I need to configure 2 different api with routes – “heartbeat” and “notifications” in the same script. Earlier when I clicked “Configure API”, I was able to see a dialog box which had option to select either of the api to open and edit but now I am unable to see it which results in only one api hosted at a time which should not happen. Please advise how to configure both api’s in my script.
Hi Harinee,
To view and configure your User-Defined API definitions, you can either open them from the script as you are doing, or view them all in the System Center as explained on this docs page: https://docs.dataminer.services/user-guide/Advanced_Modules/User-Defined_APIs/UD_APIs_Viewing_in_Cube.html#configuring-apis-and-tokens.
When you link multiple API definitions to the same script, and try to configure the API from the Automation window, you should indeed get a pop-up asking you to select which one you would like to configure. If you cannot see that pop-up, I would assume that there is only one definition configured. Can you check on the System Center page?
Hi Harinee,
My advice would be to configure 1 API definition on the script and then update the script so the routes are divided within the same API definition. Different repsonse can then be passed for each route.
I have an example to showcase you on how you can change results based on route:
This example shows us that different data can be passed as output, based on the route that is given. For instance, the Info route will pass information (config) data, while Metrics will be effective measured data as JSON.
UPDATE: If there’s a need to have separate handling, such as different token, then it is possible to have multiple definitions:
I do hope this answer will help you further.
Looking forward to your positive reply.
I am using a similar way in handling both routes..not sure if I need to add something else here..
[AutomationEntryPoint(AutomationEntryPointType.Types.OnApiTrigger)]
public ApiTriggerOutput OnApiTrigger(IEngine engine, ApiTriggerInput requestData)
{
try
{
var method = requestData.RequestMethod;
var route = requestData.Route;
var body = requestData.RawBody;
engine.Log($"API Triggered: Route = {route}, Method = {method}, Body= {body}");
if (method != RequestMethod.Post)
{
return new ApiTriggerOutput
{
ResponseBody = "Only POST requests are supported.",
ResponseCode = (int)StatusCode.MethodNotAllowed,
};
}
if (route == "heartbeat")
{
return HandleHeartbeatRequest(engine, body);
}
else if (route == "notifications")
{
return HandleNotificationRequest(engine, body);
}
else
{
return new ApiTriggerOutput
{
ResponseBody = "Invalid route. Please check the endpoint URL.",
ResponseCode = (int)StatusCode.NotFound,
};
}
}
catch (Exception ex)
{
engine.Log($"Error: {ex.Message}");
return new ApiTriggerOutput
{
ResponseBody = "An internal server error occurred.",
ResponseCode = (int)StatusCode.InternalServerError,
};
}
}
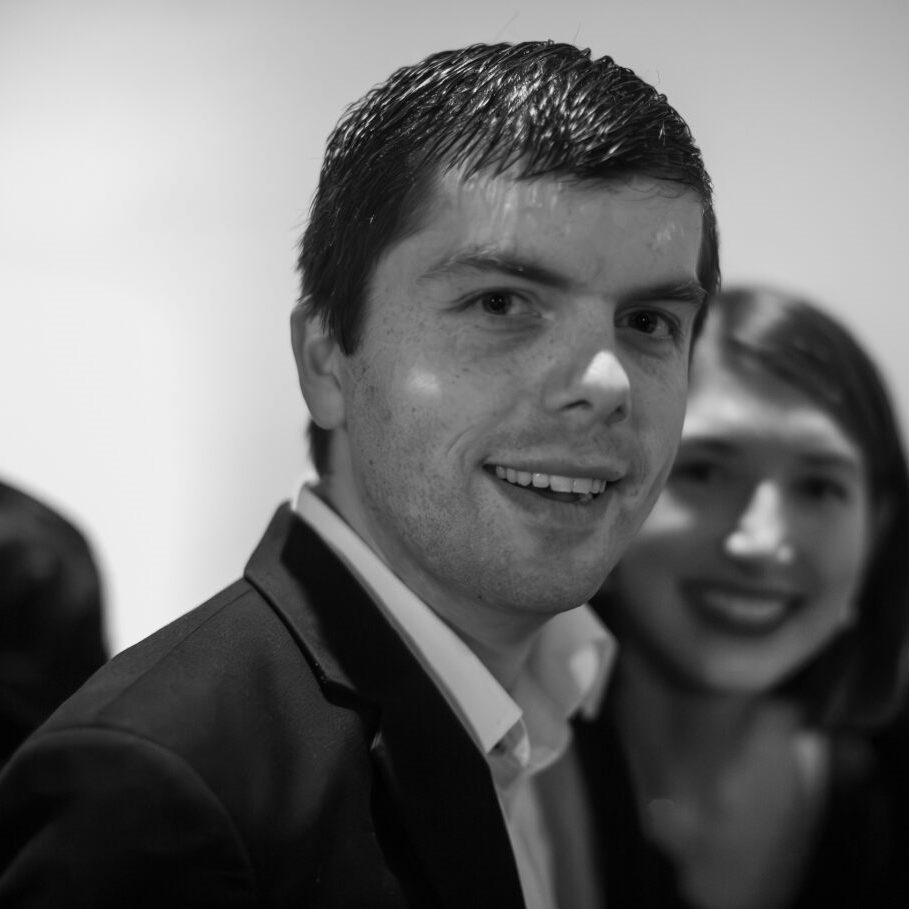
Hi,
I don't see exactly what the problem is now. Are the requests being handled and/or is the method POST actually used (did you test with GET)?
Small feedback on the code you give: I would add some additional sanity checks whether the route is valid on 'heartbeat' and 'notifications', case insenstive.
Updated code:
[AutomationEntryPoint(AutomationEntryPointType.Types.OnApiTrigger)]
public ApiTriggerOutput OnApiTrigger(IEngine engine, ApiTriggerInput requestData)
{
try
{
var method = requestData.RequestMethod;
var route = requestData.Route;
var body = requestData.RawBody;
engine.Log($"API Triggered: Route = {route}, Method = {method}, Body= {body}");
if (method != RequestMethod.Post)
{
return new ApiTriggerOutput
{
ResponseBody = "Only POST requests are supported.",
ResponseCode = (int)StatusCode.MethodNotAllowed,
};
}
if (string.IsNullOrWhiteSpace(route))
{
return new ApiTriggerOutput
{
ResponseBody = $"An empty route is passed.",
ResponseCode = (int)StatusCode.BadRequest,
};
}
if (route.Equals("heartbeat", StringComparison.OrdinalIgnoreCase))
{
return HandleHeartbeatRequest(engine, body);
}
else if (route.Equals("notifications", StringComparison.OrdinalIgnoreCase))
{
return HandleNotificationRequest(engine, body);
}
else
{
return new ApiTriggerOutput
{
ResponseBody = "Invalid route. Please check the endpoint URL.",
ResponseCode = (int)StatusCode.NotFound,
};
}
}
catch (Exception ex)
{
engine.Log($"Error: {ex.Message}");
return new ApiTriggerOutput
{
ResponseBody = "An internal server error occurred.",
ResponseCode = (int)StatusCode.InternalServerError,
};
}
}
Thankyou, Its working now 🙂