I need to create a view and add elements to it. Therefore I try to execute following code:
IDmsView rootView = dms.GetView(viewId);
ViewConfiguration edgeDevicesSourceViewConfiguration = new ViewConfiguration(“1. Edge Devices – Source [VSG]”, rootView);
IDmsView edgeDevicesSourceView = dms.GetView(dms.CreateView(edgeDevicesSourceViewConfiguration));
Unfortunately this results in a ViewNotFoundException.
What is the best way to retrieve my new view?
I guess adding this method in a loop, but what is the max time to wait to prevent an infinite loop?
Views are indeed created asynchronously in DataMiner. They are not immediately available after sending the request to create the new view.
Few possibilities to wait until the view is created, without a loop that blocks everything else:
- Store the data of the requested new views in a QAction instance (private non-static field). Frequently trigger the QAction with a timer and check which views are already available.
- Subscription that receives a notification when a new view is created.
- Note that this is an internal API and we do not recommend using this, as it is not officially supported and we cannot guarantee that it will still work in the future. As a rule, you should avoid using subscriptions, as these are subject to change without notice. We recommend to instead always use the correct UI or automation options provided in DataMiner Automation or through our web API.
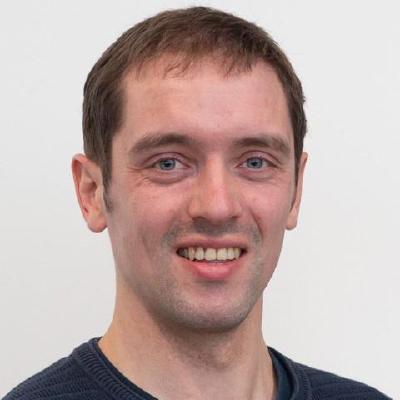
Wasn’t aware that it’s an automation script. In that case you are probably best of with a loop unless there is a blocking call in DataMiner itself that waits until the view has been created.
I will do it like that. Thanks!
Hi. Wondering what’s the best way to have this working out. I’ve tried to create 2 views one after the other, as subviews, but it fails to get the newly created view, after the second one.
private void BuildByHand()
{
IDmsView dmsRootView = dms.GetView(“dmsRootView”);
engine.GenerateInformation($”GetView(\”dmsRootView\”) {dmsRootView.Id}”);
engine.GenerateInformation($”Create view {dmsRootView.Name}\\Subview1″);
var subview1Configuration = new ViewConfiguration(“Subview1″, dmsRootView);
int subview1ViewId = dms.CreateView(subview1Configuration);
engine.GenerateInformation($”Subview1 View ID: {subview1ViewId}”);
Thread.Sleep(5000);
IDmsView subview1View = dms.GetView(subview1ViewId);
engine.GenerateInformation($”GetView(\”Subview1\”) {subview1View.Id}”);
engine.GenerateInformation($”Create view {subview1View.Name}\\Subview2″);
var subview2Configuration = new ViewConfiguration(“Subview2″, subview1View);
int subview2ViewId = dms.CreateView(subview2Configuration);
engine.GenerateInformation($”subview2 View ID: {subview2ViewId}”);
Thread.Sleep(5000);
IDmsView subview2View = dms.GetView(subview2ViewId);
engine.GenerateInformation($”GetView(\”Subview2\”) {subview2View.Id}”);
}
Thanks
Actually this should be working. The name of the view should be unique on the dms system.
Hi Tom, how do you see this implemented in Automation Scripts?