Hi I am trying to do _dms.SendMessages in loop to the value of parameters of elements it takes lot of time and goes into timeout, what is the workaround for this?
Scenario:
I am iterating over services in a view, then on each element of that followed by PIDs for which I want value and do _dms.SendMEssages call, but it takes lot of time.
If I try to do in one go, then later it becomes difficult to identify based on primary key.
Can anyone please suggest the best way or approach
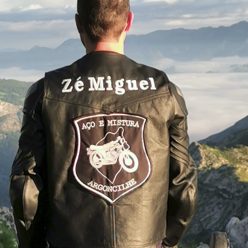
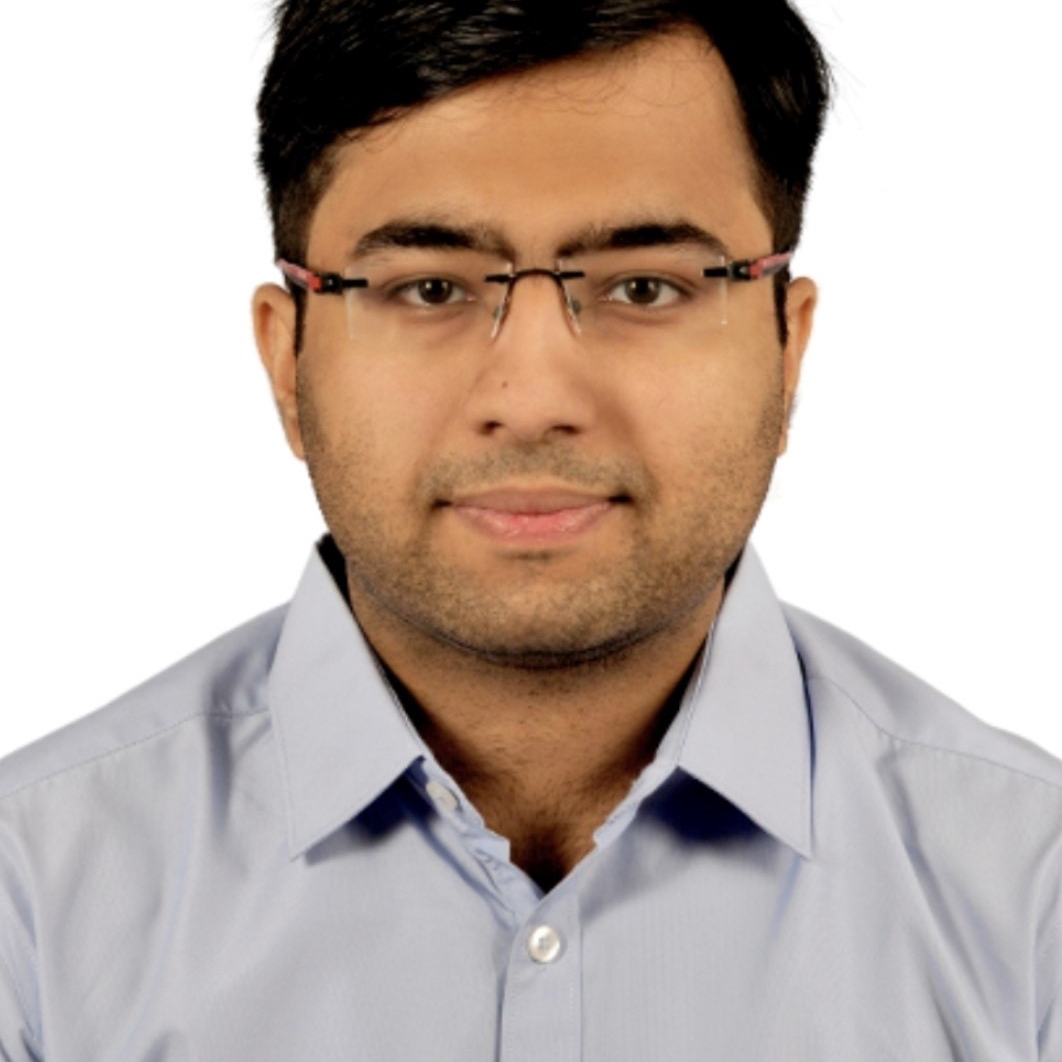
try
{
foreach (var service in serviceList)
{
var childInfos = service.Children?.ToList();
if (childInfos.Count > 0)
{
var reqParam = new List<DMSMessage>();
foreach (var childInfo in childInfos)
{
var paramsNeeded = childInfo.Parameters
.Where(x => _resources.Any(y => y.ParamterId == x.ParameterID))
.ToList();
foreach (var parameter in paramsNeeded)
{
var msg = new GetParameterMessage(childInfo.DataMinerID, childInfo.ElementID,
parameter.ParameterID, parameter.FilterValue, true);
reqParam.Add(msg);
}
}
try
{
var responseMsg = _dms.SendMessages(reqParam.ToArray());
var response = responseMsg.OfType<GetParameterResponseMessage>().ToList();
paramInfo = response.FindAll(x => !string.IsNullOrEmpty(x.Value.ToString()) && !x.Value.ToString().Equals("EMPTY", StringComparison.CurrentCultureIgnoreCase)).ToList();
}
catch (Exception e)
{
_logger.Error($"Error fetching parameters: {e.Message}");
continue;
}
if (paramInfo.Any())
{
foreach (var childInfo in childInfos)
{
var cells = InitializeTableCells();
CreateElementRow(ref rows, childInfo, cells, paramInfo, service.Name);
}
}
}
}
return new GQIPage(rows.ToArray()) { HasNextPage = false };
}
catch (Exception e)
{
_logger.Error($"Error fetching elements: {e.Message}");
return new GQIPage(rows.ToArray()) { HasNextPage = false };
}
Hi Apurva,
You might try sending all requests in one go and then processing the responses while mapping them to their respective service names. Here's a structured approach:
1. Create a list of all DMS messages you want to send.
2. Use a dictionary to map (DataMinerID, ElementID, ParameterID)
to the corresponding service name:
var requestMap = new Dictionary<(int DataMinerID, int ElementID, int ParameterID), string>();
3. Iterate over your service list and populate both the request list and the mapping dictionary:
requestMap[(childInfo.DataMinerID, childInfo.ElementID, parameter.ParameterID)] = service.Name;
4. Send all requests at once and process the responses efficiently. When iterating over the responses, reconstruct the key and retrieve the service name:
var key = (response.DataMinerID, response.ElementID, response.ParameterID);
if (requestMap.TryGetValue(key, out var serviceName))
{
// Process response for this service
}
5. (Optional) If you need to iterate responses per service, you can group them by serviceName
for easier processing.
This method should significantly improve performance by reducing the number of individual _dms.SendMessages
calls
Just an idea - hope it helps!
Kind regards
Hi Apurva,
I’m not sure I fully understood your question. Could you please provide more details about your scenario and share the code you’re trying to execute? This will help us better understand your issue and assist you more effectively.
Thanks in advance,
Kind regards