We have created libraries that make use of the IDms class library. These libraries provide interfaces/classes that make sense to the solution. These libraries can then be used in Automation Scripts, Protocols and external tools in C# code.
In order to reuse these libraries in our ad-hoc data sources, we want to retrieve an object that implements the IDms interface as provided by Skyline.DataMiner.Core.DataMinerSystem.Common library. We would like to retrieve the object from the IGQIOnInit | DataMiner Docs method. The GQIDMS class does not implement any interface and IMO would make more sense that the class implements the IDms interface as this is now the most common way to retrieve information from a DMS.
With the current features available, what is the best way to get an object that implements the IDms interface from the IGQIOnInit method?
Hi all,
Starting from DM Feature release 10.4.6 or DM Main release 10.5.0, DM now exposes the underlying GQI SLNET connection to extensions like Ad Hoc data sources and custom operators.
More information can be found on DataMiner Docs: GQIDMS class | DataMiner Docs
Hi Michiel, currently there is no straightforward way to get an IDms object in the IGQIOnInit method. However, by creating a class that implements the ICommunication interface, based on the GQIDMS you can get close. Unfortunately the GQIDMS object lacks methods to use subscriptions, so it could be that certain functionality doesn’t work.
public class DataSource : IGQIOnInit
{
public OnInitOutputArgs OnInit(OnInitInputArgs args)
{
var dms = DmsFactory.CreateDms(new GqiConnection(args.DMS));}
}
public class GqiConnection : ICommunication
{
private readonly GQIDMS _gqiDms;public GqiConnection(GQIDMS gqiDms)
{
_gqiDms = gqiDms ?? throw new ArgumentNullException(nameof(gqiDms));
}public DMSMessage[] SendMessage(DMSMessage message)
{
return _gqiDms.SendMessages(message);
}public DMSMessage SendSingleResponseMessage(DMSMessage message)
{
return _gqiDms.SendMessage(message);
}public DMSMessage SendSingleRawResponseMessage(DMSMessage message)
{
return _gqiDms.SendMessage(message);
}public void AddSubscriptionHandler(NewMessageEventHandler handler)
{
throw new NotImplementedException();
}public void AddSubscriptions(NewMessageEventHandler handler, string handleGuid, SubscriptionFilter[] subscriptions)
{
throw new NotImplementedException();
}public void ClearSubscriptionHandler(NewMessageEventHandler handler)
{
throw new NotImplementedException();
}public void ClearSubscriptions(NewMessageEventHandler handler, string handleGuid, bool replaceWithEmpty = false)
{
throw new NotImplementedException();
}
}
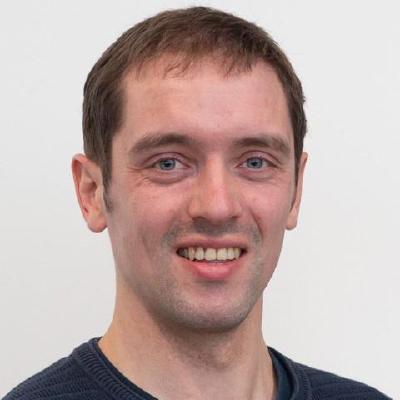
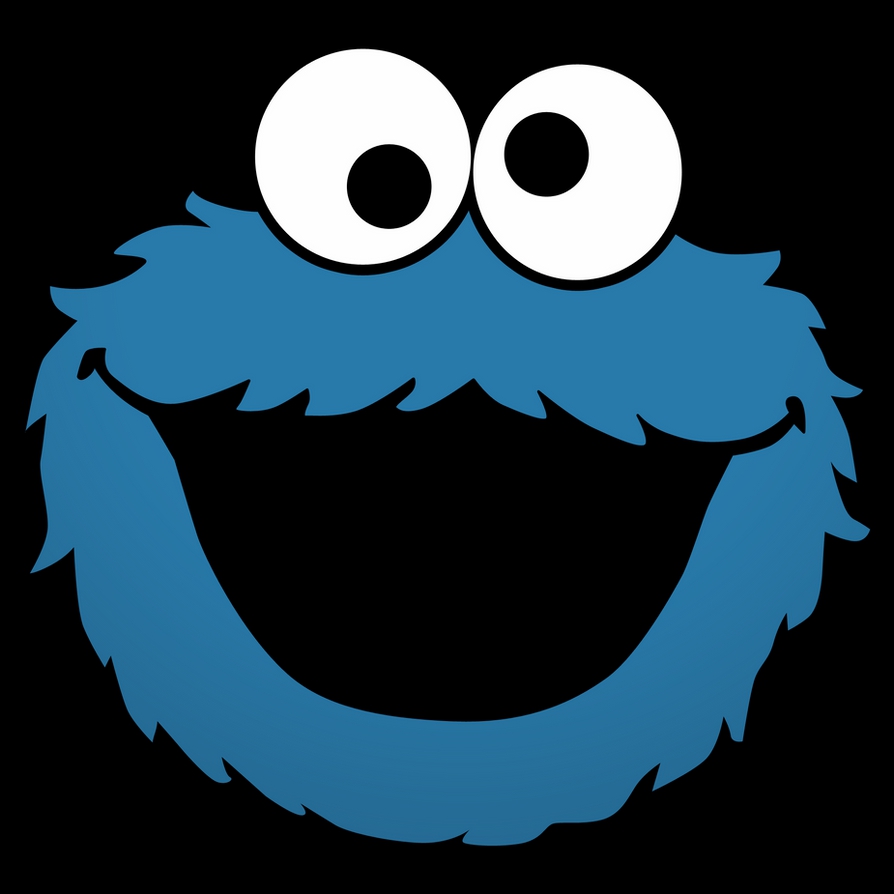
Hi Tom,
I was able to compile from the automation module and the source is available on my dashboards, however when I select it I get the following error:
Error trapped: Error thrown in implementation of OnInit: Could not load file or assembly ‘SLManagedAutomation, Version=1.0.0.0, Culture=neutral, PublicKeyToken=null’ or one of its dependencies. An attempt was made to load a program with an incorrect format.
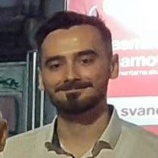
Hello Tom,
Seems like this method will only work if you have single responses from the SLNet calls.
For example: The GetElements will only return one element currently.
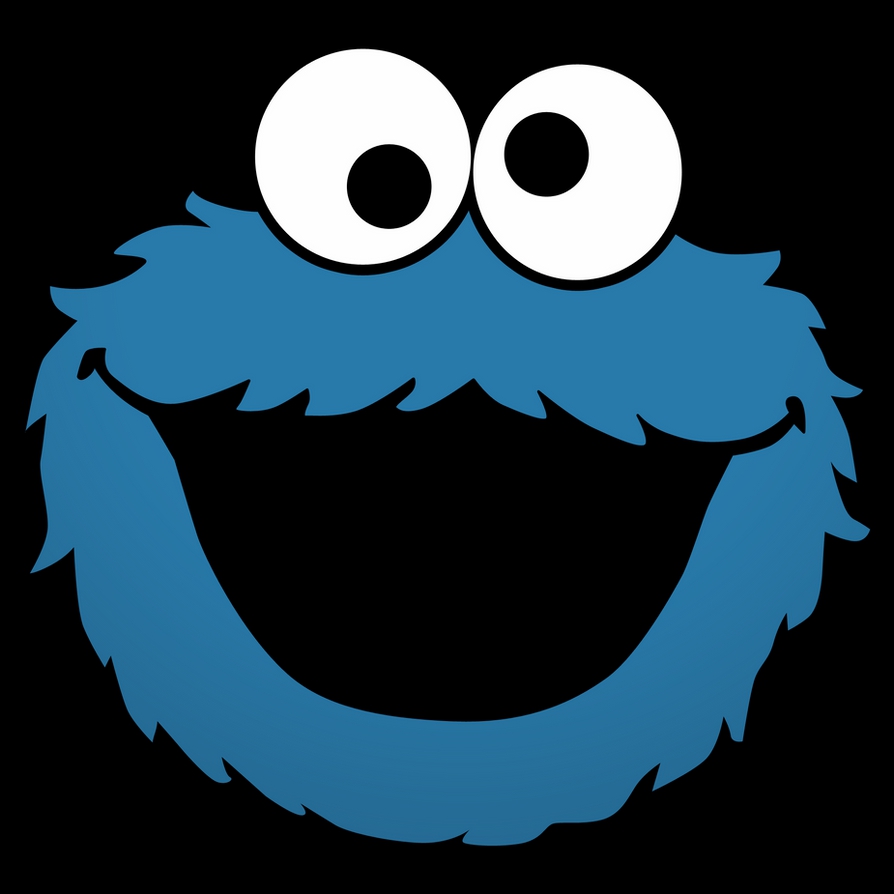
FYI: If you change the DMSMessage[] SendMessage() implementation to return _gqiDms.SendMessages(message) instead it will work.
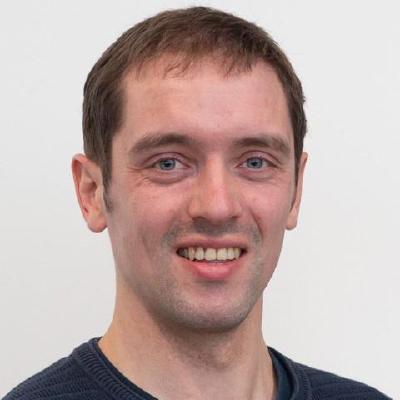
Indeed, I’ve updated my answer. The class can also be downloaded from https://gist.github.com/TomW-Skyline/188d70e8cb775087e7718359fab9a158.
Another option could be to use the static “Engine.SLNetRaw” property, but not sure if that works or not:
var dms = DmsFactory.CreateDms(new RemotingCommunication(Engine.SLNetRaw));