Hi, I’m looking for a way to create a scheduled task in an automation script. The NuGet package apparently cannot be found in Visual Studio. Is this outdated?
“Skyline.DataMiner.Core.Scheduler.Automation”
Thank you!
Using the IDmsScheduler from the Skyline.DataMiner.Core.DataMinerSystem.Common nuget, you can create and schedule your tasks in the Task Scheduler.
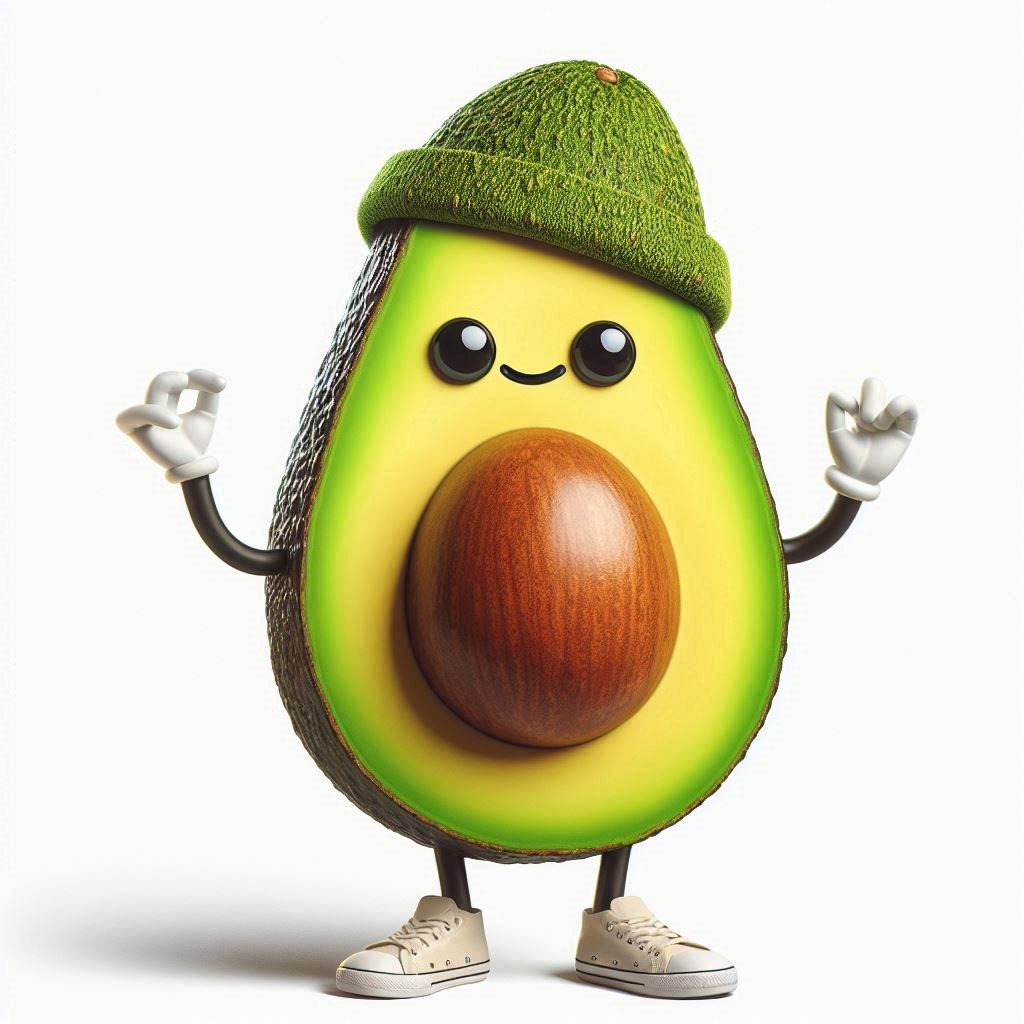
The above example works, but using the API with specific domain classes looks way better:
https://docs.dataminer.services/develop/devguide/ClassLibrary/ClassLibraryScheduler.html#defining-the-task-recurrence
But I can not find any of the classes mentioned above. I tried to use
var scheduleOnce = new ScheduleOnce(createSchedulerEvent: true);
but the class ScheduleOnce does not exists even when I added all necessary NuGet packages. And as @David Rid already described, the NuGet package for the Scheduler is not available with
Install-Package Skyline.DataMiner.Core.Scheduler.Automation
Which NuGet package do I need to install?
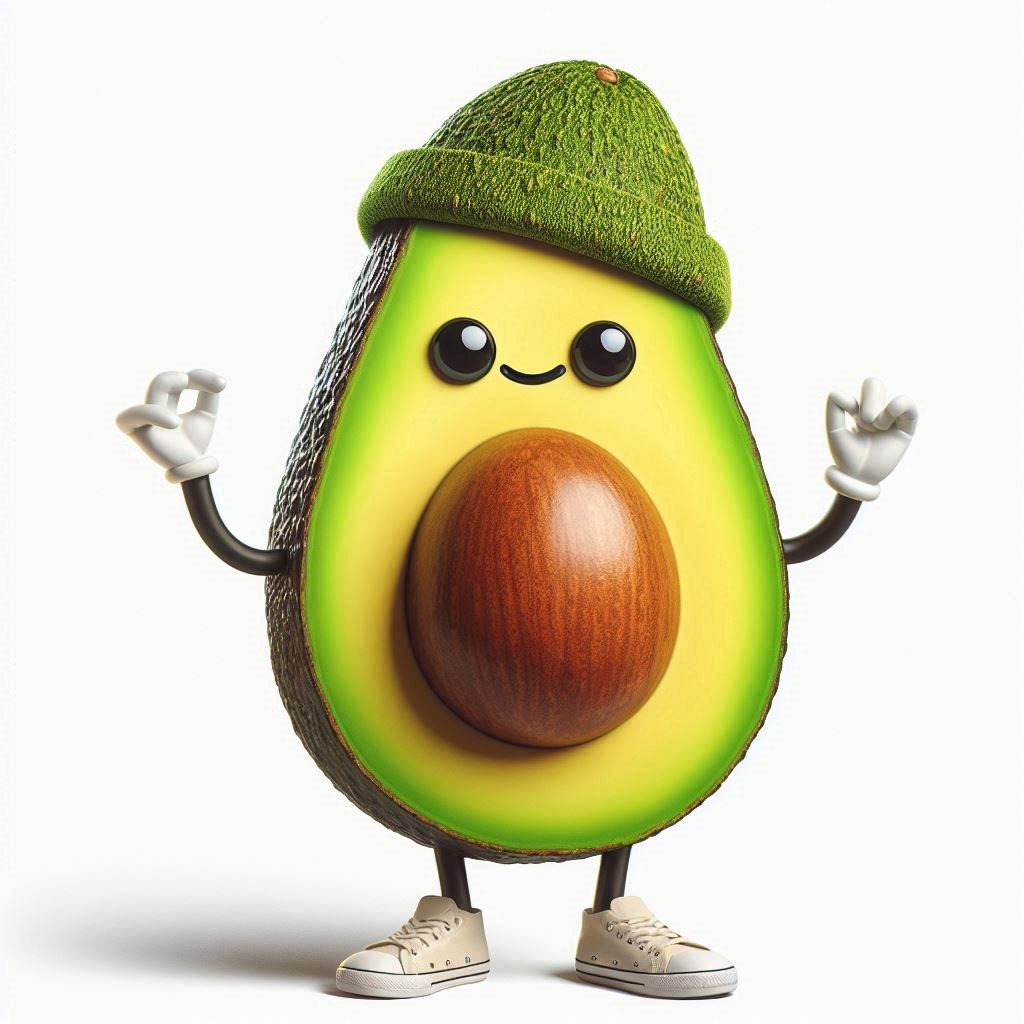
I went ahead with the solution I mentioned earlier, and it's still running without any issues so far. I used it as a base, not only for creating tasks but also to create events in the scheduler. If you're looking to clean up the code, consider creating your own scheduling function or method that accepts arguments, offering more flexibility.
At the moment, I haven't come across any other solutions. While it's not the most elegant approach, do you think it would work for your use case?
Yes that works, I already using it. But I would like to use the scheduler task classes like in the docs described. Using object arrays is not a good solution and if they provide a API for that I want to use it.
I will create a new question to address this issue.
Could anybody tell how can we use “paramLinked” parameter in the above script?
how can we pass value for paramLinked and how to retrieve that value in another automation script ? Is there any example please.
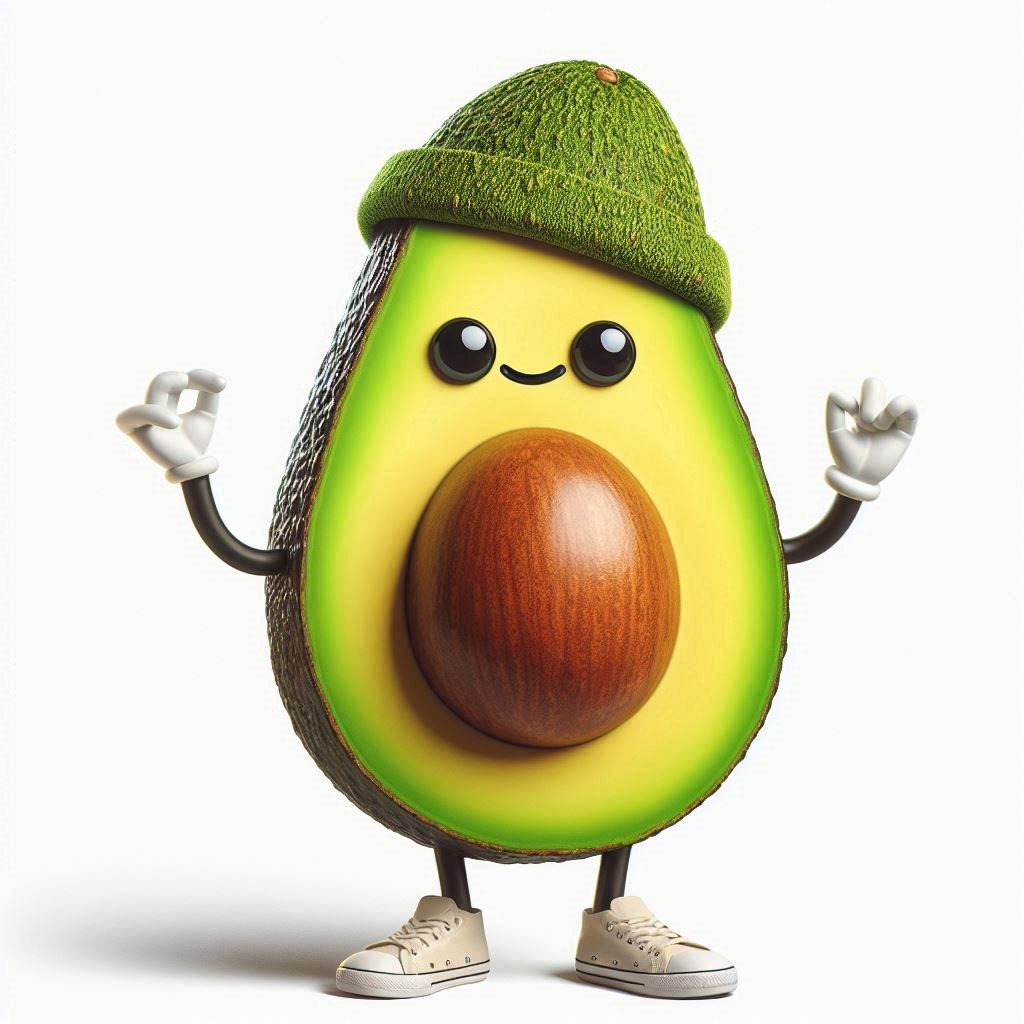
This is what needs to be typed into the linked parameter:
Specify the parameter number and whatever string value that should be assigned to it. ("START", "STOP" or the value of a nother param: $"{channel}")
If there are multiple values, just number them in the correct order.
_____________________________________________________________
string scriptName = "ExecuteBlackout";
string paramLinkedStart = "PARAMETER:1:START";
string paramLinkedStop = "PARAMETER:1:STOP";
string paramEventName = $"PARAMETER:2:{channel}";
string taskDescription = title;
_____________________________________________________________
new object[] // repeat actions
{
new string[]
{
"automation", // action type
scriptName, // name of automation script
paramLinkedStart,
paramEventName, // … other options & further linking can be added
"CHECKSETS:TRUE",
"DEFER:False", // run sync
},
},
new object[] {}, // final actions
};
Thank you! The documentation helped me. With minor modifications, I was able to make it run in an automation script.
private void RunSafe(IEngine engine)
{
var dms = engine.GetDms();
var dma = dms.GetAgent(Engine.SLNetRaw.ServerDetails.AgentID);
var scheduler = dma.Scheduler;
DateTime startDate = DateTime.Now.AddMinutes(1);
DateTime endDate = DateTime.Now.AddMinutes(2);
string activStartDay = startDate.ToString("yyyy-MM-dd");
string activStopDay = endDate.ToString("yyyy-MM-dd");
string startTime = startDate.ToString("HH:mm:ss");
string endTime = endDate.ToString("HH:mm:ss");
string taskType = "once"; // Task type (1=once , 2=weekly, 3=monthly, 4=daily)
string runInterval = "1"; // Run interval (x minutes(daily) / 1,…,31,101,102(monthly) / 1,3,5,7 (1=Monday, 7=Sunday)(weekly))
string scriptName = "scriptName";
string elemLinked = ""; //"PROTOCOL:1:123:456", // example of linking element 123/456 to script dummy 1
string paramLinked = "";
string taskName = "SchedulerCreateTaskTest";
string taskDescription = "Task description";
object[] schedulerTaskData = new object[] {
new object[] {
new string[] // general info
{
taskName, // [0] : name
activStartDay, // [1] : start date (YYYY-MM-DD) (leave empty to have start time == current time)
activStopDay, // [2] : end date (YYYY-MM-DD) (can be left empty)
startTime, // [3] : start run time (HH:MM)
taskType, // [4] : task type (daily / monthly / weekly / once)
runInterval, // [5] : run interval (x minutes / 1,…,31,101,102 / 1,3,5,7 (1=Monday, 7=Sunday)) (101-112 -> months)
"", // [6] : # of repeats before final actions are executed
taskDescription, // [7] : description
"TRUE", // [8] : enabled (TRUE/FALSE)
endTime, // [9] : end run time (HH:MM) (only affects daily tasks)
"", // [10]: minutes interval for weekly/monthly tasks. Either an end date or a repeat count should be specified
}
},
new object[] // repeat actions
{
new string[] {
"automation", // action type
scriptName, // name of automation script
elemLinked, // example of linking element 123/456 to script dummy 1
paramLinked, // … other options & further linking of dummies to elements can be added
// elem2Linked,
"CHECKSETS:FALSE",
"DEFER:False", // run sync
}
},
new object[] {} // final actions
};
scheduler.CreateTask(schedulerTaskData);
}