Hi all,
I want to create an automation script that updates an element to use another protocol version. Is there any method that does this in the automation module?
Thanks,
Ryan
Hi Ryan,
to change the version of a protocol you can use the below piece of code in your Automation script.
Do note that this will only change the version and not configure any extra items that might be required with the (major) version change.
The use-case should only be to update an iteration of a specific range.
For example 1.0.0.x to 1.0.0.y or 1.1.0.x tot 1.1.0.y
public static class MyElementExtentions
{
public static void AssignProtocolVersion(this Element element, Engine engine, string versionToSet)
{
AddElementMessage msg = new AddElementMessage
{
DataMinerID = element.DmaId,
ElementName = element.ElementName,
ElementID = element.ElementId,
ProtocolVersion = versionToSet,
};engine.SendSLNetMessage(msg);
}
}
Example of usage:
public class Script
{
/// <summary>
/// The Script entry point.
/// </summary>
/// <param name="engine">Link with SLAutomation process.</param>
public void Run(Engine engine)
{
Element element = engine.FindElementsByName("MyTestElement").FirstOrDefault();
string versionToSet = "1.0.0.1";
if (element.ProtocolVersion == "1.0.0.1")
{
versionToSet = "1.0.0.2";
}element.AssignProtocolVersion(engine, versionToSet);
}
}
Disclaimer:
"Note that this is an internal call and we do not recommend using this, as it is not officially supported and we cannot guarantee that it will still work in the future. As a rule, you should avoid using SLNet calls, as these are subject to change without notice. We recommend to instead always use the correct UI or automation options provided in DataMiner Automation or through our web API."
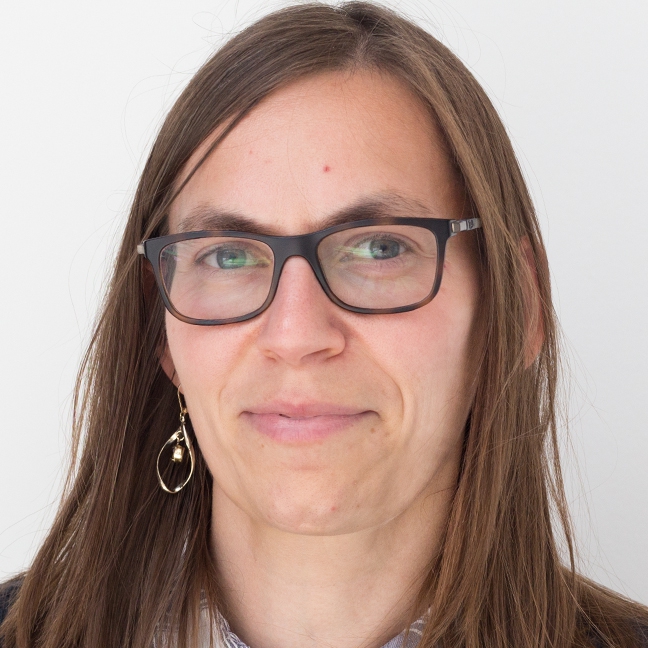
Hi Ryan,
When using “Production” instead of the version (for example “1.0.0.2”) the element will switch to the version that is defined as Production.
You do need to have a version set as production.
Could it be it is failing because none of the versions are set as Production?
I have tested it by using this on the code I shared previously :
Element element = engine.FindElementsByName(“MyTestElement”).FirstOrDefault();
string versionToSet = “1.0.0.1”;
if (element.ProtocolVersion == “1.0.0.1”)
{
versionToSet = “Production”;
}
element.AssignProtocolVersion(engine, versionToSet);
Thank you that works for me now!
Hi Mieke,
Thank you for the solution it works for normal protocol versions. When trying to upgrade to a production version it doesn’t find any of them, is there any particular format I need to do it for Production versions of protocols/ is there any other parameters I need to add for upgrades to Production versions.
Thanks,
Ryan