I have two buttons in the dialog which I created from the script but on press although event is registered it works for one and not for other
_dialog.SearchButton.Pressed += SearchButton_Pressed;
_dialog.SaveButton.Pressed += SaveButton_Pressed;
I dont know what can be reason here?
Hi Apurva,
It's difficult to pinpoint the issue without seeing more of your code. Could you please share additional details, such as how the buttons are initialized or if there are any conditions that might affect their behavior?
I can confirm that it's definitely possible to have multiple buttons in a dialog, each with its own event handler. Once we have more context, we can help troubleshoot the issue further.
Kind regards,
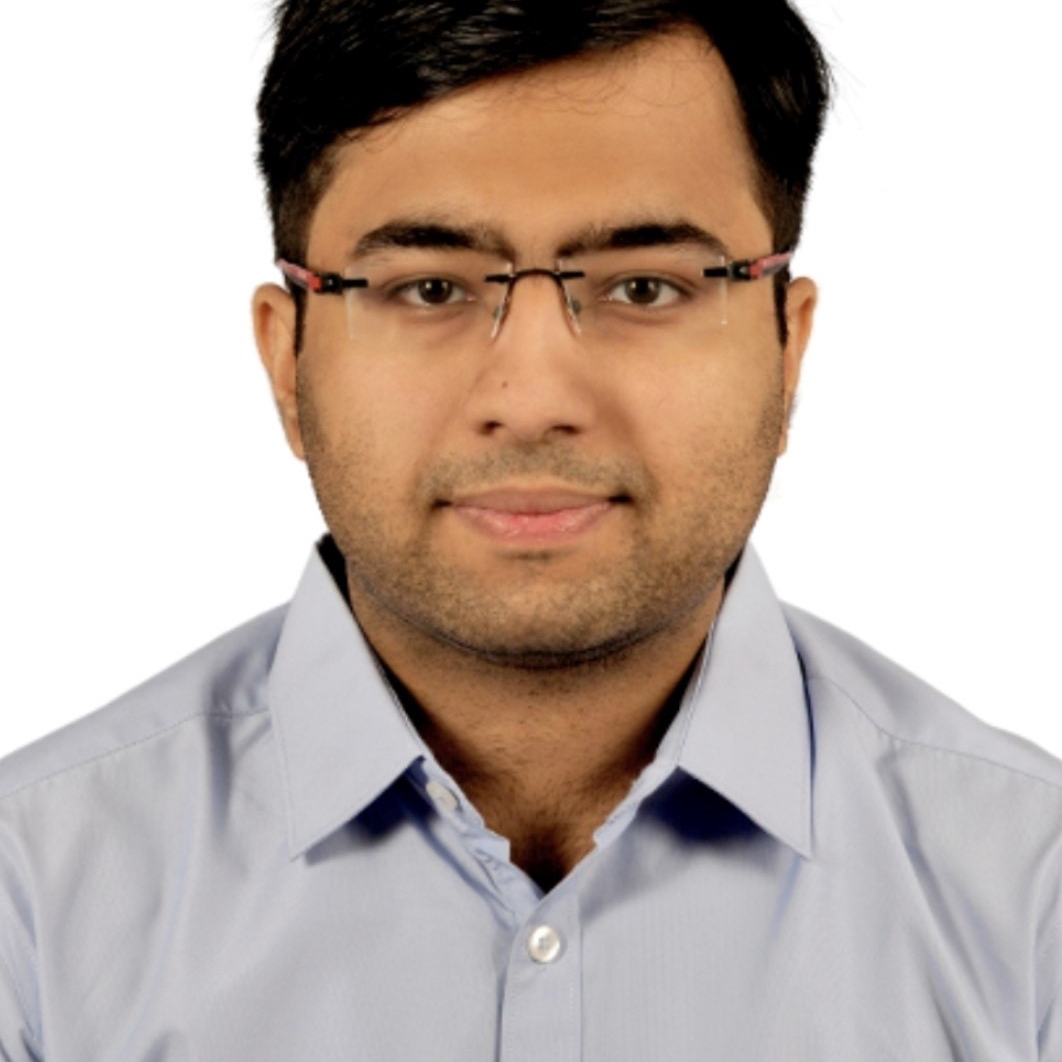
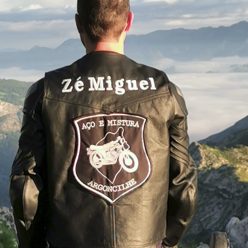
In the SearchButton_Pressed method, a new CreateElementDialog is being instantiated after the search operation completes:
_dialog = new CreateElementDialog(_engine, _elementList, _elementEdit, _dialog.ElementIpTextBox.Text);
This effectively replaces the old dialog with a new one, which might also replace the existing button event handlers. If the dialog is replaced after the SearchButton is pressed, the SaveButton might lose its event handler because it’s part of a new dialog instance.
Solution: After creating a new CreateElementDialog, you need to re-register the event handlers for the buttons again, or better, avoid re-instantiating the dialog if it’s unnecessary.
Update your code as follows:
_dialog = new CreateElementDialog(_engine, _elementList, _elementEdit, _dialog.ElementIpTextBox.Text);
_dialog.SearchButton.Pressed += SearchButton_Pressed; // Re-register event handlers
_dialog.SaveButton.Pressed += SaveButton_Pressed;
Let me know if this solves your problem
Kind regards,
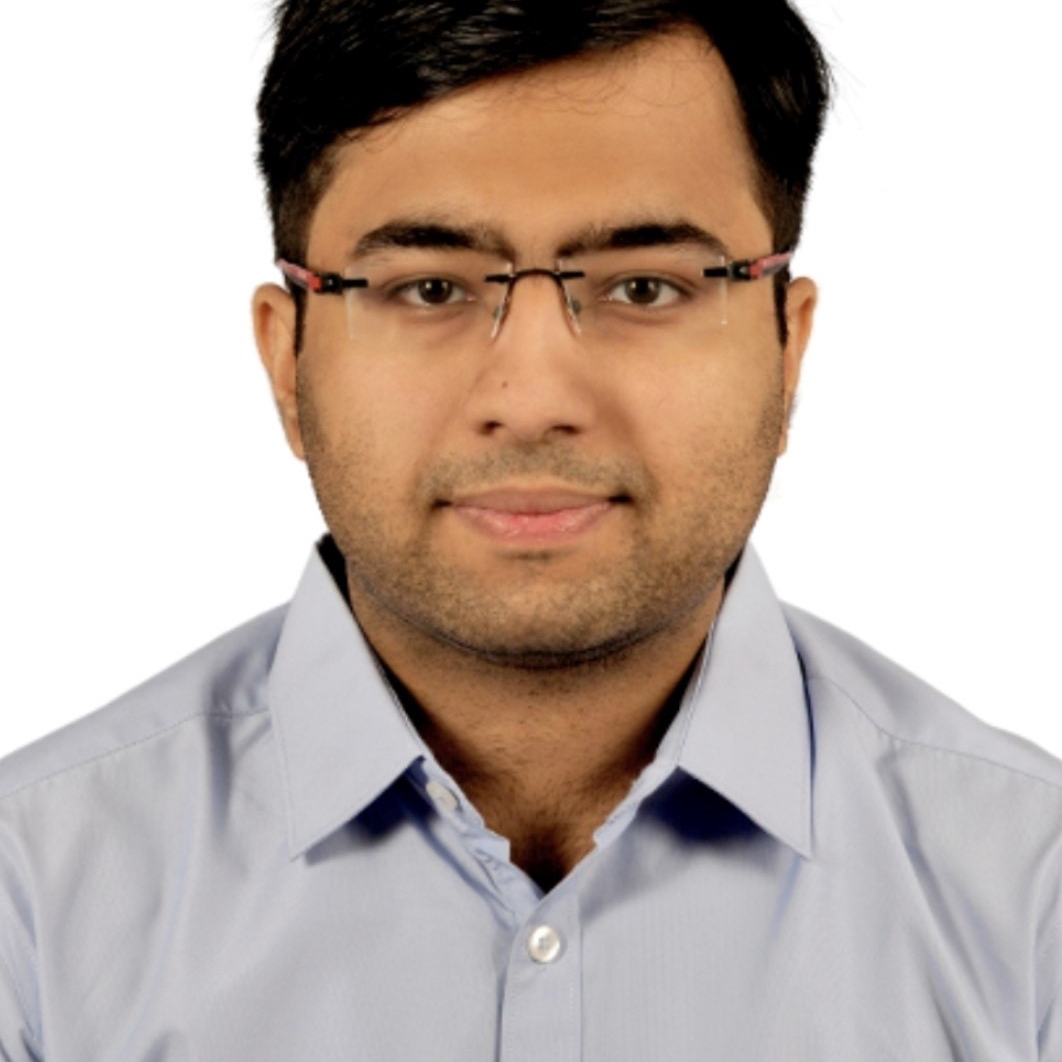
Hi thanks, yes it worked 🙂
public CreateElementDialog(IEngine engine,List _elementList,string option,string elementIp) : base(engine)
{
this.Title = “Add Elements”;
Label elementIpLabel = new Label(“Element IP *”);
ElementNameLabel = new Label(“Element Name”);
SearchButton = new Button(“Search”);
SaveButton = new Button(“Save”);
ValidationLabel = new Label(string.Empty);
engine.Log(“option-“+ option);
engine.Log(“_elementList count-” + _elementList.Count);
if (option.Equals(“Create”, StringComparison.CurrentCultureIgnoreCase))
{
ElementIpTextBox = new TextBox();
ElementNameText=new Label(string.Empty);
SaveButton.IsVisible = false;
ElementNameLabel.IsVisible=false;
ElementNameText.IsVisible = false;
AddWidget(ElementNameText, 1, 1);
}
else
{
ElementIpTextBox = new TextBox(elementIp);
if (_elementList.Count > 1)
{
ElementDropDown = new DropDown(_elementList.Select(x=>x.ElementName).ToList());
ElementDropDown.IsVisible = true;
AddWidget(ElementDropDown, 1, 1);
}
else if(_elementList.Count == 1)
{
ElementNameText = new Label(_elementList.Select(x => x.ElementName).FirstOrDefault());
ElementNameText.IsVisible = true;
AddWidget(ElementNameText, 1, 1);
}
SaveButton.IsVisible = true;
ElementNameLabel.IsVisible = true;
}
AddWidget(elementIpLabel, 0, 0);
AddWidget(ElementIpTextBox, 0, 1);
AddWidget(SearchButton, 0, 2);
AddWidget(ElementNameLabel, 1, 0);
AddWidget(ValidationLabel, 2, 2);
AddWidget(SaveButton, 3, 1);
}
private IEngine _engine;
private CreateElementDialog _dialog;
private LogHelper _logHelper;
private ServiceHelper _serviceHelper;
private DMS _dms;
private const int elementIdType = 76;
private const int SubType = 0;
private string _elementCreate=”Create”;
private string _elementEdit = “Edit”;
private string protocolName;
private List _elementList=new List();
private string truckId;
private static UserDefinedAppHelper _appHelper;
public void Run(IEngine engine)
{
// .ShowUI(); Don’t remove this comment, this is needed for interactive automation.
_logHelper = new LogHelper(engine as Engine);
try
{
_engine = engine;
_engine.Log(“Add Elements started”);
_dms=new DMS();
_appHelper = new UserDefinedAppHelper(engine);
_dialog = new CreateElementDialog(engine, _elementList, _elementCreate,string.Empty);
// _serviceHelper = new ServiceHelper(engine as Engine);
_engine.Log(“Line 106”);
_dialog.SearchButton.Pressed += SearchButton_Pressed;
_dialog.SaveButton.Pressed += SaveButton_Pressed;
do
{
_dialog.Show();
}
while (!_dialog.Close);
}
catch (Exception e)
{
_logHelper.Log(LogType.Error, “Add Element”, e.Message);
Dialogs.ShowMessageDialog(engine as Engine, “Failed adding OB element”, e.Message, e.StackTrace);
}
}
private void SearchButton_Pressed(object sender, EventArgs e)
{
try
{
_engine.Log(“Search started”);
_elementList = new List();
_engine.Log(“Element IP = ” + _dialog.ElementIpTextBox.Text);
string[] ipBus = { _dialog.ElementIpTextBox.Text };
_dms.Notify(elementIdType /*DMS_GET_ELEMENT_ID_FROM_IP*/, SubType, ipBus, null, out var element);
_engine.Log(“element id-” + element);
if (element == null)
throw new Exception(“Element not found”);
else
{
if (element.ToString().Contains(“;”))
{
var elementsList = element.ToString().Split(‘;’);
foreach (var elements in elementsList)
{
_elementList.Add(new ElementDetails
{
ElementName = GetElementName(elements),
ElementId = elements
});
}
}
else
{
_elementList.Add(new ElementDetails
{
ElementName = GetElementName(element.ToString()),
ElementId = element.ToString()
});
}
}
_dialog = new CreateElementDialog(_engine, _elementList, _elementEdit, _dialog.ElementIpTextBox.Text);
}
catch (Exception ex)
{
_logHelper.Log(LogType.Error, “Create Truck”, ex.Message);
throw;
}
}
private void SaveButton_Pressed(object sender, EventArgs e)
{
try
{
_engine.GenerateInformation(“Save started”);
truckId = _appHelper.GetAppValue(“Truck Id”);
DomHelper domHelper = new DomHelper(_engine.SendSLNetMessages, Ob_Resourcemanagement.ModuleId);
string elementId = _elementList
.Where(x => x.ElementName.Equals(_dialog.ElementNameText.Text,
StringComparison.CurrentCultureIgnoreCase)).Select(y => y.ElementId).ToString();
_engine.GenerateInformation(“elementId-“+ elementId);
var elementInfoSection = new Section
{
SectionDefinitionID = Ob_Resourcemanagement.Sections.Elements.Id,
};
elementInfoSection.AddOrReplaceFieldValue(new FieldValue(Ob_Resourcemanagement.Sections.Elements.Device_Ip, new ValueWrapper(_dialog.ElementIpTextBox.Text)));
elementInfoSection.AddOrReplaceFieldValue(new FieldValue(Ob_Resourcemanagement.Sections.Elements.Element_Name, new ValueWrapper(_dialog.ElementNameText.Text)));
elementInfoSection.AddOrReplaceFieldValue(new FieldValue(Ob_Resourcemanagement.Sections.Elements.Protocol_Name, new ValueWrapper(protocolName)));
elementInfoSection.AddOrReplaceFieldValue(new FieldValue(Ob_Resourcemanagement.Sections.Elements.Element_Id, new ValueWrapper(elementId)));
elementInfoSection.AddOrReplaceFieldValue(new FieldValue(Ob_Resourcemanagement.Sections.Elements.Truck_Id, new ValueWrapper(truckId)));
var newElementInstance = new DomInstance
{
DomDefinitionId = Ob_Resourcemanagement.Definitions.Truckelements,
};
_engine.GenerateInformation(“line 197”);
newElementInstance.Sections.Add(elementInfoSection);
_engine.GenerateInformation(“line 199”);
domHelper.DomInstances.Create(newElementInstance);
_engine.GenerateInformation(“line 201”);
_dialog.Close = true;
}
catch (Exception ex)
{
_logHelper.Log(LogType.Error, “Create Truck”, ex.Message);
throw;
}
}
so SaveButtonClicked doesn’t works but searchButtonClicked works