- getting data through Api call, now for edited and completed job status rows i need to show start in update job status column and for running job status stop
- on toggle i need to send a post api (start or stop ).so how will be the flow for that .
Hi Chirangee,
I’m not sure if I fully understood the question, but it seems clear that you'll need a QAction to manage what you want to achieve with the table.
1) What type of table is it? SNMP?
If it’s an SNMP table, you can set a trigger on the PID of the table, triggering a QAction to read the received values. Inside the QAction, you can read the value from the JobStatus column and check each row's job status. Based on the value, update the UpdateJobStatus column with the corresponding value (either "start" or "stop"). In the end, you can fill the array with the column's data.
E.g:
If the table isn’t SNMP-based, are you already using a QAction to populate it? If so, you just need to read the information from the JobStatus column, append the corresponding value to the new UpdateJobStatus column, and then fill the array with all the data.
2) If you want to trigger a column in a table to send a command, you should create a QAction with an "OnChange" event for the parameter of type write:
Using DIS:
You will also need to add this in the QAction:
This will allow you to know, within the QAction, which row was clicked.
After that, you can perform the post call for the desired Job.
Hope this helps you,
Kind regards,
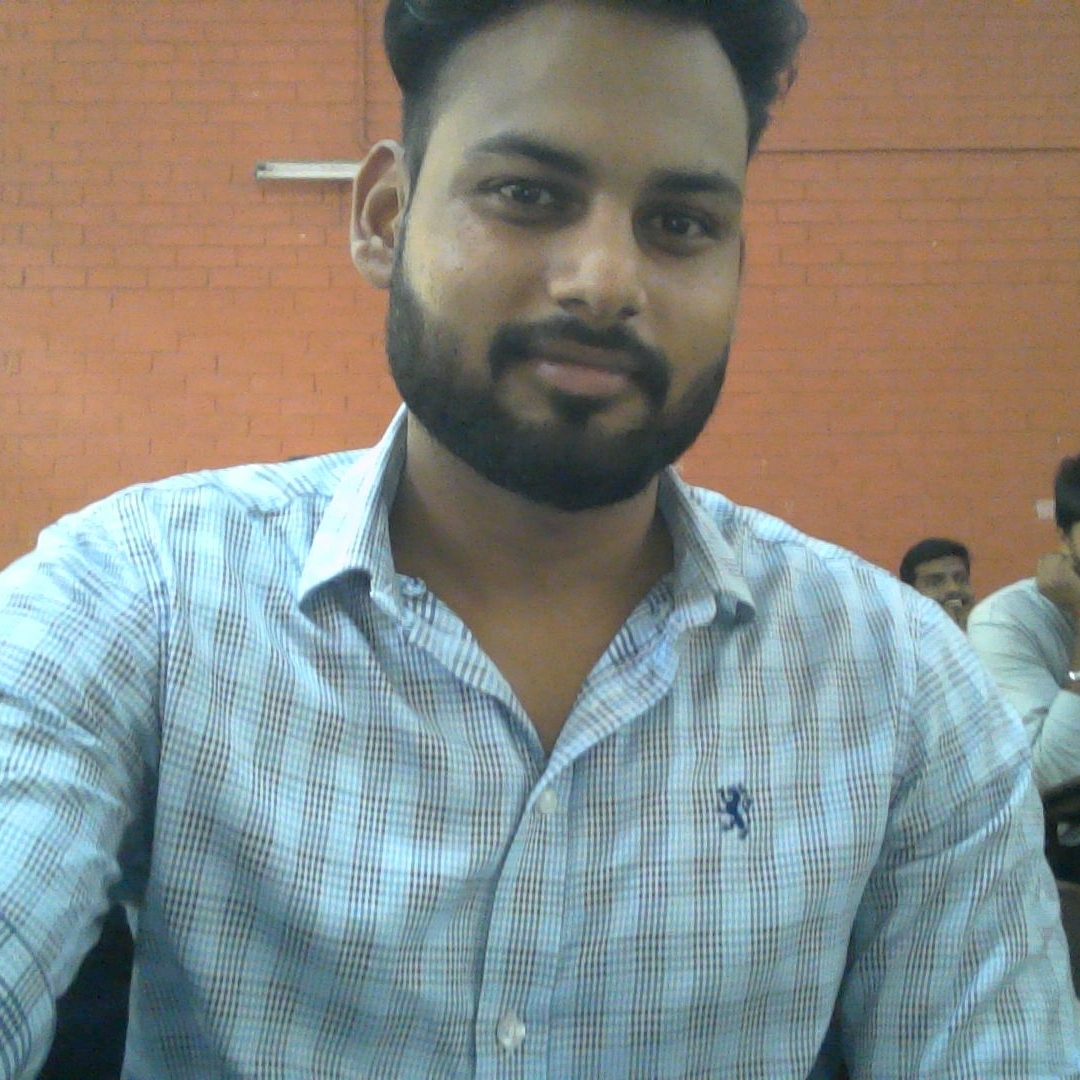
things are working well now i want a post api call on this toggle button click data will update job status column value {start , stop } .
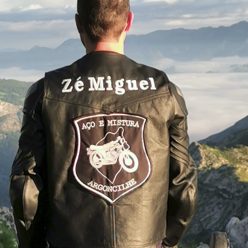
Hi Chirangee,
Did you try what I suggested in my second point?
In a nutshell, you can create a QAction for the write parameter with row=”true”, and inside the QAction, use protocol.RowKey() to retrieve the table’s key.
With this approach, you should be able to target the specific row and modify it as needed (e.g, get the full row, or just a column and set a row, or just a cell…)
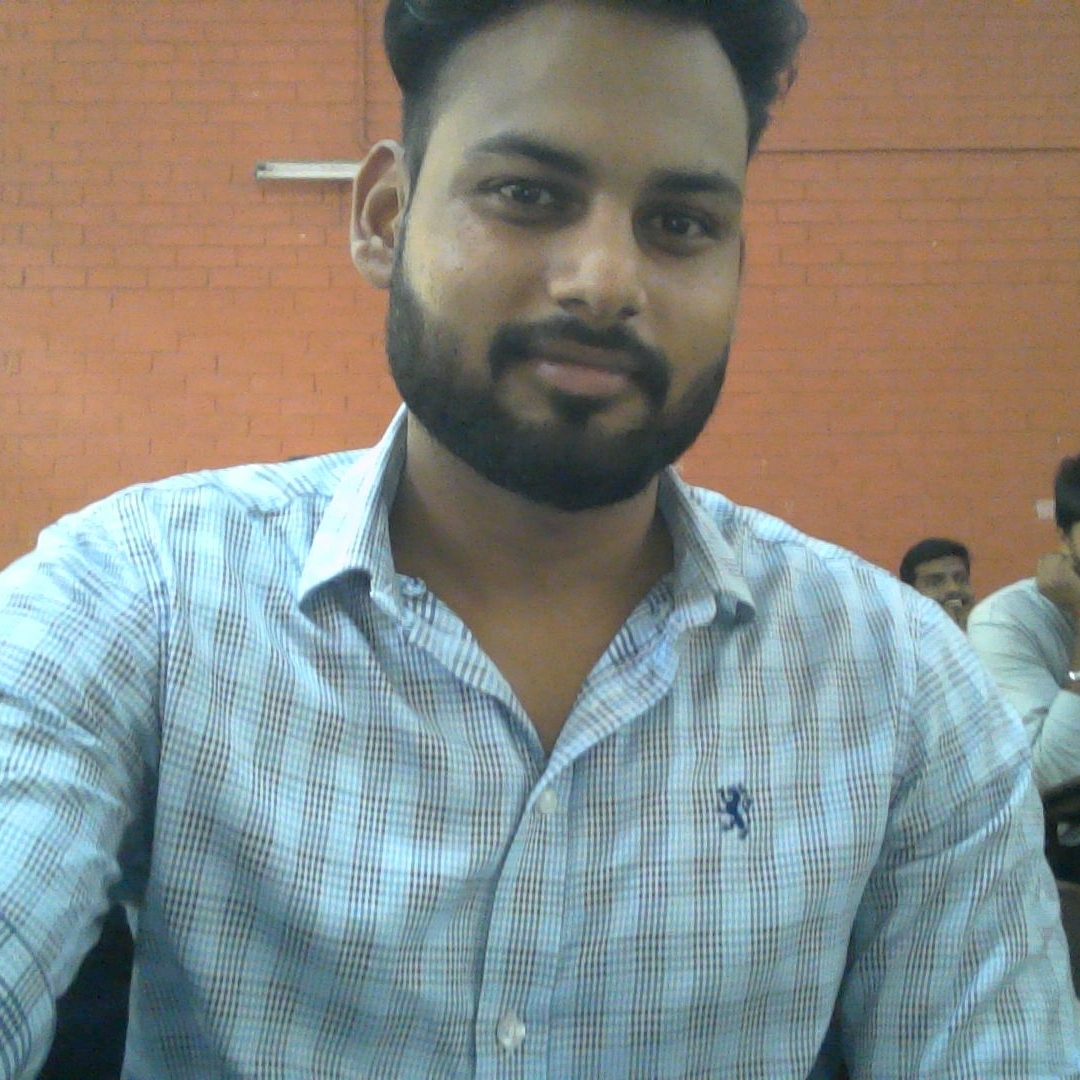
yes i jost got the flow now it works. Thanks
Hi Chirangee,
Overall, your C# code looks good, but there are a few issues in your XML file.
First, you should always define the PID of the read parameters in your columnOptions, unless the column is exclusively a write-only column.
Second, parameter 216 shouldn’t be of type button, especially since you want to display the current UpdateJobStatus as well.
Try the following:
hi jose silva,
thanks for reply see this code once i m getting respective data in log but not in table
using System;
using System.Collections.Generic;
using System.Diagnostics;
using System.Globalization;
using System.Text;
using Newtonsoft.Json;
using Skyline.DataMiner.Net.NetworkDiscovery;
using Skyline.DataMiner.Scripting;
public static class Parameter
{
public static class Jobs
{
public const int TablePid = 210; // Table ID for the Jobs table
}
}
/// <summary>
/// DataMiner QAction Class.
/// </summary>
public static class QAction
{
/// <summary>
/// The QAction entry point.
/// </summary>
/// <param name="protocol">Link with SLProtocol process.</param>
public static void Run(SLProtocol protocol)
{
try
{
// Get the JSON data as a string from a parameter (e.g., parameter ID 200)
string source = Convert.ToString(protocol.GetParameter(19));
// Deserialize the JSON content into C# objects
Rootobject rootObjects = JsonConvert.DeserializeObject<Rootobject>(source);
List<object[]> jobs = new List<object[]>();
// Iterate over each job in the JSON and map it to the table structure
foreach (Job job in rootObjects.Jobs)
{
string buttonAction = GetButtonForJobStatus(job.Job_Status);
protocol.Log(buttonAction + "data for button ");
jobs.Add(new JobsQActionRow
{
Jobname_201 = job.Job_Name,
Jobtype_202 = job.Job_Type,
Jobstatus_203 = job.Job_Status,
Updatedtime_204 = job.Updated_Time,
Nodename_205 = job.Node_Name,
Button_206 = buttonAction,
}.ToObjectArray());
}
// Insert the data into the DataMiner table (with table PID 301)
protocol.FillArray(Parameter.Jobs.TablePid, jobs, NotifyProtocol.SaveOption.Full);
}
catch (Exception ex)
{
protocol.Log("QA" + protocol.QActionID + "|Deserializing JSON text failed: " + ex.Message, LogType.Error, LogLevel.NoLogging);
}
}
private static string GetButtonForJobStatus(string jobStatus)
{
switch (jobStatus.ToLower())
{
case "running":
return "Stop";
case "edited":
case "completed":
return "Start";
default:
return "Unknown"; // Handle unexpected statuses
}
}
}
public class Rootobject
{
public Job[] Jobs { get; set; }
}
public class Job
{
public string Job_Name { get; set; }
public string Job_Type { get; set; }
public string Job_Status { get; set; }
public string Updated_Time { get; set; }
public string Node_Name { get; set; }
}
/// <summary>
/// Class to represent a row in the DataMiner table.
/// </summary>
public class JobsQActionRow
{
public string Jobname_201 { get; set; }// Column 201: Job Name
public string Jobtype_202 { get; set; }// Column 202: Job Type
public string Jobstatus_203 { get; set; }// Column 203: Job Status
public string Updatedtime_204 { get; set; }// Column 204: Updated Time
public string Nodename_205 { get; set; }// Column 205: Node Name
public string Button_206 { get; set; }
// Convert the properties to an object array for insertion into the DataMiner table
public object[] ToObjectArray()
{
return new object[]
{
Jobname_201, // Maps to Column 201
Jobtype_202, // Maps to Column 202
Jobstatus_203, // Maps to Column 203
Updatedtime_204, // Maps to Column 204
Nodename_205, // Maps to Column 205
Button_206,
};
}
}
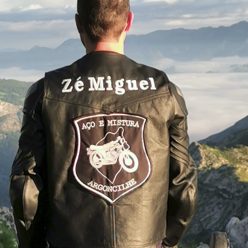
Hi Chirangee,
See my response below
……