I was wondering if you'd be able to give me some guidance on whether what I want to achieve is possible in automation without having to write any C# code...
I want to make two scripts;
- One which is given a neuron element name, and iterates through every row in a given table, and changes a parameter in that table to 'Enabled' (which resets a counter).
- Another script which gets every element in a given 'View', and feeds them to the above script, so that I can run the above script on all neurons in a given view. P.S. I want to make two separate scripts so that I can either run this on a single neuron, or all of them.
Thanks, James.
Hi James,
You will have to use C# for this, but luckily there are already plenty of things to support you with to make it as easy as possible! 🚤
I would suggest you create a new automation script by using Visual Studio and our DIS plugin.
You can easily create a new project solution of type "DataMiner Automation"
If you need some extra help on this? Please take a look at our Automation training course. In specific following topic: Creating automation scripts using DataMiner Integration Studio (DIS) - DataMiner Dojo
To help you out for your specific use-case: (script 1) set values on rows of a table:
Simply adding the "neuron element" placeholder as an input under "protocols" on the script xml inside the solution. (example below)
In this solution there will already be a C# script block by default. Inside the "RunSafe()" method you can start adding your lines of code to represent your workflow in a simple manner.
I created a quick sample for what you try to achieve below.
Note that all "parameter IDs" and "newValue" are fictional of course.
var neuronElement = engine.GetDummy("Neuron Element");
var primaryKeys = neuronElement.GetTablePrimaryKeys(1000/*table ID of the given table*/);object newValue = 1 /*Enabled*/;
foreach (var pk in primaryKeys)
{
neuronElement.SetParameterByPrimaryKey(1210 /*write parameter ID of table column to set*/, pk, newValue);
}
Regarding the second script, you can easily find elements from a certain view by using "engine.FindElementsInView()"
Below example shows how you could gather elements from a specific view, iterate them, and for the elements that match the expected protocolname, run the subscript.
var elements = engine.FindElementsInView("Neuron overview");
foreach (var element in elements)
{
if (!element.ProtocolName.Equals("NEURON PROTOCOLNAME"))
{
continue;
}// prepare a subscript
SubScriptOptions subscript = engine.PrepareSubScript("Neuron Reset Counters");// link the main script dummies to the subscript
subscript.SelectDummy("Neuron Element", element);// set some more options
subscript.Synchronous = false;
subscript.LockElements = true;// launch the script
subscript.StartScript();
}
note that if you plan on doing this frequently, still consider combining both scripts into one. Depending on the number of expected subscript runs.
The examples I made above are all using methods that are directly available on top of the IEngine object. For more advanced interactions with DataMiner objects from within automation module, We would recommend you to approach this via the iDMS class library features that are available via following nuget: NuGet Gallery | Skyline.DataMiner.Core.DataMinerSystem.Automation 1.1.1.11
Once the nuget has been added to your solution, you can start to consume it by following this docs pages: Class library introduction | DataMiner Docs
Hope this helped you out! let us know if you need anything more!
Have fun! 🙌
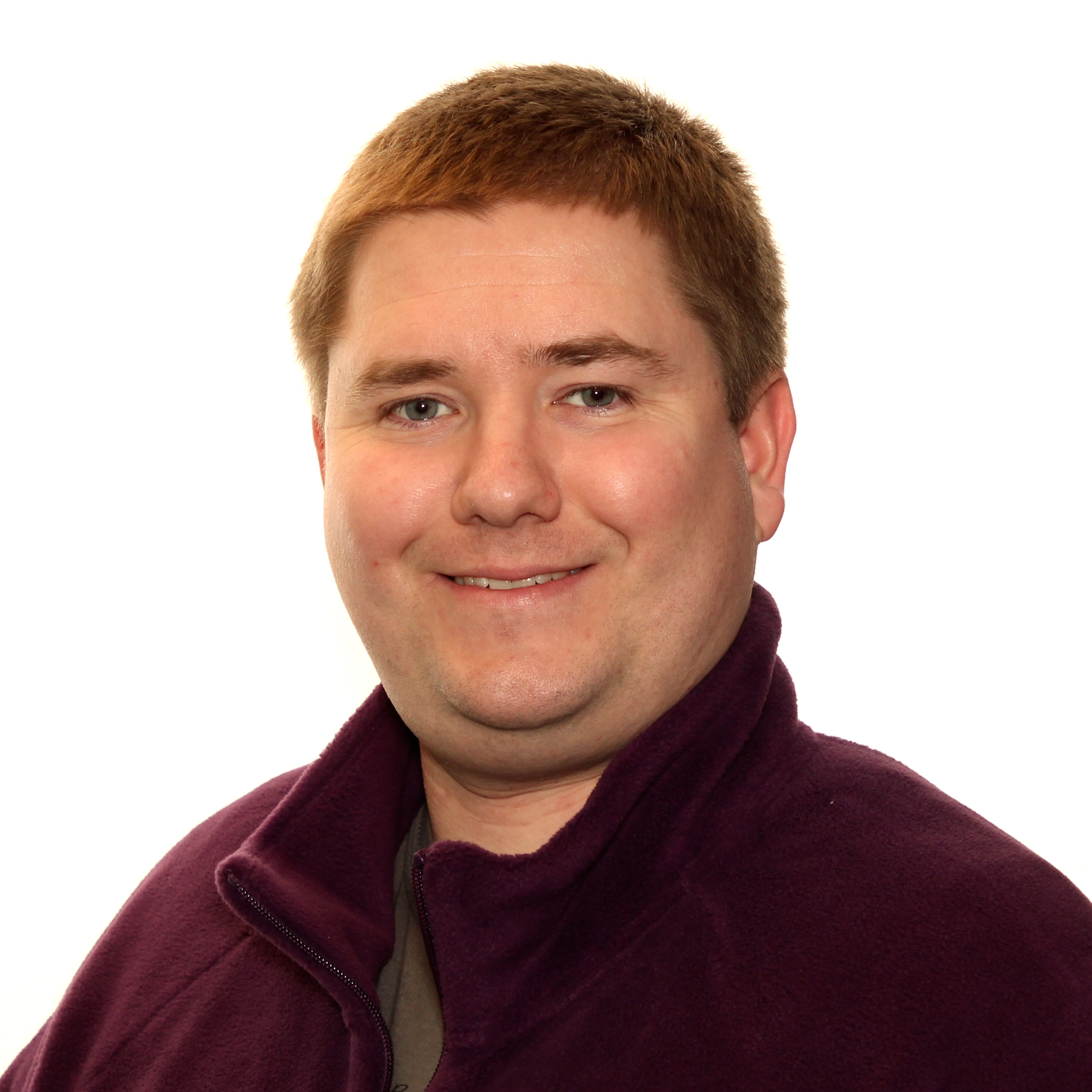
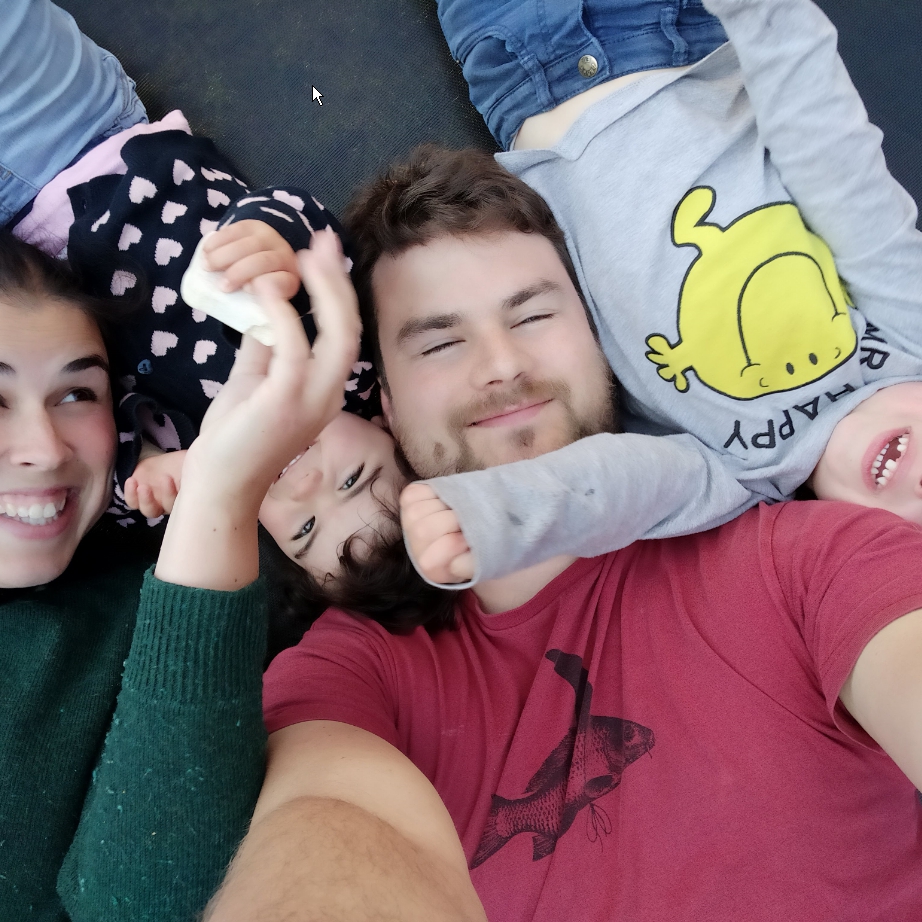
Hi James,
Good to hear! Have fun with Visual Studio and the world of coding!
I’m sure this will open new DataMiner opportunities and challenges for you to tackle. Reach out if you need any help!
Hi James - definitely working with two scripts is the way forward. I would even recommend that if you would not be planning on using the scripts individually or combined, just for modularity and to make it easier to maintain. But you can definitely run an automation to iterate on all elements in a view and then launch a subscript on each of those (changing that parameter to enabled).
I'm not that versed at creating automation scripts, but I doubt that both would be achievable without C#. But not 100% sure, so let's see if some more experienced people can confirm.
Maybe quick question in that respect, is the number of items in the table fixed (e.g. related to a fixed / pre-defined number of input ports) or can it be variable from one Neuron to another?
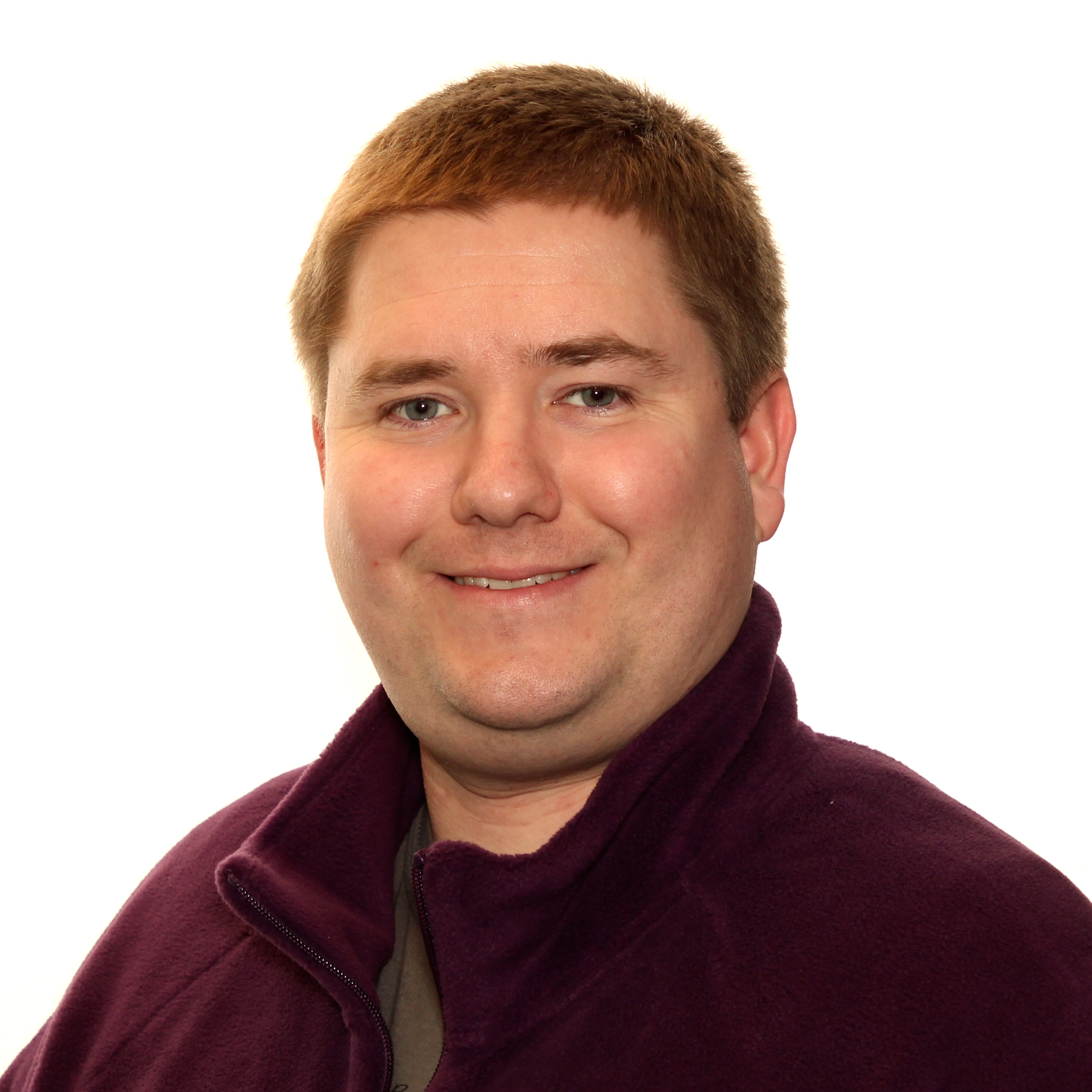
Hello Ben.
Thanks for your answer, as for your question; it can vary between 32 and 64 rows, but this is deterministic from the FW the neuron is running. That said it would probably be more reliable to just get the count of rows and doing a for loop, but I see no way to do that without having to write C#, so I’m pretty sure the answer is you can only iterate through a table with C# but I’m hopeful that’s not the case, but if it is I’ll give it a go.
Thanks Thijs.
I was mostly trying to avoid C# as I don’t have a Visual Studio license, but if that’s the only solution to my requirement then I’ll look to get one, thanks!
James.