I have tried to use the UIResult GetString() method and it works but the WasOnChange method always returns false. Can anyone confirm if there is better method to check event changes with a UIBlockType.DropDown.
Hi Curtis,
Back in the days when I implemented some enhancements/options on the dropdown in Interactive Automation, I used the following C# block as a base for my other test scripts.
It generates an information event when the value of a dropdown has changed.
Hope this helps!
using System; using System.Collections.Generic; using Skyline.DataMiner.Automation; public class Script { public void Run(Engine engine) { UIResults uir = null; string selectedVal = "option 3"; List<string> dropDownOptions = new List<string>(); for (int i = 0; i < 10; i++) { if (i % 2 == 0) //even = value, oneven = otion { dropDownOptions.Add(string.Format("value {0}", i)); } else { dropDownOptions.Add(string.Format("option {0}", i)); } } string extraOptions = String.Join(";", dropDownOptions); do { UIBuilder uib = new UIBuilder(); uib.RequireResponse = true; uib.RowDefs = "a;25;a;25;a"; uib.ColumnDefs = "150;200"; uib.AddStaticText("Dropdown_Change+filter", 1, 0); uib.AddDropDown("cbBox4", selectedVal, dropDownOptions, 1, 1, width: 200, wantsOnChange: true, displayFilter: true); uib.AddButton("Close", "close", 3, 1, 170, 25); uir = engine.ShowUI(uib); if (uir.WasOnChange("cbBox4")) { selectedVal = uir.GetString("cbBox4"); engine.GenerateInformation(string.Format("SELECTED DROPDOWN VALUE: {0}", selectedVal)); } } while (!uir.WasButtonPressed("close")); } } public static class AutomationUIExtensions { public const string InitialDropDownValue = "-Select-"; #region Public Enums public enum UIBlockStyle { Title1, Title2, Title3 }; public enum UIBlockHorizontalAlignment { Left, Center, Right }; public enum UIBlockVerticalAlignment { Top, Center, Bottom }; #endregion #region Public Methods public static void AddStaticText(this UIBuilder uib, string text, uint row, uint column, uint rowSpan = 1, uint columnSpan = 1, UIBlockHorizontalAlignment horizontalAlignment = UIBlockHorizontalAlignment.Left, UIBlockVerticalAlignment verticalAlignment = UIBlockVerticalAlignment.Center, string margin = "", bool isMultiLine = false) { UIBlockDefinition uibDef = new UIBlockDefinition(); uibDef.Type = UIBlockType.StaticText; uibDef.Text = text; uibDef.Row = (int)row; uibDef.RowSpan = (int)rowSpan; uibDef.Column = (int)column; uibDef.ColumnSpan = (int)columnSpan; uibDef.HorizontalAlignment = GetHorizontalAlignment(horizontalAlignment); uibDef.VerticalAlignment = GetVerticalAlignment(verticalAlignment); uibDef.IsMultiline = isMultiLine; if (!string.IsNullOrEmpty(margin)) { uibDef.Margin = margin; } uib.AppendBlock(uibDef); } public static void AddDropDown(this UIBuilder uib, string destVar, string initialValue, List<string> dropDownOptions, uint row, uint column, uint rowSpan = 1, uint columnSpan = 1, int width = -1, int minWidth = -1, int maxWidth = -1, int height = -1, int minHeight = -1, int maxHeight = -1, UIBlockHorizontalAlignment horizontalAlignment = UIBlockHorizontalAlignment.Left, UIBlockVerticalAlignment verticalAlignment = UIBlockVerticalAlignment.Center, string margin = "", bool wantsOnChange = false, bool displayFilter = false) { UIBlockDefinition uibDef = new UIBlockDefinition(); uibDef.Type = UIBlockType.DropDown; uibDef.DestVar = destVar; uibDef.InitialValue = initialValue; uibDef.Row = (int)row; uibDef.RowSpan = (int)rowSpan; uibDef.Column = (int)column; uibDef.ColumnSpan = (int)columnSpan; uibDef.Width = width; uibDef.MinWidth = minWidth; uibDef.MaxWidth = maxWidth; uibDef.Height = height; uibDef.MinHeight = minHeight; uibDef.MaxHeight = maxHeight; uibDef.HorizontalAlignment = GetHorizontalAlignment(horizontalAlignment); uibDef.VerticalAlignment = GetVerticalAlignment(verticalAlignment); uibDef.WantsOnChange = wantsOnChange; uibDef.DisplayFilter = displayFilter; if (initialValue == InitialDropDownValue) { uibDef.AddDropDownOption(InitialDropDownValue); } foreach (string sOption in dropDownOptions) { uibDef.AddDropDownOption(sOption); } if (!string.IsNullOrEmpty(margin)) { uibDef.Margin = margin; } uib.AppendBlock(uibDef); } public static void AddDropDown(this UIBuilder uib, string destVar, string initialValue, List<string[]> dropDownOptions, uint row, uint column, uint rowSpan = 1, uint columnSpan = 1, int width = -1, int minWidth = -1, int maxWidth = -1, int height = -1, int minHeight = -1, int maxHeight = -1, UIBlockHorizontalAlignment horizontalAlignment = UIBlockHorizontalAlignment.Left, UIBlockVerticalAlignment verticalAlignment = UIBlockVerticalAlignment.Center, string margin = "", bool wantsOnChange = false) { UIBlockDefinition uibDef = new UIBlockDefinition(); uibDef.Type = UIBlockType.DropDown; uibDef.DestVar = destVar; uibDef.InitialValue = initialValue; uibDef.Row = (int)row; uibDef.RowSpan = (int)rowSpan; uibDef.Column = (int)column; uibDef.ColumnSpan = (int)columnSpan; uibDef.Width = width; uibDef.MinWidth = minWidth; uibDef.MaxWidth = maxWidth; uibDef.Height = height; uibDef.MinHeight = minHeight; uibDef.MaxHeight = maxHeight; uibDef.HorizontalAlignment = GetHorizontalAlignment(horizontalAlignment); uibDef.VerticalAlignment = GetVerticalAlignment(verticalAlignment); uibDef.WantsOnChange = wantsOnChange; foreach (string[] asOption in dropDownOptions) { uibDef.AddDropDownOption(asOption[0], asOption[1]); } if (!string.IsNullOrEmpty(margin)) { uibDef.Margin = margin; } uib.AppendBlock(uibDef); } public static void AddButton(this UIBuilder uib, string text, string destVar, uint row, uint column, uint width, uint height, uint rowSpan = 1, uint columnSpan = 1, UIBlockHorizontalAlignment horizontalAlignment = UIBlockHorizontalAlignment.Left, UIBlockVerticalAlignment verticalAlignment = UIBlockVerticalAlignment.Center, string margin = "") { UIBlockDefinition uibDef = new UIBlockDefinition(); uibDef.Type = UIBlockType.Button; uibDef.Text = text; uibDef.DestVar = destVar; uibDef.Row = (int)row; uibDef.RowSpan = (int)rowSpan; uibDef.Column = (int)column; uibDef.ColumnSpan = (int)columnSpan; uibDef.Width = (int)width; uibDef.Height = (int)height; uibDef.HorizontalAlignment = GetHorizontalAlignment(horizontalAlignment); uibDef.VerticalAlignment = GetVerticalAlignment(verticalAlignment); if (!string.IsNullOrEmpty(margin)) { uibDef.Margin = margin; } uib.AppendBlock(uibDef); } #endregion #region Private Methods private static string GetStyle(UIBlockStyle style) { switch (style) { case UIBlockStyle.Title1: return "Title1"; case UIBlockStyle.Title2: return "Title2"; case UIBlockStyle.Title3: return "Title3"; default: return "Title3"; } } private static string GetHorizontalAlignment(UIBlockHorizontalAlignment horizontalAlignment) { switch (horizontalAlignment) { case UIBlockHorizontalAlignment.Left: return "Left"; case UIBlockHorizontalAlignment.Center: return "Center"; case UIBlockHorizontalAlignment.Right: return "Right"; default: return "Left"; } } private static string GetVerticalAlignment(UIBlockVerticalAlignment verticalAlignment) { switch (verticalAlignment) { case UIBlockVerticalAlignment.Top: return "Top"; case UIBlockVerticalAlignment.Center: return "Center"; case UIBlockVerticalAlignment.Bottom: return "Bottom"; default: return "Center"; } } #endregion }
Hello Matthias, thank you for your code example, it really helps. I have one question: For my script I want to select a value but only make changes after a button is clicked, I don’t want the change to happen straight away. Do I have to set the WantsOnChange param to false, and if I do that I am not sure how to use UIResult to get the selected value.
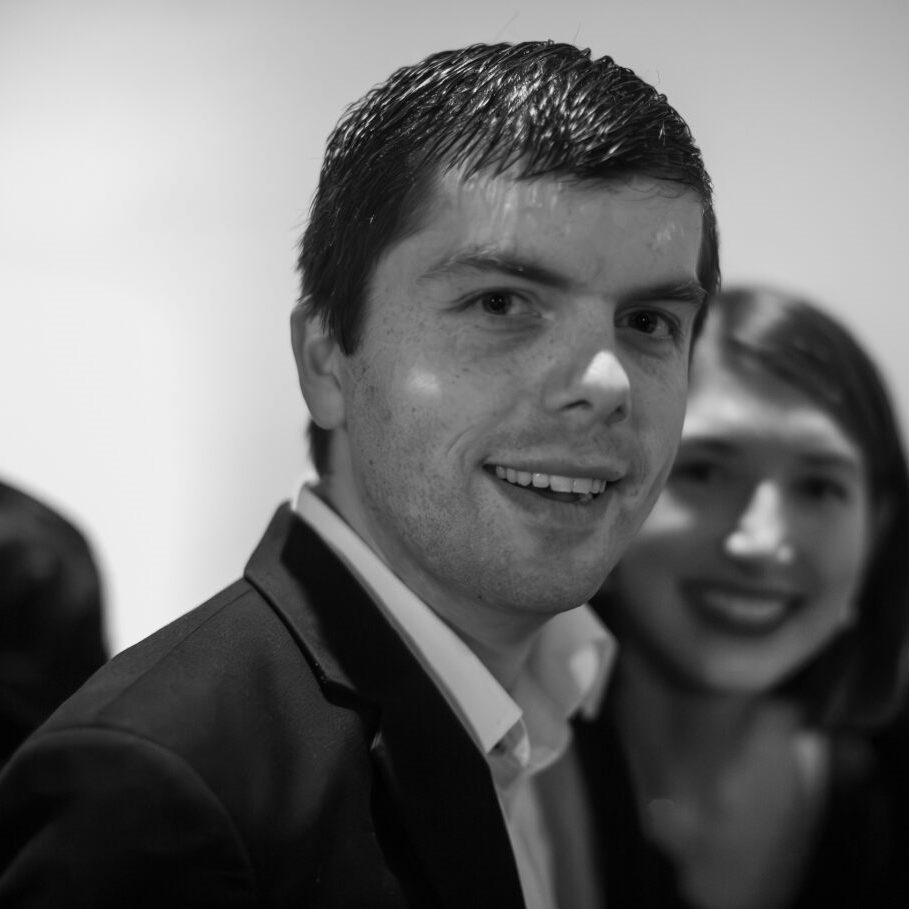
Hi Curtis, UIresult will work as the same.
When you press the button, all loaded destination variables will fill in their correspondent value. So the one of your dropdown will be the selected value.
that collection of variables will be passed from client to server, which then will be parsed into sort of dictionary. The UIresult will then look up by variable name. You can always try and experiment, for example duplicate the script and figure it out ☺ Hope this helps you further.
I have managed to make it work after all your with a bit of experimenting, thank you
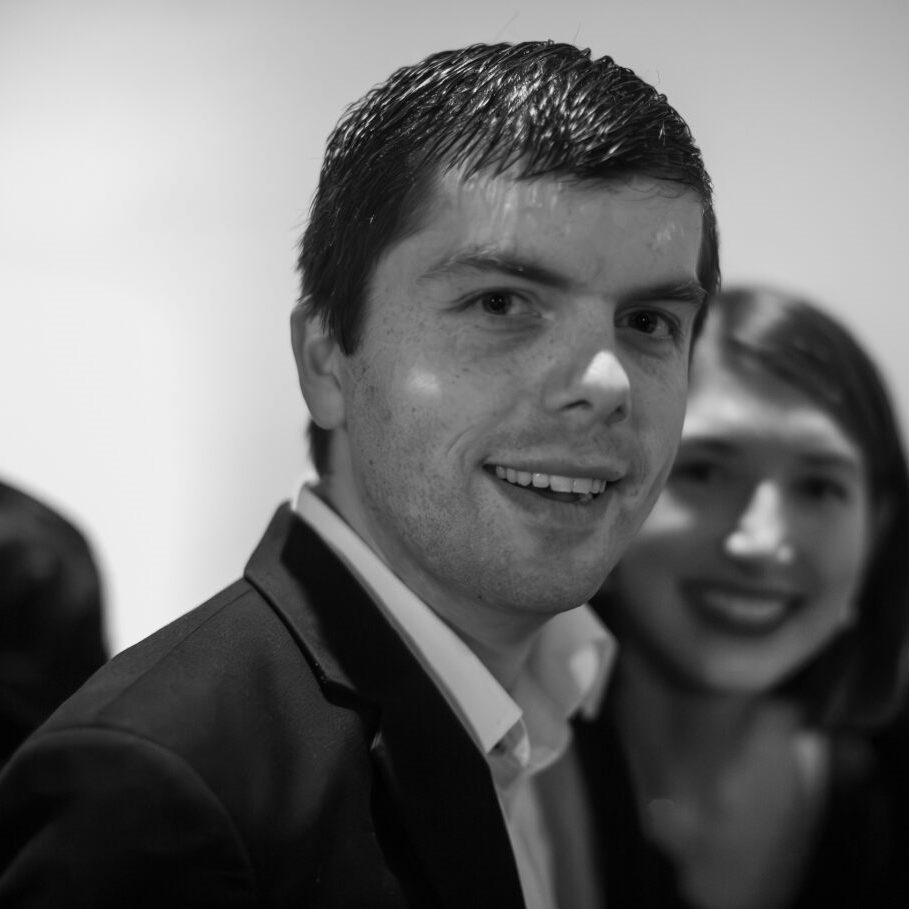
Curtis, that’s great to hear! Hopefully you gained bit of experience as well 🙂 In order to finish up this post thread, could you mark this answer as Best answer? Just simply select the check marker on the top left corner of this answer. Thanks in advance and have a nice day further.
Hi Curtis,
How are you defining your Dropdown block definition?
If you use the uibuilder.AppendDropDown, will not enable the on-change option for you.
You would need to define a UIBlockDefinition and use the method uibuilder.AppendBlock and in the UIBlockDefinition you need to make sure you set the WantsOnChange property to true
I just set the this parameter to be true but now every time I select a different option in the dropdown my script crashes.
UIBlockDefinition dropdownBlockItem = new UIBlockDefinition
{
Type = UIBlockType.DropDown,
Text = “Polling”,
InitialValue = initialValue,
Row = row,
Column = column,
Width = 70,
DestVar = “dropdown_polling”,
WantsOnChange = true
};
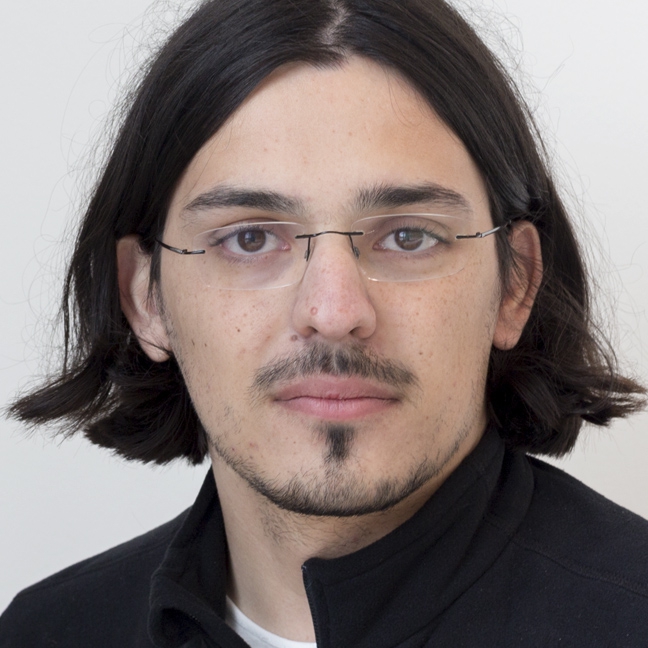
Do you have an error message or does it show anything in the information events that would indicate the cause of your crash?
According to the documentation link on my original answer, it should be compatible with dropdowns
No error messages but I might have used the wrong word. In DataMiner terms it completed succeeded after a change. It is working but ends the script, which is strange.
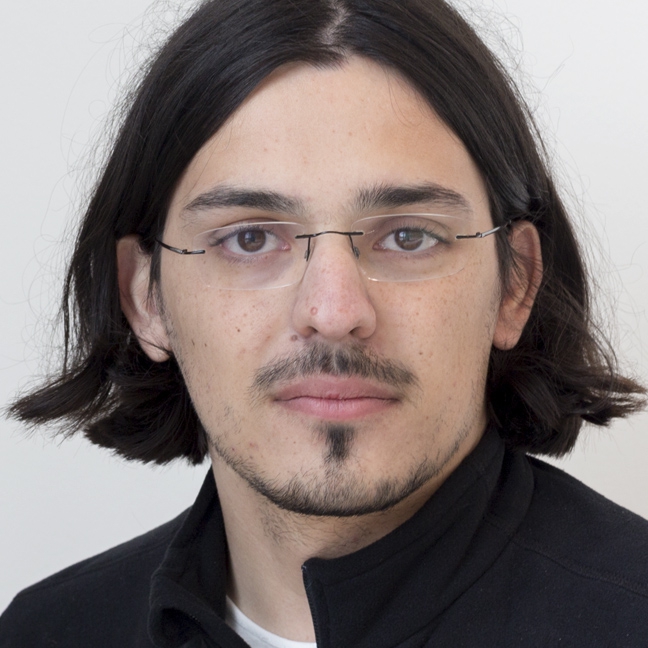
Without knowing how your entire code is, I could be suggesting things you already have in place, however, I would check if you have the UIResults object verifying the events and reading the data from the block definitions.
After those events are checked and if you still want to remain on the same screen, there should be a loop that sends you back to the code where it draws the screen.
Hi Curtis,
Did you hear about the Interactive Automation Script Toolkit?
You should be able to use this to implement a dropdown,
in the dialog you could do something like:
ResourceSelectionDropDown = new DropDown { Width = 300, IsDisplayFilterShown = true };
AddWidget(ResourceSelectionDropDown, 0, 0);
ResourceSelectionDropDown.Changed += Resource_Selected;
and your method would look like
private void Resource_Selected(object sender, DropDown.DropDownChangedEventArgs e)
{if (String.IsNullOrWhiteSpace(ResourceSelectionDropDown.Selected) || ResourceSelectionDropDown.Selected == Na)
{
return;
}
// do something with ResourceSelectionDropDown.Selected}
Hope this help a bit.
Thank you for sharing this but I am far too gone in my script to use this so if you have anything to help with BlockType elements, please do share.
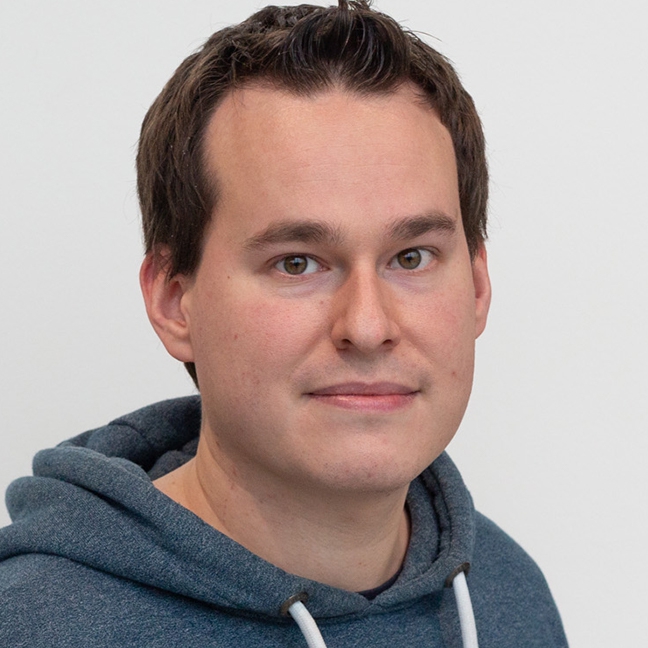
Hi Curtis, I would still highly recommend to work with the IAS Toolkit. Regardless of the size of you current script you will, in the long run, increase your readability and the maintainability to rework it. I don’t think Skyline has created scripts implementing this kind of logic in the last +/-4 years.
That being said, I do understand your position so, there is no better way to get events on UIBlocks that I know of…. There might be something wrong with the structure of your code? In the background an IAS using UIBlocks requires a do while loop. In the do you will implement your uiblocks and uir.WasOnChange(“DropdownName”). But i’m not an expert on that, I hope someone else can further help you out with this.
this problem is solved now