Hello Dojo,
I am currently working on a project where I created a Low Code App that runs an Interactive Automation Script with the click of a button.
On the screen that appears, the user needs to fill in some parameters and then click the ‘Apply’ button. After clicking the button, the script executes and applies the required action.
However, the customer would like to see a pop-up window after clicking the ‘Apply’ button. I am unsure if this is possible. I am aware of a notification that appears after execution, which can indicate that the action has been taken, but this happens post-execution.
Ideally, after the user selects all the parameters and clicks ‘Apply’, a pop-up would appear confirming their selections and asking, “Are you sure you want to apply?” Only when confirming on ‘Yes’ the action will take place.
I hope someone can help with this.
Thank you in advance. 🙂
Hey Nejra, the simplest way to accomplish this is by using another IAS within the one you’re already using this. Make a simple “ConfirmDialog” with just a cancel/confirm button and display some text. I made a sample below:
public class ConfirmDialog : Dialog
{
public ConfirmDialog(IEngine engine, string message) : base(engine)
{
// Init widgets
Label = new Label(message);// Define layout
AddWidget(Label, 0, 0);
AddWidget(CancelButton, 1, 0);
AddWidget(ConfirmButton, 1, 1);
}public Button CancelButton { get; } = new Button(“Cancel”) { Width = 100 };
public Button ConfirmButton { get; } = new Button(“Confirm”) { Width = 100 };
private Label Label { get; set; }
public static bool ShowMessage(IEngine engine, string message)
{
bool confirmed = false;try
{
var dialog = new ConfirmDialog(engine, message);
dialog.CancelButton.Pressed += (sender, args) =>
{
confirmed = false;
engine.ExitSuccess(“Close”);
};dialog.ConfirmButton.Pressed += (sender, args) =>
{
confirmed = true;
engine.ExitSuccess(“Close”);
};var controller = new InteractiveController(engine);
controller.ShowDialog(dialog);return confirmed;
}
catch (ScriptAbortException)
{
return confirmed;
}
}
}
This could be called by doing ConfirmDialog.ShowMessage(engine, “Are you sure you want to perform the operation?”) which should then return the boolean of if it was confirmed or not.
Minor note: make sure you’re using the InteractiveAutomationScriptToolkit nuget library.
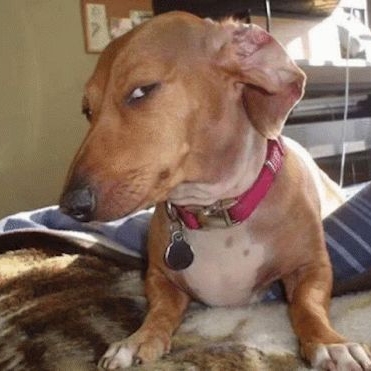
Hi Blake, thanks a lot for your help, I will use this solution!