation script as indicated in the image marked in green containing a specific resource. This is the algorithm that I’m using but I’m not getting any results. We are unsure about the formatting, could anyone help us out? (We already tried using a NOT(expression) but this gives compile errors)
var filter = ReservationInstanceExposers.ResourceIDsInReservationInstance
.Contains(neededResource.ID)
.AND(ReservationInstanceExposers.Status.NotEqual((int)ReservationStatus.Canceled))
.AND(ReservationInstanceExposers.Status.NotEqual((int)ReservationStatus.Ended))
.AND(ReservationInstanceExposers.Start.GreaterThanOrEqual(start).OR(ReservationInstanceExposers.End.GreaterThanOrEqual(start)))
.AND(ReservationInstanceExposers.Start.LessThanOrEqual(stop).OR(ReservationInstanceExposers.End.LessThanOrEqual(stop)));var instances = SrmManagers.ResourceManager.GetReservationInstances(filter);
I’m guessing from your image you want to retrieve all bookings that have overlap with a certain time range. For that, I believe you can simplify your query to use 2 conditions:
ReservationInstanceExposers.Start.LessThanOrEqual(stop).AND(ReservationInstanceExposers.End.GreaterThanOrEqual(start))
A more detailed answer on StackOverflow.
The rest of your query looks good though.
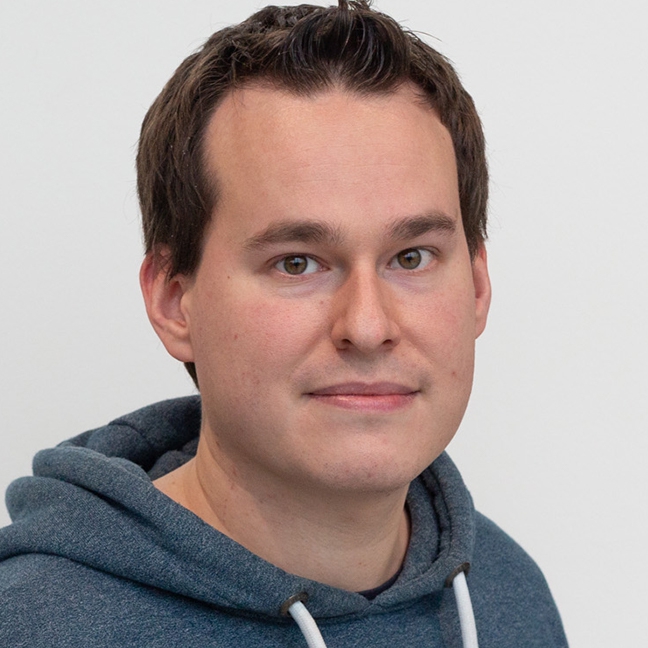
Hi Timothy. In addition to Sebastiaan’s answer, here’s a function which should return the names of the planned reservations that have overlap with a defined time range.
/// <summary>
/// Gets the names of all planned reservations that have overlap with the time range defined by the begin and end arguments. In case no reservations are planned during the time range, an empty array will be returned.
/// </summary>
/// <param name=”begin”>The begin of the time range.</param>
/// <param name=”end”>The end of the time range.</param>
/// <returns>A string array that contains the names of the planned reservations.</returns>
public static string[] GetPlannedReservationNames(DateTime begin, DateTime end)
{
DateTime start = begin.ToUniversalTime();
DateTime stop = end.ToUniversalTime();FilterElement<ReservationInstance> filter = ReservationInstanceExposers.Start.LessThanOrEqual(stop).AND(ReservationInstanceExposers.End.GreaterThanOrEqual(start));
ReservationInstance[] reservations = SrmManagers.ResourceManager.GetReservationInstances(filter);
return reservations.Select(reservation => reservation.Name).ToArray();
}
Kind regards
Thanks Sebastiaan, This works but only if I convert my datetimes to ToUniversalTime