Hi,
I’m extracting data with an ad-hoc data source from a DOM definition that consists of three section definitions.
Currently, I’m using the Read method to retrieve all the data. However, in this scenario, I’m solely concerned with the data into a particular section definition.
var records = GoldenBatchManager.DomHelper.DomInstances.Read(fullFilter);
Is there a way to exclusively retrieve information from just one specific section definition? I understand that a GetFieldValue operation could be performed for the various fields within that specific section definition.
Thanks
Leander,
Thanks for the call. as you mentioned the problem lays in the loading time of reading the DomInstances because your architecture has a DomDefinition where 1 of it’s section has multiple records you don’t need at that point of your flow. And you would like to read out the instance without the impact of this section.
I do not think it is possible today to exclude a section from the Read since you are asking to read the full Instance (and not just a specific section or sections)
There are a few things I can advise to improve the speed:
- Change the architecture of your DOM Module. I personally always make a new Dom Definition as soon as there is a 1-to-many relation. I like to view things as basic Database architecture and see a Dom Definition as a Table. So, when I have multiple rows that should link to 1 other row I will create 2 tables. => 2 Dom Definitions.
I believe this will make the most impact and provide you with more control what and how to do things. - Use a helper to process big data chunks:
Example: (Tools is part of Skyline.DataMiner.Net)
public static List<DomInstance> GetDomInstances(this DomHelper domHelper, List<Guid> instanceIds)
{
var instances = Tools.RetrieveBigOrFilter(
instanceIds,
id => DomInstanceExposers.Id.Equal(id),
filter => domHelper.DomInstances.Read(filter));return instances;
} - Use paging. This is useful when processing is needed based on the result. I think it is not applicable to your use case as you want to always show all the DomInstances (based on your filter) in the low code app table.
Example: (this will get all instances based on a field descriptor, kind of the select * where field = “hello” )public static List<DomInstance> GetAllDomInstanceByFieldValueByPages(this DomHelper domHelper, FieldDescriptor fieldDescriptor, string value)
{
var filter = DomInstanceExposers.FieldValues.Field(fieldDescriptor.ID.Id.ToString()).Equal(value);
var allDomInstances = new List<DomInstance>();
var pagingHelper = domHelper.DomInstances.PreparePaging(filter);
while (pagingHelper.MoveToNextPage())
{
var currentPage = pagingHelper.GetCurrentPage();
allDomInstances.AddRange(currentPage);
}return allDomInstances;
}
Don’t just copy this last example as it has no value it this form. It’s simply an example how to use it. Instead of adding it to a list, one should add logic to process the currentPage before moving to the next.
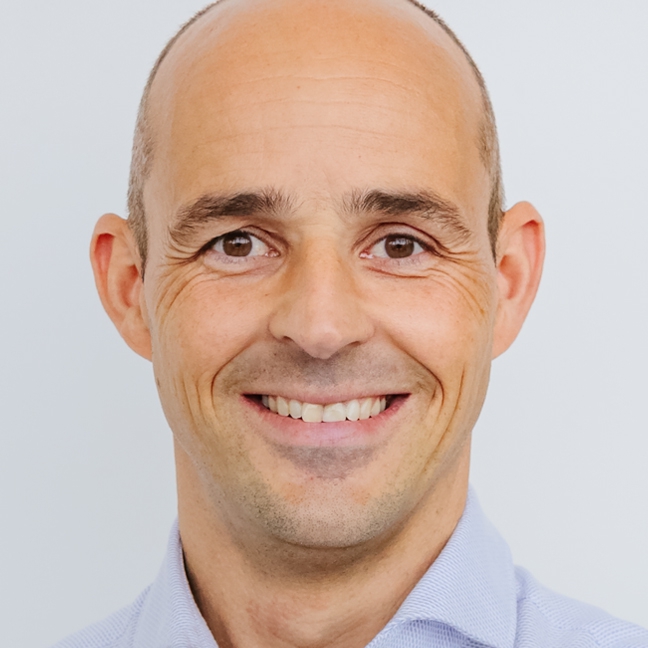
Hi Leander,
The current API (10.3.10) will always return the entire DOM instance including all sections. There’s a roadmap item coming up, to only return specific fields from the API, although the specifics of this plan are still under development.
Leander,
I have not tested this, but perhaps this filter does what you need:
var filter = DomInstanceExposers.SectionDefinitionIds.Contains(sectionIdGuid)
Please note that the same Sectiondefinition can be used by different DomDefinitions. So your result might provide Instances of different DomDefinitions. This is something you might need to take into account when parsing.
For completeness: DomInstanceExposers requires
using Skyline.DataMiner.Net.Apps.DataMinerObjectModel;
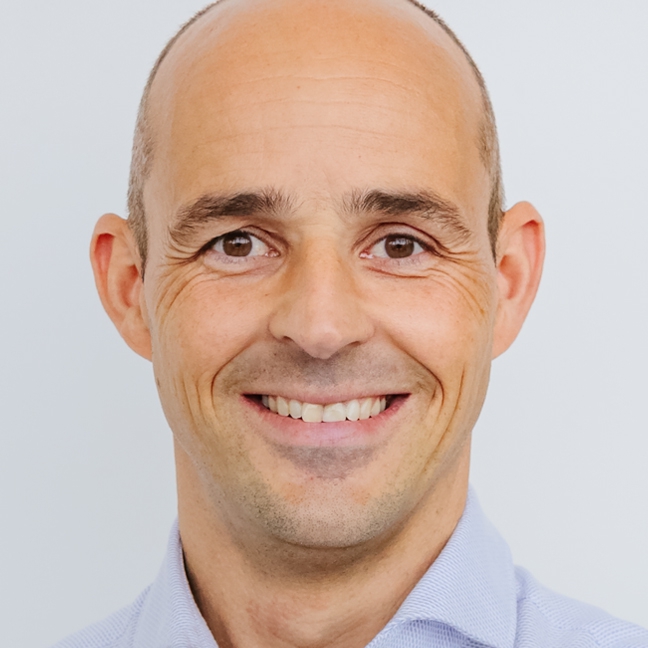
Thanks for your response, Mieke.
I’ve implemented the extra filter, but the outcome remains unchanged. It appears that the filter is designed to exclude records that entirely lack that section definition. However, if the section is present, the information retrieved from the record still includes all sections.
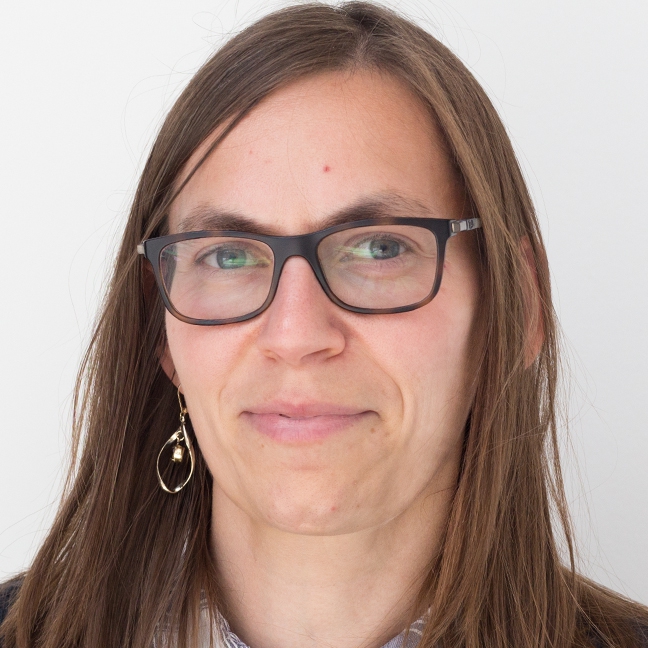
Leander,
Seems like you want a completely new method to ReadSection instead of reading Instances.
It is normal that domHelper.DomInstances.Read will return the full instance reference since you ask to read the Instance.
The filter will make sure that the result is limited to instances that contain the section definition in their Dom definition.
I personally don’t really see a problem in this as you can just get the fields from the section you want.
I do a similar thing in a datasource I created.
Hey Mieke, thanks for the excellent advice on how to efficiently load the data.
By reworking the database structure, I managed to improve speed with a factor of 10. In my specific case, it was important to understand that most of the data that was linked with a many to one relation would only be requested with a user click. By separating this specific data into a different DomDefinition, i.e. not only a different SectionDefinition as it used to be, the data can be much easier consulted.