Hello Dojo,
I am working on a code that has some hardcoded information that is sensible and would like to give an extra level of security to. Right now this information is formed by two strings that are being passed hardcoded.
The idea is to try to get some kind of UI that allows the user to include these parameters, to write those parameters to an encrypted file and to pass this to the AdHocDataSource, which should be able to decrypt that file and extract these strings. Is this possible?
If this is not possible, I am wondering if there is any other smarter way to find a solution to this use case and give that extra layer of security.
Thank in advance
Hi Jon,
What I was thinking that could be an idea to achieve this:
- Create a very tiny protocol with a parameter with the "save"-attribute and that is writeable and has measurement type string with option "password". The user is then able to set this password safely in Cube already.
- To elaborate this, you could write an Interactive Automation Script, that you can embed in an elegant way in your Low Code App, where you can let the user type in a password, by using the "PasswordBox" . This could then in the background let the password be saved in that element.
- In your Ad Hoc Data Source, you can only store "encrypted strings" hardcoded, and use that password in the element parameter to actually decrypt it and show it. In this way, when a user for example checks the Ad Hoc Data Source in the Automation module, he will only see the encrypted string. Also if you were to push your code to a github solution published on a repo, you would not risk that sensitive date is leaked.
- I think on the internet there are many examples on using a password, encrypt and decrypt strings, one of the more recent good examples I found was via this link : A straightforward way in C# .NET to encrypt and decrypt a string using AES | Asp.Net Core, Angular, Xamarin and Maui (wordpress.com) . Just for future reference, in case that the link could break maybe in the future, I will also copy-paste it below. Of course you can go one step further, and start to work with public and private keys, but that's only going to add value if you also want users to give the possibility to pass on sensitive data. Based on your information, I think you only want to let the users read sensitive date (if they have the password) and in that case one password is enough.
Maybe there are better ways, but I hope this maybe can help you already.
Kind regards,
Joachim
using
System;
using
System.IO;
using
System.Security.Cryptography;
namespace
MyNiceApp;
class
Program
{
static
void
Main(
string
[] args)
{
// The plaintext string
string
plaintext =
"Hello, Baha'is of the World!"
;
// The password used to encrypt the string
string
password =
"my-secret-ian-password"
;
// Encrypt the string
string
encrypted = EncryptString(plaintext, password);
// Decrypt the encrypted string
string
decrypted = DecryptString(encrypted, password);
// Print the original and decrypted strings
Console.WriteLine(
"Original: "
+ plaintext);
Console.WriteLine(
"Decrypted: "
+ decrypted);
}
static
string
EncryptString(
string
plaintext,
string
password)
{
// Convert the plaintext string to a byte array
byte
[] plaintextBytes = System.Text.Encoding.UTF8.GetBytes(plaintext);
// Derive a new password using the PBKDF2 algorithm and a random salt
Rfc2898DeriveBytes passwordBytes =
new
Rfc2898DeriveBytes(password, 20);
// Use the password to encrypt the plaintext
Aes encryptor = Aes.Create();
encryptor.Key = passwordBytes.GetBytes(32);
encryptor.IV = passwordBytes.GetBytes(16);
using
(MemoryStream ms =
new
MemoryStream())
{
using
(CryptoStream cs =
new
CryptoStream(ms, encryptor.CreateEncryptor(), CryptoStreamMode.Write))
{
cs.Write(plaintextBytes, 0, plaintextBytes.Length);
}
return
Convert.ToBase64String(ms.ToArray());
}
}
static
string
DecryptString(
string
encrypted,
string
password)
{
// Convert the encrypted string to a byte array
byte
[] encryptedBytes = Convert.FromBase64String(encrypted);
// Derive the password using the PBKDF2 algorithm
Rfc2898DeriveBytes passwordBytes =
new
Rfc2898DeriveBytes(password, 20);
// Use the password to decrypt the encrypted string
Aes encryptor = Aes.Create();
encryptor.Key = passwordBytes.GetBytes(32);
encryptor.IV = passwordBytes.GetBytes(16);
using
(MemoryStream ms =
new
MemoryStream())
{
using
(CryptoStream cs =
new
CryptoStream(ms, encryptor.CreateDecryptor(), CryptoStreamMode.Write))
{
cs.Write(encryptedBytes, 0, encryptedBytes.Length);
}
return
System.Text.Encoding.UTF8.GetString(ms.ToArray());
}
}
}
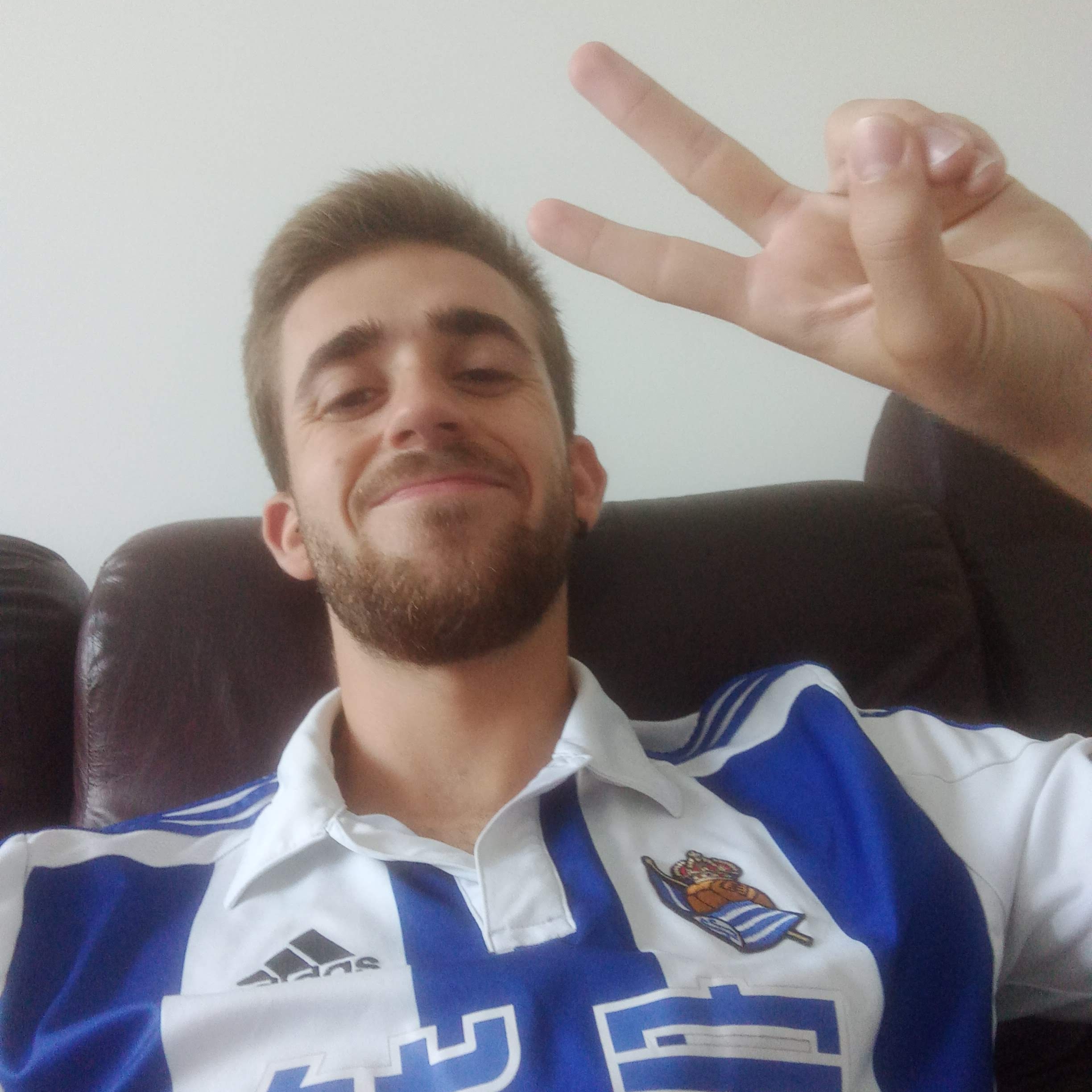
This is a great approach Joachim! Thanks for the help